How to Fix ImportError: Missing Required Dependencies Numpy
-
What Is the
ImportError: Missing required dependencies ['numpy']
in Python -
How to Fix the
ImportError: Missing required dependencies ['numpy']
in Python
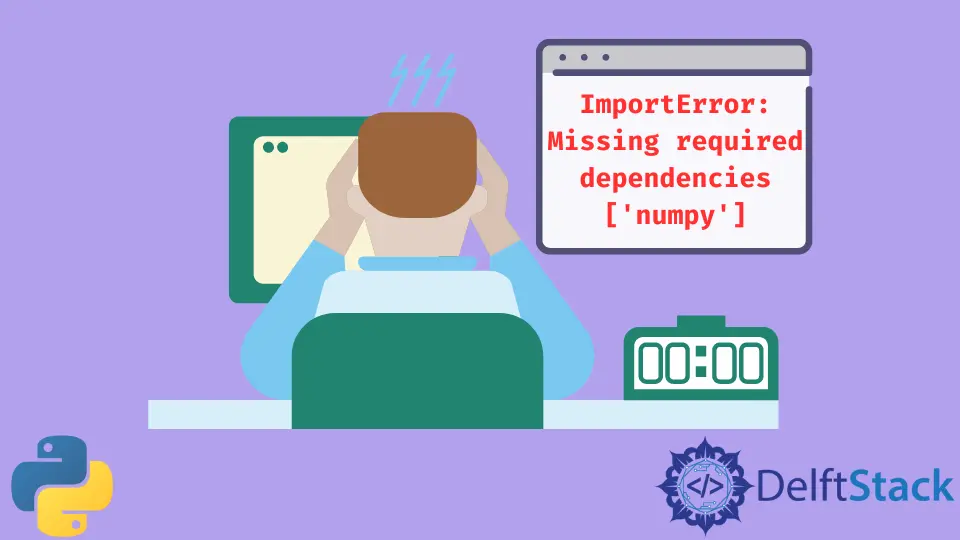
The ImportError
is a common error when new to Python libraries like pandas
, Numpy
, and TensorFlow
.
Some libraries need external packages and classes to download, so you must import them through the Command Line Interface (CLI) and then import them to your program using the import
keyword.
The syntax of importing a library is as follows.
import pandas as pd
The above line of code will import the pandas
library as pd
, and you can use pd
to access the different classes and functions of pandas
.
What Is the ImportError: Missing required dependencies ['numpy']
in Python
As we know, there are some modules, classes, packages, and libraries in Python that you can directly import into your program with the import
keyword.
But then we have some libraries and packages which you cannot directly import into your program, and if you try with the import
keyword, it will throw the ImportError
and ask you to import the required dependencies.
Let’s see an example of the ImportError: Missing required dependencies
.
import pandas as pd
Output:
ImportError: Missing required dependencies ['numpy']
In the above piece of code, we are importing pandas
as pd
, but it throws an ImportError: Missing required dependencies ['numpy']
, which means we are missing some dependencies either numpy
isn’t installed or we have an old version of pandas
that needs an update.
The pandas
capabilities are built on top of the numpy
library so in one way or the other, the numpy
is a dependency of the pandas
library, and that’s the reason to use pandas
you need to make sure to install numpy
as well.
How to Fix the ImportError: Missing required dependencies ['numpy']
in Python
There is a famous saying in the software engineering domain, “Change is constant”, which means in software, or any computer programs; the changes will be requested either by updating the modules or adding additional functionalities.
Similarly, the Python libraries and packages are continuously upgrading to include more functionalities and improve the current ones.
So there are a few commands you should run in the Command Line Interface (CLI) to install the libraries if they aren’t yet installed or update if they exist.
# install the numpy library
pip install numpy
#or
conda install numpy
# install the pandas library
pip install pandas
The above commands are used to install numpy
and pandas
; after importing, you can use the import
to import it into your current program.
If these libraries are installed on your machines and require an update, then you can use the below command to update the libraries.
# update numpy
pip install --upgrade numpy
# update pandas
pip install --upgrade pandas
If you are still facing the same errors, then it is suggested to uninstall the current versions of pandas
and numpy
and install them again using the pip
command.
# uninstalling pandas
pip uninstall pandas
# uninstalling numpy
pip uninstall numpy
You can use the famous pip
command to reinstall them again.
# re-installing pandas
pip install pandas
# re-installing numpy
pip install numpy
The above are a few solutions to fix the ImportError: Missing required dependencies ['numpy']
in Python.
import pandas as pd
import numpy as np
print("The version of pandas is:\t", pd.__version__)
print("The version of numpy is:\t", np.__version__)
Output:
The version of pandas is: 1.3.5
The version of numpy is: 1.22.0
The above commands have fixed the ImportError
as the program runs smoothly without causing any errors.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python ImportError
- How to Fix ImportError: No Module Named mysql.connector
- How to Fix ImportError: No Module Named Sklearn in Python
- How to Fix Python ImportError: No Module Named Requests
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python