How to Fix Python Int Object Is Not Iterable Error
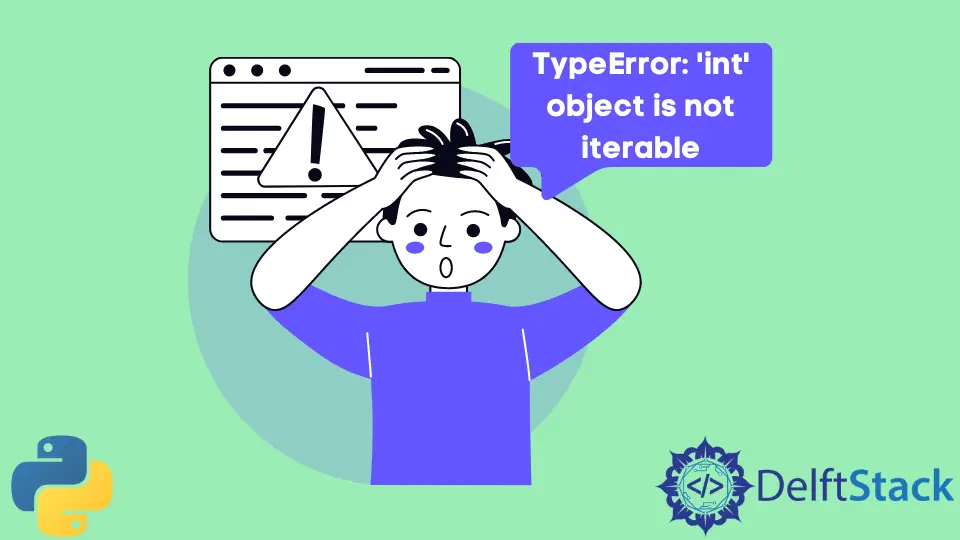
This error itself is self-explanatory. 'int' object is not iterable
, it’s clearly saying that you can’t run iteration on an integer. An integer is a single digit, not a list that is iterable. Let’s take a look at some examples.
Fix 'int' object is not iterable
Error in Python
Anything that returns or stores an integer is not iterable. That’s common knowledge. If you are not familiar with it, let’s understand iteration in python. Iterating can be done over a list, not on an integer. For instance, you can’t run a loop for iteration on an integer; it just doesn’t make sense. Take a look at the following code.
# integer
number = 123
# loop over an integer
for i in number:
print(i)
Running the above code will give you the same error you are trying to avoid. In the above code, number
is an integer having a single value 123
. You can’t run a loop over it. If you are confused about the data type and its relevant functionalities, you can easily resolve it by finding the magic methods. In this case, we’ll use an integer. Take a look.
# integer
number = 123
# built-in / magic methods of an integer
print(dir(number))
The output of the above code will be as follows.
[
"__abs__",
"__add__",
"__and__",
"__class__",
"__cmp__",
"__coerce__",
"__delattr__",
"__div__",
"__divmod__",
"__doc__",
"__float__",
"__floordiv__",
"__format__",
"__getattribute__",
"__getnewargs__",
"__hash__",
"__hex__",
"__index__",
"__init__",
"__int__",
"__invert__",
"__long__",
"__lshift__",
"__mod__",
"__mul__",
"__neg__",
"__new__",
"__nonzero__",
"__oct__",
"__or__",
"__pos__",
"__pow__",
"__radd__",
"__rand__",
"__rdiv__",
"__rdivmod__",
"__reduce__",
"__reduce_ex__",
"__repr__",
"__rfloordiv__",
"__rlshift__",
"__rmod__",
"__rmul__",
"__ror__",
"__rpow__",
"__rrshift__",
"__rshift__",
"__rsub__",
"__rtruediv__",
"__rxor__",
"__setattr__",
"__sizeof__",
"__str__",
"__sub__",
"__subclasshook__",
"__truediv__",
"__trunc__",
"__xor__",
"bit_length",
"conjugate",
"denominator",
"imag",
"numerator",
"real",
]
As you can see, you can’t find the iterator method in the above list. Let’s see what’s the difference when it comes to a list.
# list
lst = [1, 2, 3]
# loop over a list
for j in lst:
print(j)
# built-in /magic methods of a list
print(dir(lst))
The above code will not give any error. You can iterate over a list. If you run the above code, you will also notice the _iter_
function in it, stating that you can use iteration on a list.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python