How to Print an Object of a Class in Python
-
Print an Object in Python Using the
__repr__()
Method -
Print an Object in Python Using the
__str__()
Method - Print an Object in Python by Adding New Class Method
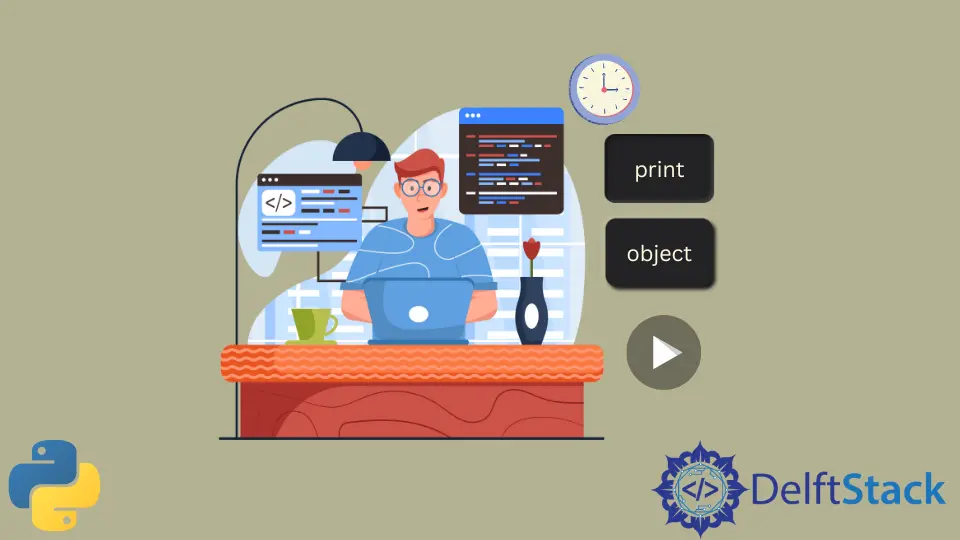
In this tutorial, we will look into multiple methods to print a class object in Python. Suppose we want to print a class instance using the print()
function, like viewing the object’s data or values. We can do so by using the methods explained below.
Print an Object in Python Using the __repr__()
Method
The __repr__()
method returns the object’s printable representation in the form of a string. It, by default, returns the name of the object’s class and the address of the object.
When we print an object in Python using the print()
function, the object’s __str__()
method is called. If it is not defined then the __str__()
returns the return value of the __repr__()
method. That is why when we try to print an object of a user-defined class using the print()
function, it returns the return value of the __repr__()
method.
Therefore, we can define or override the __repr__()
method to print an object’s summary or desired values.
Suppose we have a user-defined class ClassA
with no __repr__()
method, the below example code demonstrates the output when we try to print an object of the ClassA
with no __repr__()
method.
class classA:
def __init__(self):
self.var1 = 0
self.var2 = "Hello"
A = classA()
print(A)
Output:
<__main__.classA object at 0x7fa29aa28c50>
As we have not defined the __repr__()
method, it has by default returned the name of the class and address of the object.
Now, let’s define the __repr__()
method of the class classA
, and then use the print()
function. The print()
and print(repr())
should return the same value.
class classA:
def __init__(self):
self.var1 = 0
self.var2 = "Hello"
def __repr__(self):
return "This is object of class A"
A = classA()
print(A)
print(repr(A))
Output:
This is object of class A
This is object of class A
Print an Object in Python Using the __str__()
Method
The __str__()
method returns the string version of the object in Python. If an object does not have a __str__()
method, it returns the same value as the __repr__()
method.
We have seen in the above example codes, in case the __str__()
method is not defined, the print()
method prints the printable representation of the object using the __repr__()
method.
Now, let’s define the __str__()
method of our example class ClassA
and then try to print the object of the classA
using the print()
function. The print()
function should return the output of the __str__()
method.
class classA:
def __init__(self):
self.var1 = 0
self.var2 = "Hello"
def __repr__(self):
return "This is object of class A"
def __str__(self):
print("var1 =", self.var1)
print("var2 =", end=" ")
return self.var2
A = classA()
print(A)
Output:
var1 = 0
var2 = Hello
Print an Object in Python by Adding New Class Method
Another approach can be used instead of override or define the __str__()
and __repr__()
methods of the class. A new print() method can be described within the class, which will print the class attributes or values of our choice.
The below example code demonstrates how to define and then use the object.print()
method to print an object in Python.
class classA:
def __init__(self):
self.var1 = 0
self.var2 = True
self.var3 = "Hello"
def print(self):
print("var1 =", self.var1)
print("var2 =", self.var2)
print("var3 =", self.var3)
A = classA()
A.var2 = False
A.print()
Output:
var1 = 0
var2 = False
var3 = Hello