在 Python 中合併 CSV 檔案
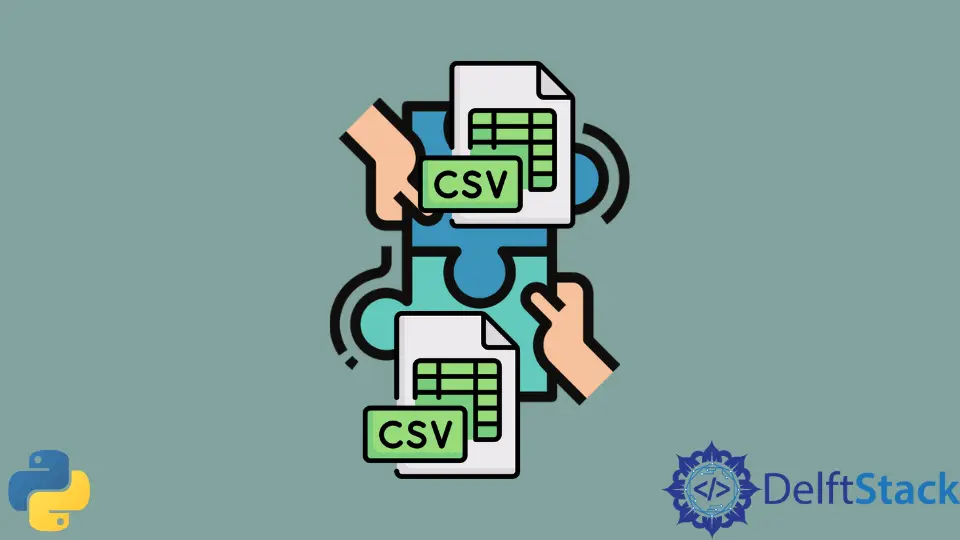
在 Pandas DataFrame
中處理 .csv 檔案形式的大型資料集時,單個檔案可能不包含用於資料分析的完整資訊。在這種情況下,我們需要在單個 Pandas DataFrame
中合併多個檔案。Python pandas 庫提供了各種方法來解決這個問題,例如 concat
、merge
和 join
。
在本指南中,我們將學習兩種不同的方法,藉助不同的示例將多個 .csv 檔案合併到一個 Pandas DataFrame
中。
使用按名稱合併將多個 CSV 檔案合併到單個 Pandas DataFrame
中
要合併多個 .csv 檔案,首先,我們匯入 pandas 庫並設定檔案路徑。然後,使用 pd.read_csv()
方法讀取所有 CSV 檔案。pd.concat()
將對映的 CSV 檔案作為引數,然後預設沿行軸合併它們。ignore_index=True
引數用於為新合併的 DataFrame
設定連續索引值。
請參閱以下示例,我們使用 pandas python 實現了上述方法:
示例程式碼:
import pandas as pd
# set files path
sales1 = "C:\\Users\\DELL\\OneDrive\\Desktop\\salesdata1.csv"
sales2 = "C:\\Users\DELL\\OneDrive\\Desktop\\salesdata2.csv"
print("*** Merging multiple csv files into a single pandas dataframe ***")
# merge files
dataFrame = pd.concat(map(pd.read_csv, [sales1, sales2]), ignore_index=True)
print(dataFrame)
輸出:
*** Merging multiple csv files into a single pandas dataframe ***
Product_Name Quantity Sale_Price
0 Acer laptop 3 500$
1 Dell Laptop 6 700$
2 Hp laptop 8 800$
3 Lenavo laptop 2 600$
4 Acer laptop 3 500$
5 Dell Laptop 6 700$
6 Hp laptop 8 800$
7 Lenavo laptop 2 600$
通過合併所有欄位在單個 Pandas DataFrame
中合併多個 CSV 檔案
為了合併 Pandas DataFrame
中的所有 .csv 檔案,我們在這種方法中使用了 glob 模組。首先,我們必須匯入所有庫。之後,我們為所有需要合併的檔案設定路徑。
在以下示例中,os.path.join()
將檔案路徑作為第一個引數,將要連線的路徑元件或 .csv 檔案作為第二個引數。在這裡,salesdata*.csv
將匹配並返回指定主目錄中以 salesdata
開頭並以 .csv 副檔名結尾的每個檔案。glob.glob(files_joined)
接受合併檔名的引數並返回所有合併檔案的列表。
請參閱以下示例以使用 glob 模組合併所有 .csv 檔案:
示例程式碼:
import pandas as pd
import glob
import os
# merging the files
files_joined = os.path.join(
"C:\\Users\\DELL\\OneDrive\\Desktop\\CSV_files", "salesdata*.csv"
)
# Return a list of all joined files
list_files = glob.glob(files_joined)
print("** Merging multiple csv files into a single pandas dataframe **")
# Merge files by joining all files
dataframe = pd.concat(map(pd.read_csv, list_files), ignore_index=True)
print(dataframe)
輸出:
** Merging multiple csv files into a single pandas dataframe **
Product_Name Quantity Sale_Price
0 Acer laptop 3 500$
1 Dell Laptop 6 700$
2 Hp laptop 8 800$
3 Lenavo laptop 2 600$
4 Acer laptop 3 500$
5 Dell Laptop 6 700$
6 Hp laptop 8 800$
7 Lenavo laptop 2 600$
まとめ
我們在本教程中介紹了兩種在 pandas python 中合併多個 CSV 檔案的方法。我們已經看到了如何使用 pd.concat()
方法讀取 .csv 檔案並將它們合併到單個 Pandas DataFrame
中。此外,我們現在知道如何在 Pandas python 程式碼中使用 glob
模組。