Python에서 CSV 파일에 새 행 추가
Najwa Riyaz
2023년1월30일
Python
Python CSV
-
writer.writerow()
를 사용하여 목록의 데이터를 Python의 CSV 파일에 추가 -
DictWriter.writerow()
를 사용하여 사전의 데이터를 Python의 CSV 파일에 추가
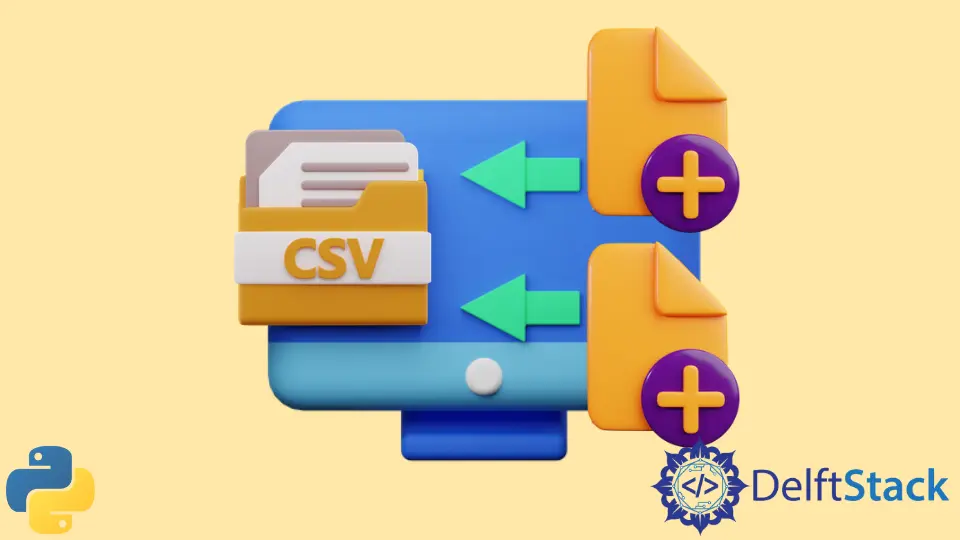
Python의 CSV 파일에 새 행을 추가하려는 경우 다음 방법 중 하나를 사용할 수 있습니다.
- 원하는 행의 데이터를 목록에 할당합니다. 그런 다음
writer.writerow()
를 사용하여이 목록의 데이터를 CSV 파일에 추가합니다. - 원하는 행의 데이터를 사전에 할당합니다. 그런 다음
DictWriter.writerow()
를 사용하여이 사전의 데이터를 CSV 파일에 추가합니다.
writer.writerow()
를 사용하여 목록의 데이터를 Python의 CSV 파일에 추가
이 경우 새 행을 이전 CSV 파일에 추가하기 전에 행 값을 목록에 할당해야합니다.
예를 들면
list = ["4", "Alex Smith", "Science"]
다음으로 목록의이 데이터를 CSV writer()
개체의writerow()
함수에 인수로 전달합니다.
예를 들면
csvwriter_object.writerow(list)
전제 조건 :
-
CSV
writer
클래스는CSV
모듈에서 가져와야합니다.from csv import writer
-
코드를 실행하기 전에 CSV 파일을 수동으로 닫아야합니다.
예-writer.writerow()
를 사용하여 목록의 데이터를 CSV 파일에 추가
다음은 목록에있는 데이터를 CSV 파일에 추가하는 방법을 보여주는 코드의 예입니다.
# Pre-requisite - Import the writer class from the csv module
from csv import writer
# The data assigned to the list
list_data = ["03", "Smith", "Science"]
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the writer() function
writer_object = writer(f_object)
# Result - a writer object
# Pass the data in the list as an argument into the writerow() function
writer_object.writerow(list_data)
# Close the file object
f_object.close()
코드를 실행하기 전에 가정하십시오. 이전 CSV 파일에는 아래 내용이 포함되어 있습니다.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
코드가 실행되면 CSV 파일이 수정됩니다.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
DictWriter.writerow()
를 사용하여 사전의 데이터를 Python의 CSV 파일에 추가
이 경우 새 행을 이전 CSV 파일에 추가하기 전에 행 값을 사전에 할당합니다.
예를 들면
dict = {"ID": 5, "NAME": "William", "SUBJECT": "Python"}
다음으로, 사전의이 데이터를 사전DictWriter()
객체의writerow()
함수에 인수로 전달합니다.
예를 들면
dictwriter_object.writerow(dict)
전제 조건 :
-
DictWriter
클래스는CSV
모듈에서 가져와야합니다.from csv import DictWriter
-
코드를 실행하기 전에 CSV 파일을 수동으로 닫아야합니다.
예-DictWriter.writerow()
를 사용하여 사전의 데이터를 CSV 파일에 추가
다음은 사전에있는 데이터를 CSV 파일에 추가하는 방법을 보여주는 코드의 예입니다.
# Pre-requisite - Import the DictWriter class from csv module
from csv import DictWriter
# The list of column names as mentioned in the CSV file
headersCSV = ["ID", "NAME", "SUBJECT"]
# The data assigned to the dictionary
dict = {"ID": "04", "NAME": "John", "SUBJECT": "Mathematics"}
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the Dictwriter() function
# Result - a DictWriter object
dictwriter_object = DictWriter(f_object, fieldnames=headersCSV)
# Pass the data in the dictionary as an argument into the writerow() function
dictwriter_object.writerow(dict)
# Close the file object
f_object.close()
코드를 실행하기 전에 이전 CSV 파일에 아래 내용이 포함되어 있다고 가정합니다.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
코드가 실행되면 CSV 파일이 수정됩니다.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
04,John,Mathematics
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다