Lexicographical Order in Python
- Lexicographic Order in Python
-
Sorting a List of Strings in Lexicographic Order Using the
sort()
Method in Python -
Sorting a String Into Lexicographic Order Using the
split()
andsort()
in Python -
Sorting Numeric Lists Into Lexicographic Order Using
sort()
andsorted()
in Python -
Sorting Characters in a String Into Lexicographic Order Using
sorted()
in Python - Sorting Tuples in Lexicographic Order Based on Fruit Names in Python
- Conclusion
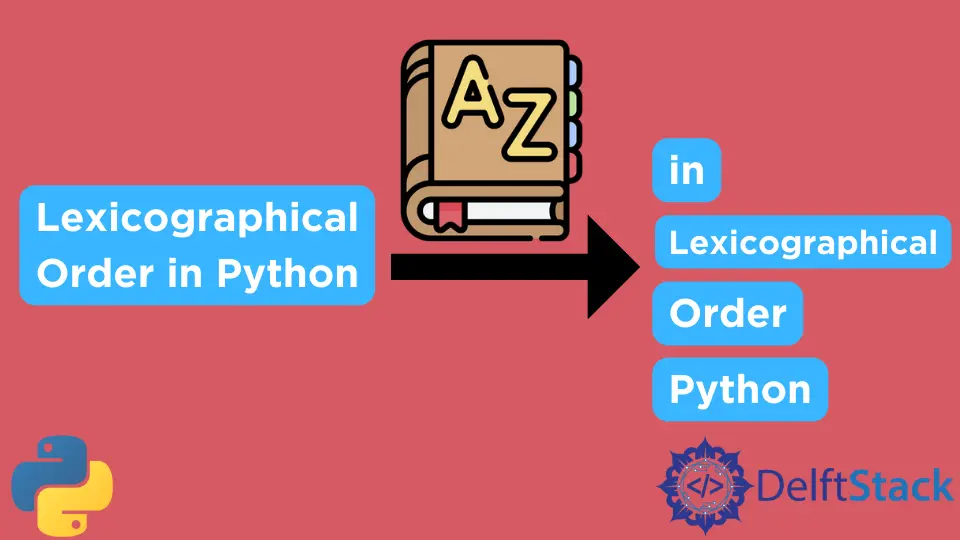
We will introduce lexicographic order
in Python. We’ll also discuss different methods to achieve lexicographic order with examples.
Lexicographic Order in Python
In math, the lexicographic
or lexicographical order
is the process of ordering a list of elements or an array of elements that are arranged alphabetically. The other term used for lexicographic order
is dictionary order
.
The lexicographic order
has a variety of forms and generalizations that can be used. In simple words, lexicographic ordering is sorting words from a list or array based on their first letters.
If the initial letter is identical, the second letter is utilized to order the words. We may come across some situations in which we need to sort the data according to our requirements, and we’ll use lexicographical order to sort the data.
Let’s discuss lexicographic order
with examples to understand it better.
Sorting a List of Strings in Lexicographic Order Using the sort()
Method in Python
The sort()
method belongs to the list data type, and it sorts the elements of the list in place and does not return a new list. The sort()
method modifies the original list in place, returns None
, and directly alters the order of elements within the existing list.
Basic Syntax:
list_name.sort(key)
In the syntax, the parameter is the key
parameter (optional), which allows you to specify a custom function to determine the sorting order.
As shown below, we will create a sample list containing the names of some random things that we will sort using the sort()
method.
Code:
sampleData = ["Egg", "Milkshake", "Banana", "Apple"]
print("List before using Lexicographical Order: ", sampleData)
sampleData.sort()
print("List after using Lexicographical Order: ", sampleData)
Output:
List before using Lexicographical Order: ['Egg', 'Milkshake', 'Banana', 'Apple']
List after using Lexicographical Order: ['Apple', 'Banana', 'Egg', 'Milkshake']
In this code, we begin with a list named sampleData
containing strings representing items. We initiate the process by printing the original list using the statement print("List before using Lexicographical Order: ", sampleData)
.
Subsequently, we apply the sort()
method to the list, which arranges the elements in lexicographical (alphabetical) order. The result is printed.
The output showcases the sorted list, demonstrating the modification made in place by the sort()
method. The final list appears as ['Apple', 'Banana', 'Egg', 'Milkshake']
.
Sorting a String Into Lexicographic Order Using the split()
and sort()
in Python
The split()
is used to split a string into individual words and then sort those words in lexicographic (alphabetical) order using sort()
. In this next example, we’ll use a string instead of a list to apply lexicographic order
.
Code:
def LexicographicSort(sampleData):
Data = sampleData.split()
Data.sort()
for a in Data:
print(a)
sampleData = "Let's try to sort this string into Lexicographical order"
print("String before using Lexicographical Order: ", sampleData)
print("String after using Lexicographical Order: ")
LexicographicSort(sampleData)
Output:
String before using Lexicographical Order: Let's try to sort this string into Lexicographical order
String after using Lexicographical Order:
Let's
Lexicographical
into
order
sort
string
this
to
try
In this code, we define a function LexicographicSort
that takes a string (sampleData
) as input. Inside the LexicographicSort
function, we use the split()
method to break the string into individual words and store them in a list called Data
.
We then apply the sort()
method to arrange these words in lexicographical order, and the subsequent loop prints each word on a new line. Moving to the main part of the code, we initialize a string (sampleData
) and print it as "String before using Lexicographical Order"
.
Following this, we print "String after using Lexicographical Order"
to indicate the upcoming output. Finally, we call the LexicographicSort
function with the original string as an argument and you can see in the output it displays each word sorted in lexicographical order, presenting a clear transformation of the string.
Sorting Numeric Lists Into Lexicographic Order Using sort()
and sorted()
in Python
We can sort numerical lists using the sort()
and sorted()
. In this first example, we will use a random array of numbers that we can sort using the sort()
method.
Code - sort()
:
def LexicographNumberSort(RangedNum):
RangedNum.sort()
print(RangedNum)
RangedNum = [1, 4, 5, 3, 10, 16, 12]
print("Sorted numbers: ")
LexicographNumberSort(RangedNum)
Output:
Sorted numbers:
[1, 3, 4, 5, 10, 12, 16]
In the code above, we define a function called LexicographNumberSort
that takes a list of numbers (RangedNum
) as input. Inside the function, we use the sort()
method to rearrange the numbers in ascending order, modifying the original list in place, and after sorting, the function prints the sorted list.
Moving to the main part of the code, we initialize a list of numbers (RangedNum
) and print "Sorted numbers:"
to signify the subsequent output. We then call the LexicographNumberSort
function with the original list as an argument.
The output displays the numbers in sorted order, [1, 3, 4, 5, 10, 12, 16]
.
Now, let’s have another example by using the sorted()
function. The sorted()
is a Python built-in function that can be applied to any iterable (lists, tuples, strings, etc.), and it returns a new sorted list and does not modify the original iterable.
Code - sorted()
:
def LexicographNumberSort(RangedNum):
sorted_numbers = sorted(RangedNum)
print(sorted_numbers)
RangedNum = [1, 4, 5, 3, 10, 16, 12]
print("Sorted numbers: ")
LexicographNumberSort(RangedNum)
Output:
Sorted numbers:
[1, 3, 4, 5, 10, 12, 16]
In this code above, we define a function named LexicographNumberSort
that takes a list of numbers (RangedNum
) as input. Inside the function, we use the sorted()
function to generate a newly sorted list without modifying the original list.
We assign this sorted list to the variable sorted_numbers
. The function then prints the newly sorted list. Then, we call the LexicographNumberSort
function with the original list as an argument to display the numbers that are in ascending order, illustrating the effect of the sorted()
function on the list.
The final sorted list is presented as [1, 3, 4, 5, 10, 12, 16]
.
In the examples above, the difference between the two is that sorted()
creates a new sorted list, leaving the original list unchanged. On the other hand, sort()
modifies the original list in place, and you can see the change directly in the original list itself.
Sorting Characters in a String Into Lexicographic Order Using sorted()
in Python
We can also sort characters in a string lexicographically using the sorted()
function. When the function is applied to a string, sorted()
rearranges its characters based on their Unicode code points.
Code:
my_string = "delftstack"
sorted_string = "".join(sorted(my_string))
print(sorted_string)
Output:
acdefklstt
In this code, we begin by initializing a string variable named my_string
with the value "delftstack"
. We then use the sorted()
function to rearrange the characters in the string in lexicographic (alphabetical) order.
The result, stored in the variable sorted_string
, is obtained by joining the sorted characters together using the join()
method. Finally, we print the sorted_string
, and the output of this code displays the characters of my_string
in lexicographically sorted order, acdefklstt
.
Sorting Tuples in Lexicographic Order Based on Fruit Names in Python
Sorting tuples in lexicographic order in Python refers to arranging a list of tuples containing both numeric values and strings in a specific order determined by dictionary or alphabetical rules.
In this following example, lexicographic order means sorting the tuples based on the fruit names in ascending order, as they would appear in a dictionary.
Code:
from operator import itemgetter
list_of_tuples = [(1, "apple"), (3, "orange"), (2, "banana")]
sorted_tuples = sorted(list_of_tuples, key=itemgetter(1))
print(sorted_tuples)
Output:
[(1, 'apple'), (2, 'banana'), (3, 'orange')]
In this code, we use the sorted()
function to sort a list of tuples named list_of_tuples
. Each tuple contains a numeric value and a string representing a fruit. We specify the key
parameter as itemgetter(1)
, indicating that the sorting should be based on the second element (index 1) of each tuple, which corresponds to the fruit names.
Consequently, the tuples are sorted in the lexicographic order of the fruit names. The output of the code displays the sorted tuples, showcasing the arrangement of fruits in ascending order as [(1, 'apple'), (2, 'banana'), (3, 'orange')]
.
Sorting tuples in lexicographic order can be recommendable in situations where readability, consistency, and adherence to alphabetical order are important. However, as with any programming decision, it’s crucial to consider the contents and specific requirements of your application.
Conclusion
In this article, we explored lexicographic order in Python, a method vital for alphabetically sorting elements.
Demonstrating various techniques, we covered sorting lists, strings, numeric values, and tuples. Examples included using the sort()
method, combining split()
and sort()
, and employing sorted()
.
The discussion highlighted the significance of lexicographic sorting for readability and consistency. Whether dealing with fruits, numbers, or strings, the article provides practical insights for developers seeking clear and meaningful data organization in Python.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn