How to Check if a String Is Integer in Python
-
Check if a String Is an Integer in Python Using the
str.isdigit()
Method -
Check if a String Is an Integer in Python Using the
try ... except
Exception Handling onint()
Function - Check if a String Is an Integer in Python Using Regular Expression
-
Check if a String Is an Integer in Python Using the
literal_eval()
Function From theast
Module -
Check if a String Is an Integer in Python Using
str.replace()
andstr.isnumeric()
- Conclusion
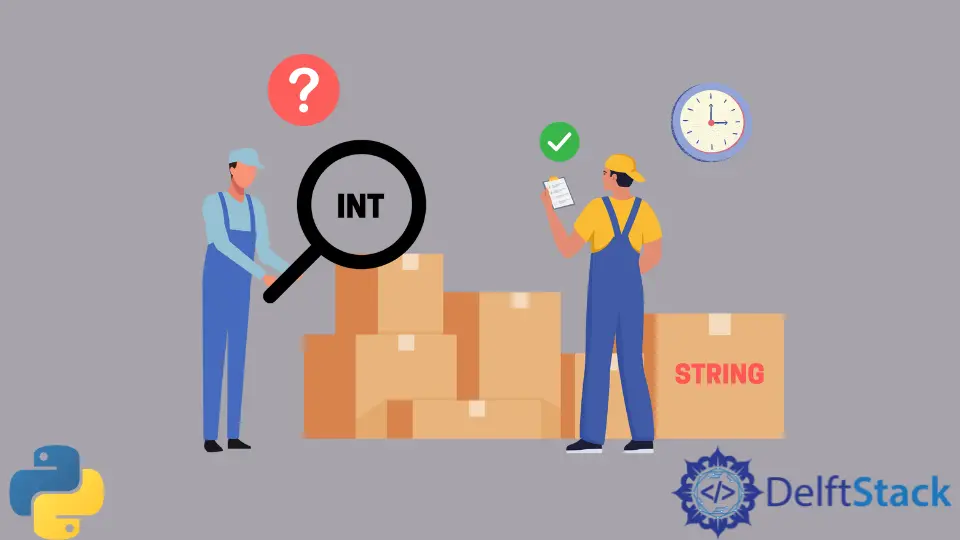
In Python, determining whether a given string represents an integer is a common task with various approaches. This comprehensive article will explore multiple methods, each with its advantages and use cases.
Check if a String Is an Integer in Python Using the str.isdigit()
Method
One of the most straightforward and efficient methods to determining whether a string represents an integer is by utilizing the str.isdigit()
method. This method checks if all the characters in the given string are digits, which makes it a suitable choice for validating integer strings.
The str.isdigit()
method has a simple syntax:
string.isdigit()
Here, string
is the string you want to check. The method returns True
if all characters in the string are digits and False
otherwise.
Let’s explore a complete working code example to illustrate how the str.isdigit()
method can be applied:
def is_integer(string):
return string.isdigit()
string1 = "132"
string2 = "-132"
string3 = "abc"
print(is_integer(string1))
print(is_integer(string2))
print(is_integer(string3))
In the code example, we define a function is_integer
that takes a string as an argument. Inside the function, the isdigit()
method is applied directly to the input string.
The function returns True
if all characters in the string are digits, indicating that the string represents an integer. Otherwise, it returns False
.
In the test cases, we apply the is_integer
function to three different strings (string1
, string2
, and string3
):
- The first string,
132
, is a valid integer, soTrue
is returned. - The second string,
-132
, contains a negative sign, soFalse
is returned. - The third string,
abc
, does not represent an integer, resulting inFalse
.
The output of the test cases is as follows:
True
False
False
This confirms that the str.isdigit()
method effectively identifies whether a given string is an integer in Python.
Check if a String Is an Integer in Python Using the try ... except
Exception Handling on int()
Function
Another effective approach to determine whether a string represents an integer involves using the try ... except
exception handling on the int()
function.
The try ... except
block allows us to execute a block of code within the try
section. If an exception occurs during this execution, the code in the except
section is triggered, allowing us to handle the exception appropriately.
try:
integer_value = int(string)
# Code to execute if conversion to integer is successful
except ValueError:
# Code to execute if conversion to integer raises a ValueError
For checking if a string is an integer, we use the int()
function inside the try
block. If the conversion is successful, it means the string is a valid integer, and the function returns True
.
If a ValueError
occurs, indicating that the string couldn’t be converted to an integer, the except
block is executed, and the function returns False
.
Let’s delve into a code example to demonstrate the application of try ... except
for checking if a string is an integer:
def is_integer(string):
try:
int(string)
return True
except ValueError:
return False
string1 = "123"
string2 = "-456"
string3 = "abc"
print(is_integer(string1))
print(is_integer(string2))
print(is_integer(string3))
Here, the is_integer
function takes a string as an argument. Inside the function, the int()
function is used within the try
block to attempt to convert the string to an integer.
If successful, the function returns True
, indicating that the string represents a valid integer. If a ValueError
occurs during the conversion, the except
block is triggered, and the function returns False
.
In the test cases, we apply the is_integer
function to three different strings (string1
, string2
, and string3
).
The first two strings, 123
and -456
, are valid integers, so True
is returned. The third string, abc
, cannot be converted to an integer, resulting in False
.
Output:
True
True
False
Check if a String Is an Integer in Python Using Regular Expression
Another versatile approach to determine if a string represents an integer involves using regular expressions. Regular expressions provide a powerful way to define and match patterns in strings, making them well-suited for tasks like this.
The regular expression pattern [+-]?\d+$
is particularly useful for validating integer strings. The regular expression [+-]?\d+$
breaks down as follows:
[+-]?
: Indicates that the presence of a positive or negative sign is optional.\d+
: Specifies that there should be one or more digits in the string.$
: Marks the end of the string.
When applied to a string, this pattern ensures that the string contains optional positive or negative signs followed by one or more digits until the end of the string. If the pattern matches, the string is considered to represent an integer.
Let’s explore a complete working code example illustrating the use of regular expressions for checking if a string is an integer:
import re
def is_integer(string):
regex_pattern = "[-+]?\d+$"
return re.match(regex_pattern, string) is not None
string1 = "123"
string2 = "-456"
string3 = "abc123"
print(is_integer(string1))
print(is_integer(string2))
print(is_integer(string3))
In the code example, the is_integer
function takes a string as an argument. Inside the function, the re.match()
function is used to apply the regular expression pattern to the input string.
If a match is found, the function returns True
, indicating that the string represents an integer. If no match is found, it returns False
.
In the test cases, we apply the is_integer
function to three different strings (string1
, string2
, and string3
). The first two strings represent integers, and the third string contains non-numeric characters, making it fail the integer validation.
Output:
True
True
False
Check if a String Is an Integer in Python Using the literal_eval()
Function From the ast
Module
In Python, the ast
module provides a powerful tool for parsing Python expressions safely. One approach to check if a string represents an integer involves using the literal_eval()
function from the ast
module.
This function evaluates a string containing a Python literal or container display, such as a string, list, or tuple, and returns the corresponding Python object.
The syntax for using ast.literal_eval()
is as follows:
import ast
result = ast.literal_eval(string)
Here, string
is the input string that we want to evaluate. If the string represents a valid Python literal, the function returns the corresponding Python object; otherwise, it raises a ValueError
.
Let’s explore a complete working code example using the ast
module to check if a string is an integer:
import ast
def is_integer(string):
try:
ast.literal_eval(string)
return True
except (ValueError, SyntaxError):
return False
string1 = "132"
string2 = "-132"
string3 = "abc"
print(is_integer(string1))
print(is_integer(string2))
print(is_integer(string3))
Here, the is_integer
function utilizes the ast.literal_eval()
function within a try ... except
block. If the evaluation is successful, indicating that the string represents a valid Python literal, the function returns True
.
If a ValueError
or SyntaxError
occurs during the evaluation (e.g., due to non-literal content in the string), the function returns False
.
In the test cases, the function is applied to three different strings (string1
, string2
, and string3
). The first two strings represent integers and are successfully evaluated, while the third string, containing non-literal content, results in a False
return value.
Output:
True
True
False
This illustrates how the ast
module’s literal_eval()
function provides a safe and effective way to check if a string represents an integer, handling potential syntax errors and non-literal content gracefully.
Check if a String Is an Integer in Python Using str.replace()
and str.isnumeric()
In certain cases, checking if a string represents an integer may involve handling negative integers or strings with leading/trailing spaces. One approach is to use the combination of str.replace()
and str.isnumeric()
to accommodate such variations.
The idea behind this method is to first remove a leading negative sign if present using str.replace("-", "", 1)
. Then, we use the str.isnumeric()
method to check if the remaining string consists of numeric characters.
This approach allows us to handle negative integers while ensuring the string only contains digits.
Let’s explore a complete working code example using str.replace()
and str.isnumeric()
to check if a string is an integer:
def is_integer(string):
cleaned_string = string.replace("-", "", 1)
return cleaned_string.isnumeric()
string1 = "132"
string2 = "-132"
string3 = "abc"
print(is_integer(string1))
print(is_integer(string2))
print(is_integer(string3))
In the code example, the is_integer
function takes a string as an argument. It uses str.replace("-", "", 1)
to remove a leading negative sign if present in the string.
The resulting cleaned string is then checked for numeric characters using str.isnumeric()
.
In the test cases, the function is applied to three different strings (string1
, string2
, and string3
). The first two strings represent integers, and the third string is not recognized as an integer.
Output:
True
True
False
Conclusion
Choosing the appropriate method depends on the specific requirements of your use case. Whether you need a quick check, want to handle variations in string content, or prefer a more flexible pattern-matching approach, these methods provide versatile options for checking if a string represents an integer in Python.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python