How to Change File Permissions in Python
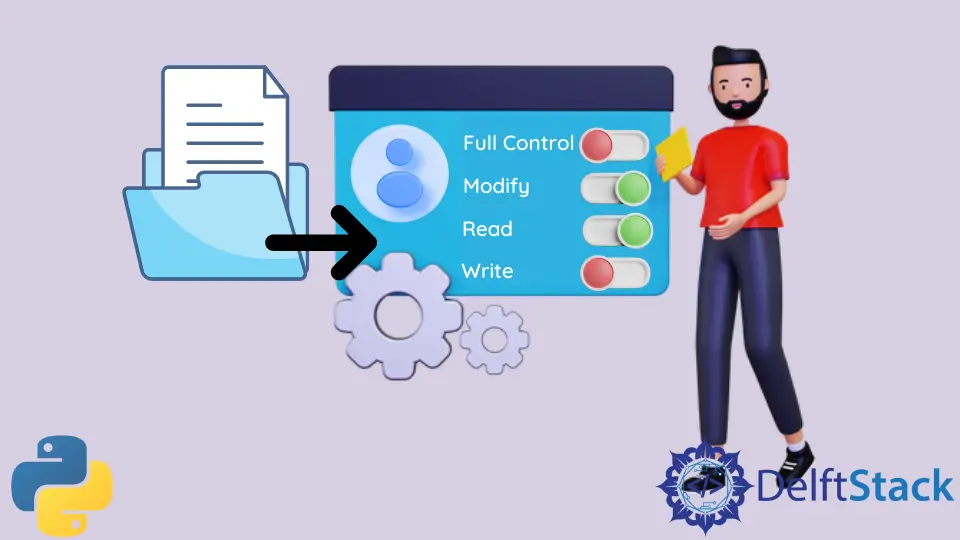
When working with files within Python or around a Python application, it can be imperative to have the proper file permissions to help achieve the operations intended. From working script files to data exports, the files created, updated, or managed by the Python applications can also be worked upon with the Python code.
Typically, the usual way for most developers to work with file permissions is to use command line commands or scripts. However, it is possible to change file permissions within Python using specific built-in libraries and their methods.
This article will discuss how to change file permissions in Python.
OS File Permissions in Python
Within the operating systems, from Windows to Linux, different permissions are native to them. The permissions to read, write, and execute are set to files depending on their purpose.
The File Explorer often provides UIs to allow typical users to change the file permissions granted to a file. However, for most developers, the command line interface is where it happens.
For Linux (generally Unix systems) and even Windows, you can check the file permissions, among other information that a file holds.
On Windows:
ls
Output:
Directory: C:\Users\USER
Mode LastWriteTime Length Name
---- ------------- ------ ----
d----- 7/13/2022 8:09 PM .android
d----- 9/23/2022 8:11 PM .vscode
d-r--- 5/9/2022 1:34 PM 3D Objects
d----- 5/12/2022 11:49 PM Apple
d----- 8/30/2022 1:20 PM Autodesk
d-r--- 3/18/2022 6:34 AM Contacts
d-r--- 9/23/2022 8:19 PM Desktop
d-r--- 9/23/2022 8:52 PM Documents
d-r--- 9/28/2022 12:48 PM Downloads
d-r--- 3/18/2022 6:34 AM Favorites
d-r--- 4/28/2022 11:03 AM Links
d-r--- 7/16/2022 10:41 PM Music
d-r--- 4/11/2022 11:39 PM OneDrive
d-r--- 9/22/2022 11:13 AM Pictures
d-r--- 3/18/2022 6:34 AM Saved Games
d-r--- 3/18/2022 6:35 AM Searches
d-r--- 9/26/2022 2:49 PM Videos
-a---- 9/23/2022 8:25 PM 5 .node_repl_history
-a---- 9/27/2022 2:34 AM 1100 pslog.txt
The mode
tab holds the file permission information which is the first column showing the type of the file.
Similarly, for Unix systems such as Linux, you can list all the file information.
ls -la
Output:
drwxr-xr-x 6 eva users 1024 Jun 8 16:46 sabon
-rw------- 1 eva users 1564 Apr 28 14:35 splus
-rw------- 1 eva users 1119 Apr 28 16:00 splus2
-rw-r--r-- 1 eva users 9753 Sep 27 11:14 ssh_known_hosts
-rw-r--r-- 1 eva users 4131 Sep 21 15:23 swlist.out
-rw-r--r-- 1 eva users 94031 Sep 1 16:07 tarnti.zip
Also, the first column holds the mode (drwxr-xr-x
). In the first column, there are four breakdowns.
The first breakdown is the first character which represents the actual file type. The second breakdown (the next three characters) shows the user’s access rights (or permissions).
The third breakdown (next three characters) shows the permissions of the group which owns the file. And the last breakdown (last three characters) shows the permissions of other users.
Now, let’s use this drwxr-xr-x
mode to explain it. Before we go on, you need to know what the three letters represent:
r
means read.w
means write.x
means execute.
Each file or directory is assigned access rights for the file owner, the members of a group of related users, and others. Rights can be set to:
- Read a file.
- Write a file.
- Execute a file.
The first character, d
, shows that it’s a directory. However, if the character is -
, it is a typical file.
The next three sets of characters, rwx
, means the users can read, write and execute the directory. The next three, r-x
, means the group can read and execute but not write the directory.
The last three, r-x
, means others can read and execute but not write the directory.
So, to change a file’s permission in Linux, a simple chmod
command is often useful.
chmod u+x someFile
The u+x
is the permission given to someFile
.
The u+x
permission adds (+
) execution rights to the user (remember, user, group, and other users) of someFile
. Remember, to execute is represented by x
.
But we can achieve this within Python quite easily. With this knowledge, you can now change the permissions of files within Python without any issues.
Use chmod()
to Change File Permissions in Python
The chmod()
method stems from the chmod
command within Linux, whose job is to change the mode of a file or directory. The chmod()
method is available via the os
module, which is a default with any Python installation or environment.
The os
module provides interfaces and methods to allow us to interact with any operating system. It provides OS routines for NT or Posix, depending on its system.
Among many functions, it allows us to manage directories, retrieve their content and change the behaviors and modes within such directories.
Aside from the chmod()
method, we need another module called stat
, which provides constants/functions for interpreting results of os.stat()
and os.lstat()
. This stat
provides the permission flags that we will need to change the file permissions across the user, group, or other users.
The permissions flags fall across include read, write, and execute operations.
We must import their modules to use chmod()
and stat
.
import os
import stat
Also, we need to access the file we want to change its permissions. The os
module is useful for that too.
The chmod()
works with the following syntax:
os.chmod(filePath, permissionFlag)
To check for the current permission mode of a file (say, a newly created index.txt
), you can use the stat()
method.
perm = os.stat("index.txt")
print(perm.st_mode)
Output:
33206
The os.stat()
gets the status of a specified path, and the st_mode
property provides the permission bits of the file where the first two octal digits specify the file type and the other four are the file permissions.
The file must be within the same directory as the Python file or place the complete file path.
Using the octal notation (one of two ways to specify permissions) for Linux users, we can think of permissions settings as a series of bits, which can be seen described in the code snippet below.
rwx rwx rwx = 111 111 111
rw- rw- rw- = 110 110 110
rwx --- --- = 111 000 000
# rwx = 111 in binary = 7
# rw- = 110 in binary = 6
# r-x = 101 in binary = 5
# r-- = 100 in binary = 4
To change the permission mode, you can then use the stat
permission flags:
import os
import stat
perm = os.stat("index.txt")
print(perm.st_mode)
os.chmod("index.txt", stat.S_IWGRP)
perm = os.stat("index.txt")
print(perm.st_mode)
Output:
33206
33060
The first octal number shows the permission status before the change, and the second octal number is the one after adding the reading by group permissions.
You can combine different permissions flags using the |
operator. In this example, we will add the permissions for the user to read, write and execute.
import os
import stat
perm = os.stat("index.txt")
print(perm.st_mode)
os.chmod("index.txt", stat.S_IREAD | stat.S_IWRITE | stat.S_IEXEC | stat.S_IRUSR)
perm = os.stat("index.txt")
print(perm.st_mode)
Output:
33060
33206
There are other permission flags which can be seen below:
stat.S_IREAD
,stat.S_IWRITE
, andstat.S_IEXEC
flags for adding read, write and execute rights for a user to a file, respectively.stat.S_IRGRP
,stat.S_IWGRP
, andstat.S_IXGRP
flags for adding read, write and execute rights for a group to a file, respectively.stat.S_IROTH
,stat.S_IWOTH
, andstat.S_IXOTH
flags for adding read, write and execute rights for other users to a file, respectively.stat.S_IRWXU
,stat.S_IRWXG
, andstat.S_IRWXO
flags for adding read, write and execute rights simultaneously for the user, group, and other users, respectively.
Aside from using the permission flags, you can use the octal notations within the chmod()
method. Let’s use the octal number 755
, which specifies that the user can read, write and execute, and the group and other users can only read and execute.
os.chmod("index.txt", 0o755)
Output:
33206
You must add the 0o
prefix to indicate that it is an octal notation.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn