在 Python 中将新行附加到 CSV 文件
Najwa Riyaz
2023年1月30日
-
使用
writer.writerow()
将列表中的数据附加到 Python 中的 CSV 文件 -
使用
DictWriter.writerow()
将字典中的数据附加到 Python 中的 CSV 文件
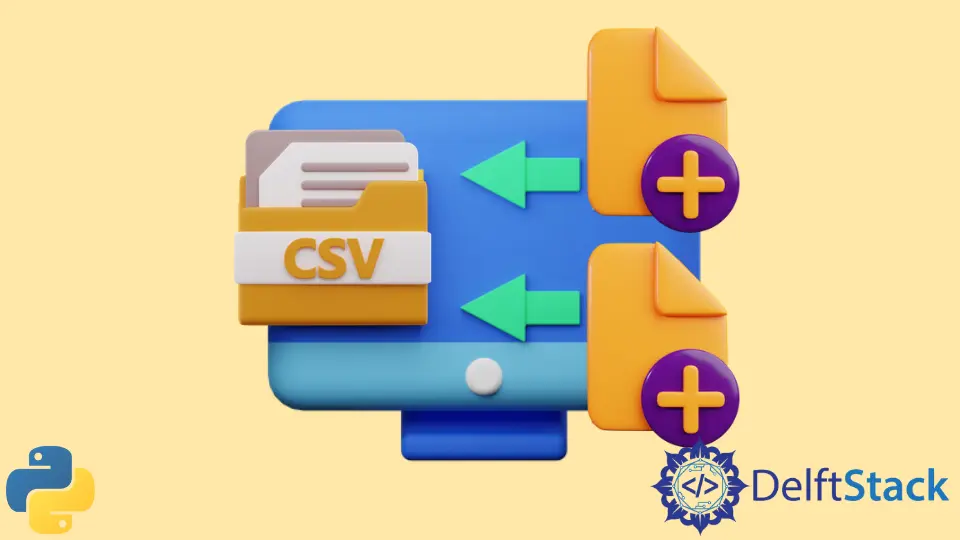
如果你希望在 Python 中将新行附加到 CSV 文件中,你可以使用以下任何一种方法。
- 将所需行的数据分配到列表中。然后,使用
writer.writerow()
将此列表的数据附加到 CSV 文件。 - 将所需行的数据分配到字典中。然后,使用
DictWriter.writerow()
将此字典的数据附加到 CSV 文件中。
使用 writer.writerow()
将列表中的数据附加到 Python 中的 CSV 文件
在这种情况下,在我们将新行附加到旧 CSV 文件之前,我们需要将行值分配给一个列表。
例如,
list = ["4", "Alex Smith", "Science"]
接下来,将 List 中的数据作为参数传递给 CSV writer()
对象的 writerow()
函数。
例如,
csvwriter_object.writerow(list)
先决条件:
-
CSV
writer
类必须从CSV
模块导入。from csv import writer
-
在运行代码之前,必须手动关闭 CSV 文件。
示例 - 使用 writer.writerow()
将列表中的数据附加到 CSV 文件
这是一个代码示例,显示了如何将列表中存在的数据附加到 CSV 文件中 -
# Pre-requisite - Import the writer class from the csv module
from csv import writer
# The data assigned to the list
list_data = ["03", "Smith", "Science"]
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the writer() function
writer_object = writer(f_object)
# Result - a writer object
# Pass the data in the list as an argument into the writerow() function
writer_object.writerow(list_data)
# Close the file object
f_object.close()
假设在运行代码之前;旧的 CSV 文件包含以下内容。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
代码运行后,CSV 文件将被修改。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
使用 DictWriter.writerow()
将字典中的数据附加到 Python 中的 CSV 文件
在这种情况下,在我们将新行附加到旧 CSV 文件之前,将行值分配给字典。
例如,
dict = {"ID": 5, "NAME": "William", "SUBJECT": "Python"}
接下来,将字典中的这些数据作为参数传递给字典 DictWriter()
对象的 writerow()
函数。
例如,
dictwriter_object.writerow(dict)
先决条件:
-
DictWriter
类必须从CSV
模块导入。from csv import DictWriter
-
在运行代码之前,必须手动关闭 CSV 文件。
示例 - 使用 DictWriter.writerow()
将字典中的数据附加到 CSV 文件
下面是一个代码示例,它展示了如何将字典中存在的数据附加到 CSV 文件中。
# Pre-requisite - Import the DictWriter class from csv module
from csv import DictWriter
# The list of column names as mentioned in the CSV file
headersCSV = ["ID", "NAME", "SUBJECT"]
# The data assigned to the dictionary
dict = {"ID": "04", "NAME": "John", "SUBJECT": "Mathematics"}
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the Dictwriter() function
# Result - a DictWriter object
dictwriter_object = DictWriter(f_object, fieldnames=headersCSV)
# Pass the data in the dictionary as an argument into the writerow() function
dictwriter_object.writerow(dict)
# Close the file object
f_object.close()
假设在运行代码之前,旧的 CSV 文件包含以下内容。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
代码运行后,CSV 文件将被修改。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
04,John,Mathematics