在 Python 中將新行附加到 CSV 檔案
Najwa Riyaz
2023年1月30日
Python
Python CSV
-
使用
writer.writerow()
將列表中的資料附加到 Python 中的 CSV 檔案 -
使用
DictWriter.writerow()
將字典中的資料附加到 Python 中的 CSV 檔案
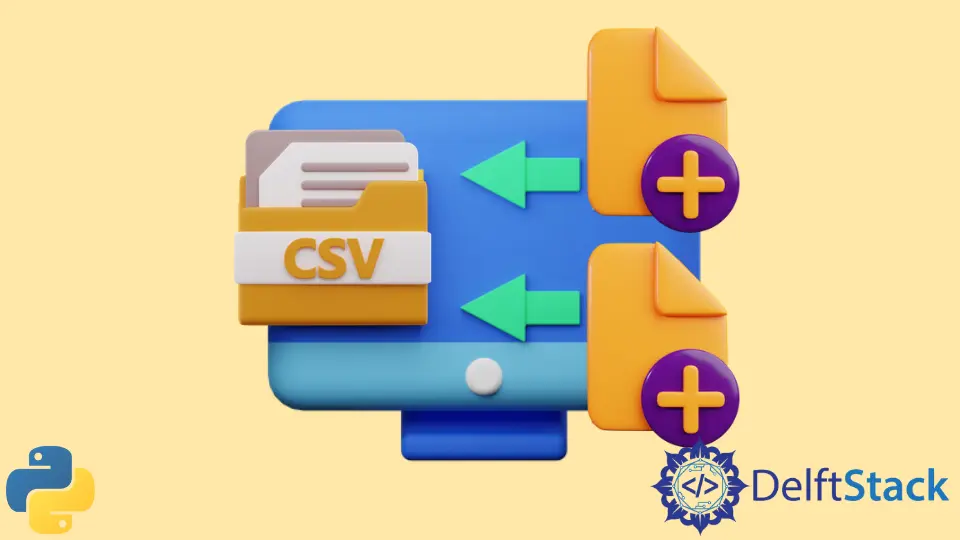
如果你希望在 Python 中將新行附加到 CSV 檔案中,你可以使用以下任何一種方法。
- 將所需行的資料分配到列表中。然後,使用
writer.writerow()
將此列表的資料附加到 CSV 檔案。 - 將所需行的資料分配到字典中。然後,使用
DictWriter.writerow()
將此字典的資料附加到 CSV 檔案中。
使用 writer.writerow()
將列表中的資料附加到 Python 中的 CSV 檔案
在這種情況下,在我們將新行附加到舊 CSV 檔案之前,我們需要將行值分配給一個列表。
例如,
list = ["4", "Alex Smith", "Science"]
接下來,將 List 中的資料作為引數傳遞給 CSV writer()
物件的 writerow()
函式。
例如,
csvwriter_object.writerow(list)
先決條件:
-
CSV
writer
類必須從CSV
模組匯入。from csv import writer
-
在執行程式碼之前,必須手動關閉 CSV 檔案。
示例 - 使用 writer.writerow()
將列表中的資料附加到 CSV 檔案
這是一個程式碼示例,顯示瞭如何將列表中存在的資料附加到 CSV 檔案中 -
# Pre-requisite - Import the writer class from the csv module
from csv import writer
# The data assigned to the list
list_data = ["03", "Smith", "Science"]
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the writer() function
writer_object = writer(f_object)
# Result - a writer object
# Pass the data in the list as an argument into the writerow() function
writer_object.writerow(list_data)
# Close the file object
f_object.close()
假設在執行程式碼之前;舊的 CSV 檔案包含以下內容。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
程式碼執行後,CSV 檔案將被修改。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
使用 DictWriter.writerow()
將字典中的資料附加到 Python 中的 CSV 檔案
在這種情況下,在我們將新行附加到舊 CSV 檔案之前,將行值分配給字典。
例如,
dict = {"ID": 5, "NAME": "William", "SUBJECT": "Python"}
接下來,將字典中的這些資料作為引數傳遞給字典 DictWriter()
物件的 writerow()
函式。
例如,
dictwriter_object.writerow(dict)
先決條件:
-
DictWriter
類必須從CSV
模組匯入。from csv import DictWriter
-
在執行程式碼之前,必須手動關閉 CSV 檔案。
示例 - 使用 DictWriter.writerow()
將字典中的資料附加到 CSV 檔案
下面是一個程式碼示例,它展示瞭如何將字典中存在的資料附加到 CSV 檔案中。
# Pre-requisite - Import the DictWriter class from csv module
from csv import DictWriter
# The list of column names as mentioned in the CSV file
headersCSV = ["ID", "NAME", "SUBJECT"]
# The data assigned to the dictionary
dict = {"ID": "04", "NAME": "John", "SUBJECT": "Mathematics"}
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the Dictwriter() function
# Result - a DictWriter object
dictwriter_object = DictWriter(f_object, fieldnames=headersCSV)
# Pass the data in the dictionary as an argument into the writerow() function
dictwriter_object.writerow(dict)
# Close the file object
f_object.close()
假設在執行程式碼之前,舊的 CSV 檔案包含以下內容。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
程式碼執行後,CSV 檔案將被修改。
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
04,John,Mathematics
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe