How to Append New Row to a CSV File in Python
-
Append Data in List to CSV File in Python Using
writer.writerow()
-
Append Data in Dictionary to CSV File in Python Using
DictWriter.writerow()
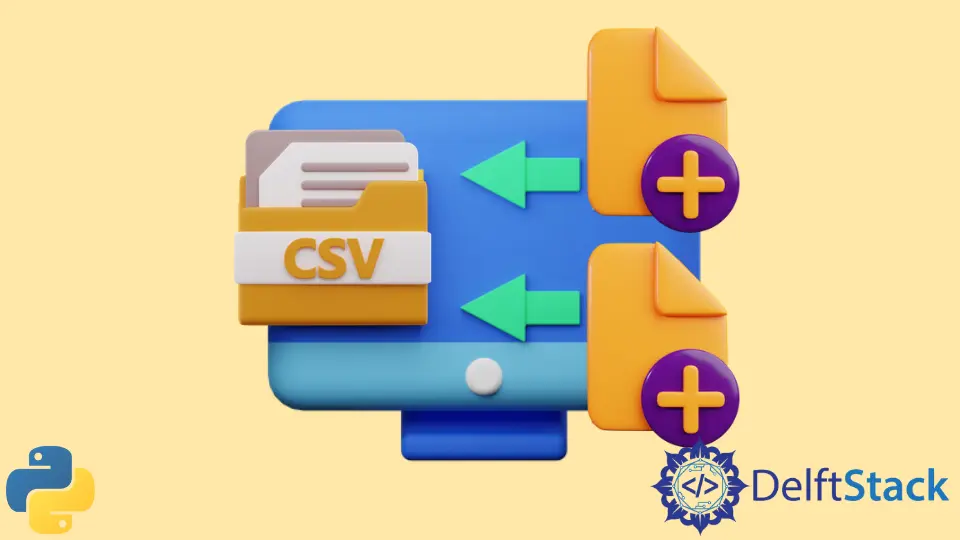
If you wish to append a new row into a CSV file in Python, you can use any of the following methods.
- Assign the desired row’s data into a List. Then, append this List’s data to the CSV file using
writer.writerow()
. - Assign the desired row’s data into a Dictionary. Then, append this dictionary’s data to the CSV file using
DictWriter.writerow()
.
Append Data in List to CSV File in Python Using writer.writerow()
In this case, before we append the new row into the old CSV file, we need to assign the row values to a list.
For example,
list = ["4", "Alex Smith", "Science"]
Next, pass this data from the List as an argument to the CSV writer()
object’s writerow()
function.
For example,
csvwriter_object.writerow(list)
Pre-requisites:
-
The CSV
writer
class has to be imported from theCSV
module.from csv import writer
-
Before running the code, the CSV file has to be manually closed.
Example - Append Data in List to CSV File Using writer.writerow()
Here is an example of the code that shows how one can append the data present in a List into a CSV file -
# Pre-requisite - Import the writer class from the csv module
from csv import writer
# The data assigned to the list
list_data = ["03", "Smith", "Science"]
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the writer() function
writer_object = writer(f_object)
# Result - a writer object
# Pass the data in the list as an argument into the writerow() function
writer_object.writerow(list_data)
# Close the file object
f_object.close()
Suppose before running the code; the old CSV file contains the below content.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
Once the code runs, the CSV file will be modified.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
Append Data in Dictionary to CSV File in Python Using DictWriter.writerow()
In this case, before we append the new row into the old CSV file, assign the row values to a dictionary.
For example,
dict = {"ID": 5, "NAME": "William", "SUBJECT": "Python"}
Next, pass this data from the dictionary as an argument to the dictionary DictWriter()
object’s writerow()
function.
For example,
dictwriter_object.writerow(dict)
Pre-requisites:
-
The
DictWriter
class has to be imported from theCSV
module.from csv import DictWriter
-
Before running the code, the CSV file has to be manually closed.
Example - Append Data in Dictionary to CSV File Using DictWriter.writerow()
Here is an example of the code that shows how one can append the data present in a Dictionary into a CSV file.
# Pre-requisite - Import the DictWriter class from csv module
from csv import DictWriter
# The list of column names as mentioned in the CSV file
headersCSV = ["ID", "NAME", "SUBJECT"]
# The data assigned to the dictionary
dict = {"ID": "04", "NAME": "John", "SUBJECT": "Mathematics"}
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the Dictwriter() function
# Result - a DictWriter object
dictwriter_object = DictWriter(f_object, fieldnames=headersCSV)
# Pass the data in the dictionary as an argument into the writerow() function
dictwriter_object.writerow(dict)
# Close the file object
f_object.close()
Suppose, before running the code, the old CSV file contains the below content.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
Once the code runs, the CSV file will be modified.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
04,John,Mathematics
Related Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python