How to Solve AttributeError: 'list' Object Attribute 'append' Is Read-Only
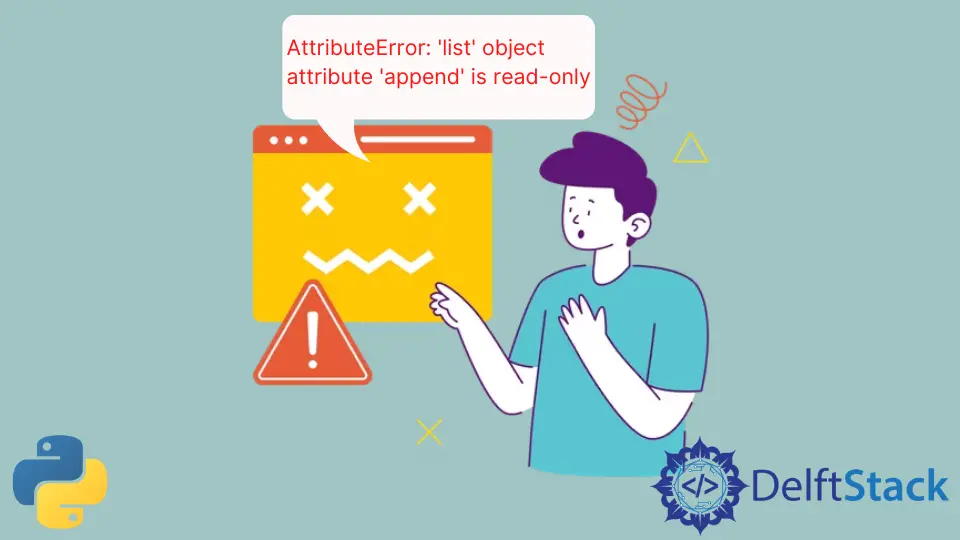
We can run different operations (methods) on the data type when working with lists in Python. We must understand how they work to use them effectively and without errors.
To uses these methods, we need to know their syntax, errors, and mode of operation. One of these many methods is the append()
method, which helps us add a new element to a list.
However, if we misuse it, we will get an AttributeError: 'list' object attribute 'append' is read-only
error message.
This article will show you what causes this AttributeError: 'list' object attribute 'append' is read-only
error message and how to solve it.
Use the Correct Syntax to Solve AttributeError: 'list' object attribute 'append' is read-only
The AttributeError: 'list' object attribute 'append' is read-only
error message is an AttributeError
which means that an attribute reference or assignment fails.
We can understand what might be happening from the error message. The object attribute append
is read-only, and a referencing or assignment operation is failing because of this condition.
When data is read-only, which append
is, it can only be accessed but not modified. Therefore, in our code, there is an expression trying to modify the 'list' object attribute 'append'
.
Let us try to replicate the same error message using a simple Python code.
In this code, we create a variable, shopList
, which holds a list of elements. Then, another variable, value
, binds to a string, toothpick
.
Afterward, it prints the content of shopList
. And finally, it tried to append the binding value
to the list shopList
.
Code:
shopList = ["banana", "orange", "sugar", "salt"]
value = "toothpick"
print(shopList)
shopList.append = value
Output:
['banana', 'orange', 'sugar', 'salt']
Traceback (most recent call last):
File "c:\Users\akinl\Documents\Python\alt.py", line 4, in <module>
shopList.append = value
AttributeError: 'list' object attribute 'append' is read-only
We can see the error message AttributeError: 'list' object attribute 'append' is read-only
we intend to solve. From the error, we know the cause of the error is present in line 4.
The code below is what’s present in line 4:
shopList.append = value
Now, what’s wrong here?
The attribute is called append
. The code tried to assign the binding value
to the append
method, which resulted in errors and exceptions because you shouldn’t replace methods on built-in objects.
What caused the AttributeError
is a SyntaxError
concerning how to use the append
method. The correct way to use the append
method can be seen below:
shopList.append(value)
Now, let’s rewrite the same code.
shopList = ["banana", "orange", "sugar", "salt"]
value = "toothpick"
print(shopList)
shopList.append(value)
print(shopList)
Output:
['banana', 'orange', 'sugar', 'salt']
['banana', 'orange', 'sugar', 'salt', 'toothpick']
Therefore, check your syntax when faced with an AttributeError
because the same error can be seen with other methods such as index
.
Code:
shopList = ["banana", "orange", "sugar", "salt"]
shopList.index = "banana"
Output:
Traceback (most recent call last):
File "c:\Users\akinl\Documents\Python\index.py", line 2, in <module>
shopList.index = "banana"
AttributeError: 'list' object attribute 'index' is read-only
This time the error is AttributeError: 'list' object attribute 'index' is read-only
instead of an append
one.
Always be careful with your syntax.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python