How to Fix Python Cannot Concatenate STR and Int Objects Error
-
the
TypeError: cannot concatenate 'str' and 'int' objects
in Python -
Fix the
TypeError: cannot concatenate 'str' and 'int' objects
Error in Python - Conclusion
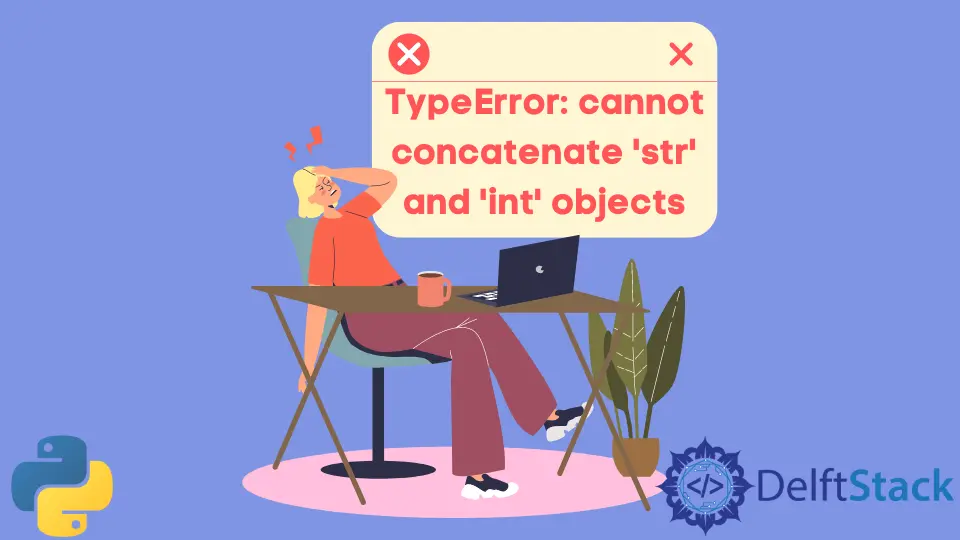
While coding in any programming language, we encounter certain unexpected situations that cause abnormal behavior in the program. These unexpected situations are known as errors.
In this article, we’ll discuss the error known as the TypeError: cannot concatenate 'str' and 'int' objects
error in Python.
the TypeError: cannot concatenate 'str' and 'int' objects
in Python
Errors in programming can arise unexpectedly due to factors such as incorrect user input, unforeseen conditions, or coding mistakes. These errors can be categorized into logical, runtime, and syntax errors, each presenting unique challenges.
The TypeError: cannot concatenate 'str' and 'int' objects
in Python is a specific type of runtime error that occurs during program execution. Attempting to divide a number by zero is an example of a runtime error, and this particular error deals with issues related to the types of variables used in a program, often arising when attempting to concatenate values of different types.
Consider the examples: a = 2 + 3
and s = "Rohan" + "Roshni"
. Here, variables a
and s
involve operations that successfully add or concatenate values of the same types.
In the code below, attempting to add a string object with an integer object is demonstrated.
Example Code:
x = "Rohan" + 2
print(x)
Output:
Traceback (most recent call last):
File "<pyshell#1>", line 1, in <module>
x = "Rohan" + 2
TypeError: can only concatenate str (not "int") to str
The output provides a TypeError
stating: "can only concatenate str (not 'int') to str"
. This indicates that a string object must only be concatenated with another string object, while an integer object must only be added to another integer object.
In scenarios where concatenating a string and an integer object is necessary, such as when printing a message along with a value, various solutions can be employed to avoid the TypeError
. The forthcoming section will delve into these solutions to provide a clear path for addressing and resolving this common runtime error.
Fix the TypeError: cannot concatenate 'str' and 'int' objects
Error in Python
To deal with such situations where we have to concatenate a string and an integer object, we have several ways to avoid this error. We’ll now discuss the ways how to resolve the TypeError: cannot concatenate 'str' and 'int' objects
error in Python.
Use the In-Built str()
Function in Python
Python has an in-built function called str()
that converts an integer value to a string. However, this str()
function takes an integer value as an argument and returns a string value to the user.
We can convert the integer value so that it is cast and can be concatenated with a string value. Let us take an example to show the error that we counter and then the solution using the str()
function.
Example Code:
a = 10
print("The value =:" + a)
In this code, we assign the value of 10 to the variable a
and attempt to print a concatenated string using the print
statement. The error occurs because we directly concatenate a string with an integer.
Output:
Traceback (most recent call last):
File "<pyshell#6>", line 1, in <module>
print("The value =:" + a)
TypeError: can only concatenate str (not "int") to str
As evident from the output example above, attempting to concatenate a string with an integer leads to a TypeError: cannot concatenate 'str' and 'int' objects
.
To address this issue, we convert the integer to a string, ensuring both operands in the concatenation are of the same type. This can be achieved using the function str()
.
Solution Code:
a = 10
print("The value =:" + str(a))
In the code above, we use the str()
function to convert the integer variable a
to a string. By incorporating str(a)
in the concatenation, we ensure that both operands share the same data type.
Output:
The value =:10
In the output, the variable a
is converted to a string value using Python’s function str()
to avoid the TypeError: cannot concatenate 'str' and 'int' objects
error.
Use Comma While Using the print
Statement in Python
The print
statement in Python can also be passed with values of different data types. All the values passed to the print
statement are internally converted to a string data type and printed.
Solution Code:
a = 10
print("The value of a=", a)
In this code, we utilize a comma (,
) within the print
statement, separating the string and the variable a
. Python automatically handles the conversion of the integer to a string, eliminating the need for explicit type conversion.
Output:
The value of a= 10
The output displays the result The value of a= 10
. Therefore, replacing the +
concatenation symbol with a comma (,
) resolved the TypeError: cannot concatenate 'str' and 'int' objects
error in Python.
This approach ensures compatibility between the string and the variable, addressing the issue of mixing str
and int
objects.
Use the format()
Function in Python
We can also resolve the error by using Python’s format()
function.
The format()
function is an in-built method used for variable substitution and data formatting. This function lets you concatenate the string parts at particular positions through placeholders.
These placeholders are represented by a pair of curly braces {}
. We must use the placeholder where we want to concatenate the other value and call the str.format()
method after it.
The format()
method takes the value that needs to be concatenated. It can be of any data type, integer, float, or string.
Example Code:
a = 10
print("The value of a = {}".format(a))
In this code, we utilize the format()
function in Python. Inside the string, we include curly braces {}
as placeholders where we want to concatenate the variable a
.
We then call the format()
method on the string, passing the variable a
as an argument. This method seamlessly substitutes the placeholder with the string representation of a
, ensuring compatibility in data types.
Output:
The value of a = 10
The output displays the message The value of a = 10
, successfully addressing the concatenation issue without explicit type conversion.
Use the f-strings
in Python
Introduced in Python 3.6, f-strings
provides a straightforward method to concatenate strings and variables without explicitly converting them to strings. We can directly include variables inside curly braces ({}
) within the string.
Example Code:
a = 10
print(f"The value of a = {a}")
In this code, we used f-strings
. The 'f'
prefix before the string allows us to embed expressions within curly braces {}
directly in the string.
By placing the variable a
within these curly braces, Python automatically converts it to its string representation.
Output:
The value of a = 10
In this output, the f-string
successfully concatenates the string "The value of a ="
with the value of the variable a
, which is 10. The use of f-strings
automatically converts the integer to its string representation, resulting in a formatted output without encountering the TypeError
.
Conclusion
In conclusion, this article has explored the TypeError: cannot concatenate 'str' and 'int' objects
error in Python, a common runtime issue encountered during program execution. By illustrating the error through examples and providing a detailed analysis of its causes, the article has offered multiple solutions to address and resolve this specific error.
The solutions discussed include using the in-built str()
function to convert integers to strings, employing commas within the print
statement for automatic type conversion, utilizing the format()
function for variable substitution and data formatting, and adopting f-strings
introduced in Python 3.6. Each solution is accompanied by practical code examples and output demonstrations, ensuring clarity and comprehension for readers facing similar challenges in their Python programming endeavors.
By understanding and applying these solutions, developers can effectively tackle the TypeError
associated with concatenating string and integer objects, enhancing their ability to write robust and error-free Python code.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python