How to Object Tracking Using OpenCV
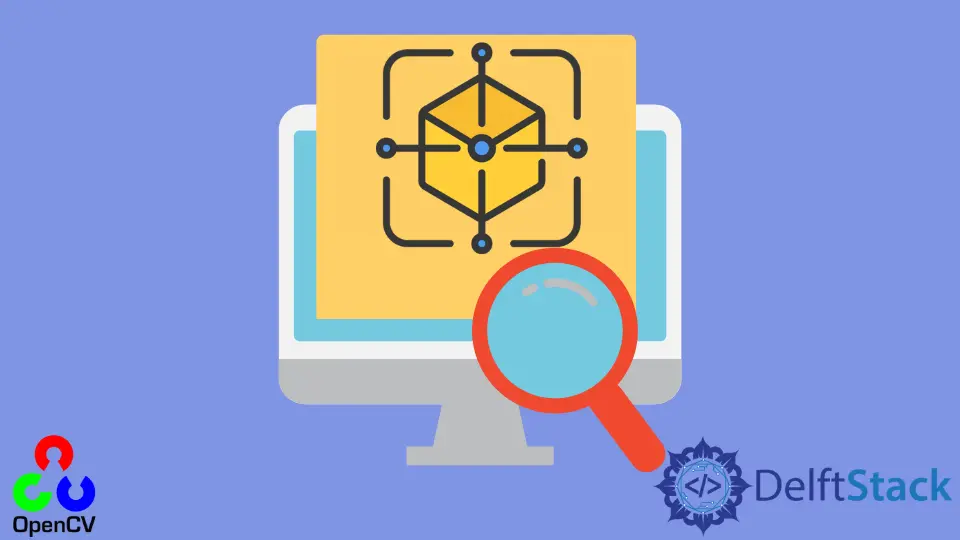
Object tracking is one of the important tasks in Computer Vision that has many real-world applications. In object tracking, we try to distinguish different objects based on some given parameters and track their movement.
Remember that object tracking is different than object detection. This is because object tracking is much faster as we do not tend to detect the object in every frame, which increases the computational load.
The most common real-life application of object tracking is tracking the movement of vehicles on the roads. We can identify different vehicles and observe whether they are obeying the rules of the road or not by tracking their trajectory.
We can use the opencv
library to work with object tracking problems in Python. This tutorial will discuss the different trackers available in opencv
for object tracking.
Trackers for Object Tracking in OpenCV
The first tracker is the cv2.TrackerBoosting_create
based on the AdaBoost algorithm of Machine Learning. It learns using the positive and negative samples of the object to be tracked on runtime, and it does not have a good track record in recent times and is considered very slow.
Then, we have the cv2.TrackerMIL_create
tracker based on the MIL algorithm. It was built as an improvement to the BOOSTING tracker and is based on similar concepts and factors in the neighborhood of positive samples to distinguish objects improving the overall accuracy.
The cv2.TrackerKCF_create
tracker is based on the mathematical approach to the overlapping regions of multiple positive matches in a MIL. The full form of KCF is Kernelized Correlation Filters, and it is considered a decent tracker and works very well in tracking single objects.
The cv2.TrackerCSRT_create
tracker is based on the Discriminative Correlation Filter with channel and spatial reliability. It filters out parts of the frame using the spatial reliability map that helps select the required object, and for lower frame rates, it gives a resounding high accuracy.
The cv2.TrackerMedianFlow_create
tracker is an advanced tracker that aims to minimize tracking failure and choose the most reliable path. An object is detected in real-time, its displacement for forwarding and backward motion is calculated, and the measurement of error and difference between the two values is compared to track the trajectory of the given object.
We also have the cv2.TrackerTLD_create
tracker. TLD stands for tracking, learning, and detecting, and it follows the trajectory of an object frame by frame. It uses the position from the previous frame to correct and improve the tracking.
The detector part is used to rectify the tracker if required using the previous frame. It handles object overlapping very efficiently.
The cv2.TrackerMOSSE_create
tracker, where MOSSE
stands for Minimum Output Sum of Squared Error, uses adaptive correlations in Fourier space to track objects. It uses these correlation values and tries to minimize the sum of squared errors between actual and predicted correlation.
This tracker has a very high tracking speed and adapts well to changes in light and the surroundings of the object.
Use OpenCV Object Trackers in Python
We will use the cv2.TrackerKCF_create
tracker in our example to track an object. We’ll start by reading the sample video using the capture()
function.
Then, we initialize a variable that keeps track of the object. Initially, it is assigned as None
and runs a loop that reads this video frame by frame.
Initially, we check if there was tracking done in the previous frame and continue with it if the initialized variable is not None
. If not, we select the object we want to track using the selectROI
function.
After that, we use the defined tracker object to track this object in every frame and display tracking information.
Code Example:
import cv2
import imutils
vid = cv2.VideoCapture("sample1.mp4")
initial_box = None
tracker = cv2.TrackerKCF_create()
while True:
fr = vid.read()
if fr is None:
break
if initial_box is not None:
(success, box) = tracker.update(fr)
if success:
(x, y, w, h) = [int(v) for v in box]
cv2.rectangle(fr, (x, y), (x + w, y + h), (0, 255, 0), 2)
fps.update()
fps.stop()
information = [
("Success", "Yes" if success else "No"),
("FPS", "{:.3f}".format(fps.fps())),
]
for (i, (k, v)) in enumerate(information):
text = "{}: {}".format(k, v)
cv2.putText(
fr,
text,
(10, H - ((i * 20) + 20)),
cv2.FONT_HERSHEY_SIMPLEX,
0.6,
(0, 0, 255),
2,
)
cv2.imshow("Output Frame", fr)
key = cv2.waitKey(1) & 0xFF
initial_box = cv2.selectROI("fr", fr, fromCenter=False, showCrosshair=True)
tracker.init(fr, initial_box)
fps = FPS().start()
cv2.destroyAllWindows()
Conclusion
We discussed the different algorithms used for object tracking available in the opencv
library. First, we discussed object tracking and its uses in real life.
Then, we discussed the eight available trackers of the opencv
library in detail and a sample code on handling object tracking using these trackers.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn