How to Iterate Through JSON Object in Python
-
Use
json.loads()
With thefor
Loop to Iterate Through a JSON Object in Python -
Use
json.loads()
With theitems()
Method to Iterate Through a JSON Object in Python - Use a Recursive Function to Iterate Through a JSON Object in Python
-
Use the
jsonpath
Library to Iterate Through a JSON Object in Python -
Use the
Pandas
Library to Iterate Through a JSON Object in Python - Conclusion
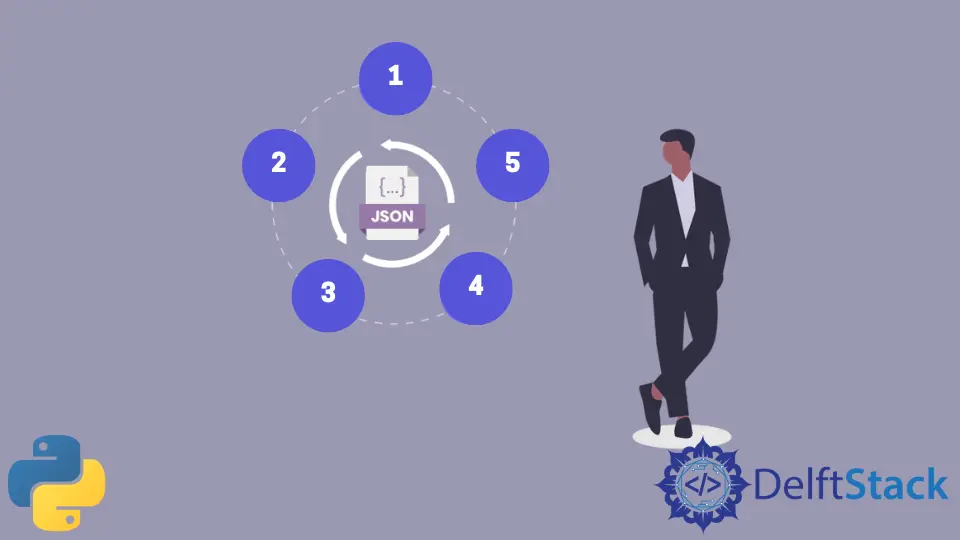
JSON (JavaScript Object Notation) is a widely used data format for data storage and exchange. When working with JSON objects in Python, it’s essential to understand how to iterate through their contents.
In this tutorial, we’ll explore the process of iterating through a JSON object using various methods.
Use json.loads()
With the for
Loop to Iterate Through a JSON Object in Python
Before diving into the code, it’s important to understand the basics of working with JSON in Python.
The json
module, part of the Python standard library, provides methods for working with JSON-formatted data. Specifically, the json.loads()
function is used to parse a JSON-formatted string into a Python dictionary.
To iterate through a JSON object in Python, we first need to convert the JSON data into a format that Python understands. The json.loads()
method is used to parse a JSON-formatted string into a Python dictionary.
Once the JSON data is in dictionary form, we can employ a for
loop to iterate through its keys and access the corresponding values.
Let’s consider a scenario where we have a JSON string representing key-value pairs, and we want to iterate through it using a for
loop.
import json
json_string = '{"name": "Joey", "age": 30, "city": "New York"}'
json_data = json.loads(json_string)
for key in json_data:
print(key, ":", json_data[key])
In this Python code snippet, we begin by importing the json
module, which provides functionalities for working with JSON-formatted data. The next line defines a JSON-formatted string (json_string
) containing key-value pairs representing information about an individual, including their name, age, and city of residence.
The json.loads()
function is then applied to the JSON string, converting it into a Python dictionary named json_data
. This process essentially parses the JSON string and creates a dictionary with keys and values corresponding to the JSON structure.
The core of the code involves a for
loop that iterates through the keys of the json_data
dictionary. During each iteration, the loop prints out the current key, followed by a colon, and the corresponding value obtained by accessing the dictionary with json_data[key]
.
Finally, when the code is executed, the output will display the keys and values of the JSON object:
name : Joey
age : 30
city : New York
This output demonstrates a successful iteration through the JSON object, printing each key-value pair in the specified format.
Use json.loads()
With the items()
Method to Iterate Through a JSON Object in Python
The items()
method is a powerful feature in Python when working with dictionaries. It has the following syntax:
dictionary.items()
Here, dictionary
is the dictionary for which you want to retrieve the key-value pairs. The items()
method returns a view object that displays a list of a dictionary’s key-value tuple pairs.
In the context of JSON objects loaded into Python dictionaries, items()
allows us to directly access key-value pairs. This method simplifies the iteration process.
To iterate through a JSON object using the items()
method, we first load the JSON data using the json.loads()
function, converting it into a Python dictionary. The items()
method is then applied to the dictionary.
Consider a scenario where we have a JSON string representing a person’s details, and we want to iterate through it using the items()
method.
import json
json_string = '{"name": "Alice", "age": 25, "city": "London"}'
json_data = json.loads(json_string)
for key, value in json_data.items():
print(key, ":", value)
Here, we define a JSON-formatted string (json_string
) containing key-value pairs representing a person’s details. Using json.loads()
, we convert this string into a Python dictionary (json_data
).
The essential part of this example is the for
loop. Instead of using the more traditional approach of iterating through keys and accessing values separately, we employ the items()
method.
This method returns an iterable of key-value pairs, allowing us to directly unpack and print both the key and value within the loop.
Output:
name : Alice
age : 25
city : London
In this output, each line corresponds to a key-value pair in the JSON object.
Use a Recursive Function to Iterate Through a JSON Object in Python
In certain scenarios, JSON objects can be nested, containing structures within structures. When faced with such complexities, a recursive approach becomes valuable for navigating through the nested layers.
Recursion is a programming concept where a function calls itself to solve a smaller instance of the problem until it reaches a base case.
To iterate through a JSON object using recursion, we define a recursive function that takes an element of the JSON object as an argument. This function checks the type of the element, distinguishing between dictionaries and lists.
For dictionaries, the function iterates through the keys and recursively calls itself with the corresponding values. For lists, the function iterates through the items and, again, calls itself recursively for each item.
Consider a scenario where we have a JSON string representing a person’s details, including nested information about their address. The goal is to iterate through this nested JSON object using recursion.
import json
def iterate_json(obj):
if isinstance(obj, dict):
for key, value in obj.items():
print(key, ":", value)
iterate_json(value)
elif isinstance(obj, list):
for item in obj:
iterate_json(item)
json_string = (
'{"name": "Joey", "age": 30, "address": {"city": "New York", "zip": "10001"}}'
)
json_data = json.loads(json_string)
iterate_json(json_data)
In this example, the iterate_json
function is defined to handle the recursive iteration process. The function checks if the input object is a dictionary or a list.
If it’s a dictionary, it iterates through the keys and values, prints them, and then recursively calls itself with the value. If it’s a list, the function iterates through the items and recursively calls itself for each item.
The JSON string representing a person’s details, including nested address information, is loaded into a Python dictionary (json_data
). The iterate_json
function is then called with this dictionary, initiating the recursive iteration process.
Output:
name : Joey
age : 30
address : {'city': 'New York', 'zip': '10001'}
city : New York
zip : 10001
This output demonstrates the successful recursive iteration through the nested JSON object. Each key-value pair, including those nested within the address
key, is printed in a structured manner.
The recursive approach ensures that all levels of the JSON object are traversed, providing a comprehensive exploration of the data structure.
Use the jsonpath
Library to Iterate Through a JSON Object in Python
When dealing with intricate JSON structures, a more expressive and XPath-like approach can simplify navigation. The jsonpath
library allows us to use XPath-like expressions to query and navigate JSON structures.
This approach is particularly beneficial when dealing with complex JSON objects, as it provides a concise and flexible syntax for selecting specific elements.
Before using the jsonpath
library, it needs to be installed. This can be done using the following pip
command:
pip install jsonpath-ng
Once the jsonpath
library is installed, we can define a JSONPath expression to specify the elements we want to extract from the JSON object. The library then returns the selected elements, allowing us to iterate through them effortlessly.
Consider a scenario where we have a JSON string representing a person’s details, including nested information about their address. The goal is to use the jsonpath
library to selectively iterate through this JSON object.
from jsonpath_ng import jsonpath, parse
import json
json_string = (
'{"name": "Joey", "age": 30, "address": {"city": "New York", "zip": "10001"}}'
)
json_data = json.loads(json_string)
expression = parse("$.address.*")
matches = [match.value for match in expression.find(json_data)]
for match in matches:
print(match)
In this example, we import the necessary modules, including jsonpath
from the jsonpath_ng
library and the standard json
module. We define a JSONPath expression ("$.address.*"
) using the parse()
function from jsonpath_ng
, specifying that we want to select all elements within the address
key.
The expression is then applied to the JSON data using the find()
method, and the selected elements are stored in the matches list. Finally, a for
loop iterates through the matched elements, printing each one.
Output:
New York
10001
This output demonstrates the successful iteration through the selected elements using the jsonpath
library. The expressive JSONPath expression allows us to precisely target and extract the desired components from the JSON object.
Use the Pandas
Library to Iterate Through a JSON Object in Python
When working with JSON objects that resemble tabular data, the Pandas
library in Python provides an efficient and organized way to iterate through the data. This library is a powerful data manipulation and analysis library in Python, commonly used for working with structured data.
It introduces the DataFrame
, a two-dimensional labeled data structure, which is particularly useful for handling JSON objects that exhibit a tabular structure.
Before using the Pandas
library, it needs to be installed. This can be accomplished using the following pip
command:
pip install pandas
Once Pandas
is installed, we can load the JSON object into a Pandas DataFrame
using the pd.json_normalize()
function. This function transforms the nested JSON object into a flat table, making it easier to iterate through rows or columns using Pandas
’ built-in functionalities.
Consider a scenario where we have a JSON string representing a list of people with their names, ages, and addresses.
import pandas as pd
import json
json_string = (
'[{"name": "Joey", "age": 30, "address": {"city": "New York", "zip": "10001"}}, '
'{"name": "Alice", "age": 25, "address": {"city": "London", "zip": "SW1A 1AA"}}]'
)
df = pd.json_normalize(json.loads(json_string))
for index, row in df.iterrows():
print(row)
In this example, we first import the Pandas
library as pd
and the standard json
module. The JSON string representing a list of people with nested addresses is loaded into a Pandas DataFrame
using pd.json_normalize()
.
The pd.json_normalize()
function flattens the nested JSON structure, creating a DataFrame
with columns for each key.
The iterrows()
function is then used to iterate through the DataFrame
rows. For each row, the loop prints the data, providing a convenient way to access and manipulate individual records.
Output:
name Joey
age 30
address.city New York
address.zip 10001
Name: 0, dtype: object
name Alice
age 25
address.city London
address.zip SW1A 1AA
Name: 1, dtype: object
This output demonstrates the successful iteration through the JSON object using Pandas
. The DataFrame
structure facilitates the traversal of the data, allowing us to handle tabular JSON objects with ease and flexibility.
Keep in mind that while iterrows()
is a convenient way to iterate through rows, it may not be the most efficient method for large DataFrames
. In such cases, other Pandas
functions or vectorized operations may offer better performance.
Conclusion
Whether your JSON object is simple or complex, nested or tabular, these methods offer flexibility and efficiency for iterating through the data. Choose the approach that best fits your specific JSON structure and processing requirements.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn