How to Handle the Python Assertion Error and Find the Source of Error
- Handle the Assertion Error and Find the Source of Error in Python
-
Use the
Try-Except
Blocks to Handle the Assertion Error in Python -
Use the Logging Module With
Try-Except
Blocks to Handle the Assertion Error in Python - Use the Traceback Module to Handle the Assertion Error in Python
-
Use a
print
Statement to Handle theAssertionError
Exception Manually in Python - Conclusion
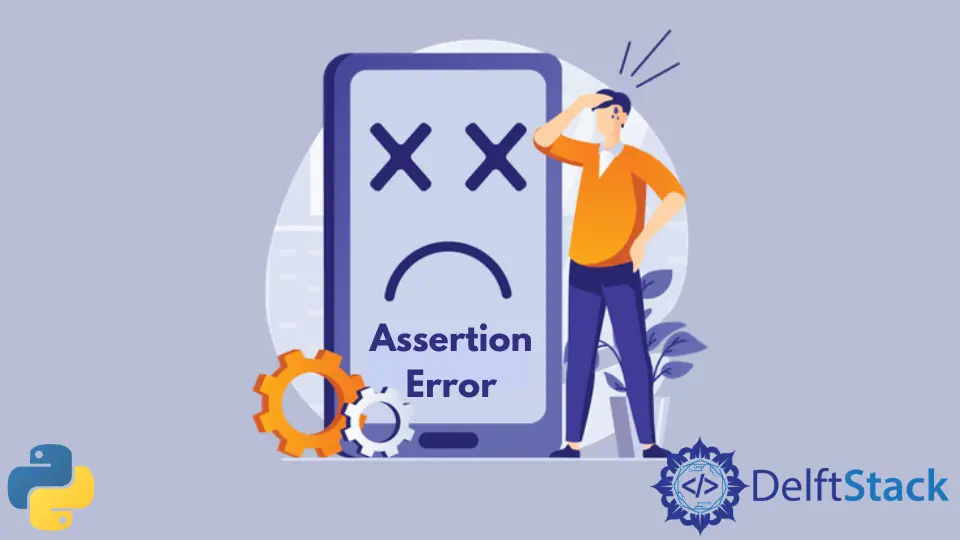
In this article, we learn how we can handle the assertion error of Python in different ways. We also see ways to identify the statement that raises this error.
Handle the Assertion Error and Find the Source of Error in Python
In Python, we can use the assert
statement to check any condition in a code. If the condition is True
, the control goes further.
But if the condition turns out to be False
, we get the AssertionError
, and the flow of the program is disrupted.
The syntax for the assert statement is as follows.
assert statement, message
Here, the statement
is a Boolean statement. If it evaluates to False
, the program raises AssertionError
.
The message
is optional and gets printed when AssertionError
occurs. If the statement
evaluates to True
, nothing happens.
It is how Python raises an AssertionError
exception.
assert True == False, "Whoops, something went wrong!"
print(True)
Output:
Traceback (most recent call last):
File "Desktop/Tut.py", line 2, in <module>
assert True == False, "Whoops, something went wrong!"
AssertionError: Whoops, something went wrong!
You can observe that we have used the statement True==False
, which evaluates to False
. Hence, the program raises the AssertionError
exception.
There are various ways in which we can handle this exception. Let us go through them one after one.
Use the Try-Except
Blocks to Handle the Assertion Error in Python
Try running the below code.
try:
assert 123 == 256432
except AssertionError:
print("There is some problem!")
Output:
There is some problem!
Here, the assert
statement checks if the two numbers are equal. Since these numbers are not equal, the AssertionError
exception is raised from the try
block.
The except
block catches the exception and executes the print statement. Here, we get the output present inside the print statement in the exception block.
To know where the source of the exception is, we can use the raise
keyword to reraise the exception in the except
block.
The raise
keyword will raise an error in case of an exception and stop the program. It helps track the current exception.
The syntax of the raise
statement is as follows.
raise {exception class}
The exception can be a built-in exception, or we can create a custom exception. We can also print some messages using the raise
keyword and create a custom exception.
raise Exception("print some string")
This example shows the working of the raise
keyword.
try:
assert 1 == 2
except AssertionError:
print("There is some problem!")
raise
Output:
There is some problem!
Traceback (most recent call last):
File "Desktop/Tut.py", line 2, in <module>
assert 1 == 2
AssertionError
We have reraised the exception in the except
block in the code above. You can observe how using the raise
keyword gives the exception source in line 2
.
This way, we can get the line number of exceptions and the exact error raising part of the code.
Use the Logging Module With Try-Except
Blocks to Handle the Assertion Error in Python
The logging
module in Python helps you keep track of the execution and errors of an application. This module keeps track of the events that take place during any operation.
It is helpful in case of crashes since we can find out the previous data from the logs. Thus, we can look back and figure out what caused the error in case of any problem.
We can import the logging
module and use the logging.error
method inside the except
block.
import logging
try:
assert True == False
except AssertionError:
logging.error("Something is quite not right!", exc_info=True)
Output:
ERROR:root:Something is quite not right!
Traceback (most recent call last):
File "Desktop/Tut.py", line 4, in <module>
assert True == False
AssertionError
This method also returns the line number and the exact source of the exception.
This module has many objects for different kinds of error messages. These objects log the messages with levels on the logger.
For example, the Logger.critical(message)
logs the message with the critical
level. The Logger.error(message)
logs the message with the level error
in the code above.
Use the Traceback Module to Handle the Assertion Error in Python
The traceback
module helps catch the exact source of error when the code has multiple assert statements.
import sys
import traceback
try:
assert 88 == 88
assert 1 == 101
assert True
except AssertionError:
_, _, var = sys.exc_info()
traceback.print_tb(var)
tb_info = traceback.extract_tb(var)
filename, line_number, function_name, text = tb_info[-1]
print(
"There is some error in line {} in this statement: {}".format(line_number, text)
)
exit(1)
Output:
File "Desktop/Tut.py", line 6, in <module>
assert 1 == 101
There is some error in line 6 in this statement: assert 1 == 101
Using the traceback
module, we can write our print
statement with placeholders, {}
.
Further, we can specify different variables for holding the file’s name, the line number, the name of the function, and the text where the exception occurs.
Here, tb
refers to the traceback object. We use only two placeholders in the print statement, one for the line number and the other for the text.
The sys.exc_info()
function returns the three parts of the raise statement - exc_type
, exc_traceback
, and exc_value
. Let us put another placeholder inside the print statement for the filename.
import sys
import traceback
try:
assert 88 == 88
assert 1 == 101
assert True
except AssertionError:
_, _, var = sys.exc_info()
traceback.print_tb(var)
tb_info = traceback.extract_tb(var)
filename, line_number, function_name, text = tb_info[-1]
print(
"There is some error in the file {} on line {} in this statement: {}".format(
filename, line_number, text
)
)
exit(1)
Output:
File "Desktop/Tut.py", line 6, in <module>
assert 1 == 101
There is some error in the file Desktop/Tut.py on line 6 in this statement: assert 1 == 101
This time we also get the complete URL of the file as the filename.
Refer to this documentation for more details on the traceback module.
Use a print
Statement to Handle the AssertionError
Exception Manually in Python
We can use a print
statement inside the except
block to handle an exception manually.
try:
assert True == False, "Operation is invalid"
print(True)
except AssertionError as warn:
print(warn)
Output:
Operation is invalid
Whatever error message the user provides goes into the print
statement and gets printed. This way, the user does not have to bother about the technical error.
A simple message is shown instead of an error.
Conclusion
This article showed how we could handle the AssertionError
in Python. We discussed using the raise keyword, the logging module, and the traceback module to work through assertion errors.
We also saw how to manually handle an AssertionError
exception using a simple print statement. In a real-world application, AssertionError
is not used.
It would help if you only used it while developing and testing programs.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python