How to Fix IndexError: Invalid Index to Scalar Variable
-
What Is the
IndexError: invalid index to scalar variable
in Python -
Fix the
IndexError: invalid index to scalar variable
in Python -
Fix the
IndexError: invalid index to scalar variable
in 2D Numpy Arrays
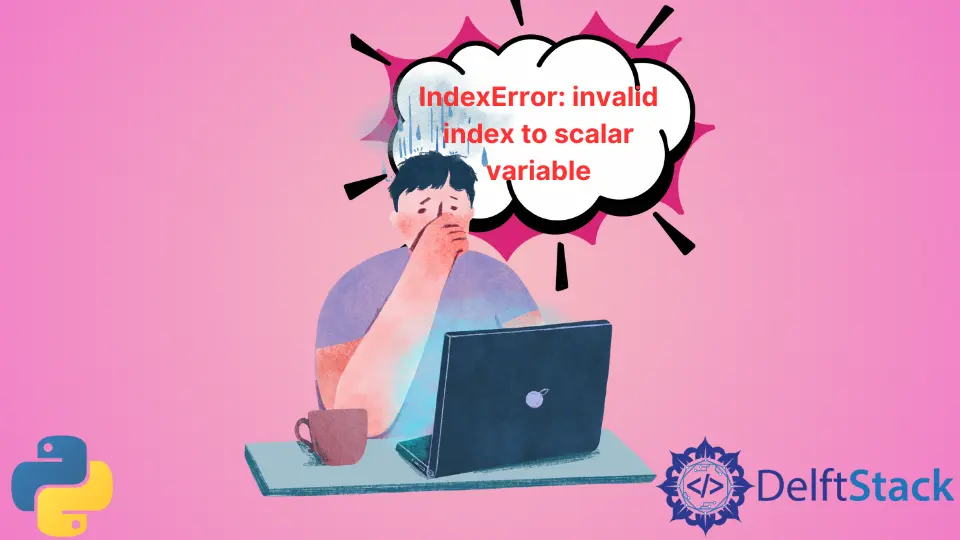
The IndexError
is too common, specifically when you are new to numpy
arrays. The index is the location of elements in an array.
It is easy when we have a simple array, but when the dimensions increase, the array becomes complex too. As the dimensional of an array increases, then indices increase too.
Let’s say when you have a simple array, you will require one index to access the elements, and in two-dimensional arrays, you will require two indices.
Example of the one and two-dimensional arrays:
One_D = [1, 2, 3, 4, 5]
print(One_D[0]) # --> 1
two_D = [[1, 2, 3], [4, 5, 6]]
print(two_D[1][0]) # --> 4
Output:
1
4
What Is the IndexError: invalid index to scalar variable
in Python
The IndexError: invalid index to scalar variable
in Python occurs when you misuse the indices of a numpy
array. Let’s say we have one-dimensional arr
.
import numpy as npy
arr = npy.array([1, 2, 3, 4, 5])
print(arr[0][1])
Output:
IndexError: invalid index to scalar variable.
In the above example, the array arr
requires only one index, but rather we are trying to access the elements with two indices [0][1]
, which doesn’t exist. Hence, it throws the IndexError: invalid index to scalar variable
.
Fix the IndexError: invalid index to scalar variable
in Python
Fixing the IndexError
is too simple and easy; the error itself is self-explanatory; it tells us that the issue is with the index and you are providing an invalid index to access the element.
We need to provide the right index according to the nature of the array. Let’s fix the IndexError
of the above program.
import numpy as npy
arr = npy.array([1, 2, 3, 4, 5])
print(arr[3])
Output:
4
Fix the IndexError: invalid index to scalar variable
in 2D Numpy Arrays
When you understand the working of an array, then two-dimensional is not a big deal to understand indices, and you are good to go.
Let’s take an example of a 2-D numpy
array.
import numpy as npy
# creating a 2-D array
arr = npy.array([[1, 2, 3], [4, 5, 6]])
# with 2 rows and 3 columns
print(arr.shape)
# arr[2nd row] [3rd column]
print(arr[1][2])
# print(arr[1][2][3]) --> IndexError: invalid index to scalar variable.
Output:
(2, 3)
6
In this example, we have a 2-D array arr
whose shape is (2,3)
means it has two rows and 3 columns, and we know that in computer programming languages, indices start with 0
, and it means 1
.
So the indices arr[1][2]
means accessing the array arr
element at the 2nd row and 3rd column, which is 6
.
And again, if you provide invalid indices like arr[1][2][3]
3 indices instead of 2 to the arr
array, this will throw the IndexError: invalid index to scalar variable
because that location does not exist in the arr
array.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python