How to Get Filename From Path in Python
-
Use the
os.path.split()
Function to Get Filename From Path in Python -
Use the
os.path.basename()
Function to Get Filename From Path in Python -
Use the
ntpath
Library to Get Filename From Path in Python -
Use the
pathlib
Module to Get Filename From Path in Python - Conclusion
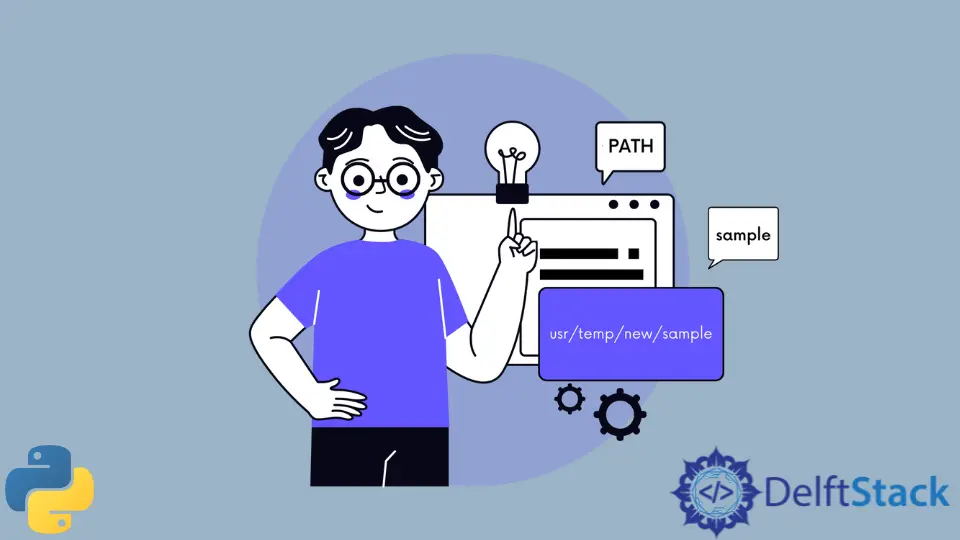
In programming, we often need to extract the filename from a given file path. Whether we’re seasoned developers or just beginning our programming journey, understanding how to retrieve the filename from a path in Python is an essential skill.
Consider a scenario where we have a file path, such as C:\Documents\Project\file.txt
, and we need to extract just the filename, i.e., file.txt
. This seemingly simple task can pose a challenge, especially when dealing with complex paths or different operating systems.
Extracting filenames from file paths is vital in many programming applications. Whether working on file management systems, data analysis, or web development, understanding how to manipulate file paths efficiently can enhance our productivity and enable us to build robust, flexible programs.
Additionally, this knowledge helps us handle edge cases, such as duplicate filenames, file extensions, or nested directory structures, ensuring our code operates reliably in real-world scenarios. Python offers various techniques and libraries to tackle this problem efficiently, allowing us to focus on the more significant aspects of our code.
This tutorial introduces how to get a file name from the path in Python. It also lists some example codes to demonstrate further the concepts associated with specific operating systems.
Use the os.path.split()
Function to Get Filename From Path in Python
The os.path.split()
function in Python is a powerful tool that allows us to extract the filename from a given file path effortlessly. This function is part of the os.path
module, which provides various path manipulation functionalities.
By utilizing os.path.split()
, we can conveniently split a file path into its directory and filename components and then retrieve just the filename portion.
Syntax:
import os
directory, filename = os.path.split(path)
Parameters: The os.path.split()
function accepts a single parameter.
path
: This parameter represents the file path from which we want to extract the filename. It can be a string containing the absolute or relative path of the file.
Return Values: The function returns a tuple containing two elements.
-
directory
: This variable holds the directory component of the path, excluding the filename. -
filename
: This variable contains the filename extracted from the path.
The os.path.split()
function effectively separates a file path’s directory and filename parts, providing us with the necessary tools to work specifically with the filename component. The following code snippet demonstrates how we can get a file name from a path in Python using the os.path.split()
function.
import os
directory, filename = os.path.split("MyFolder/MySubFolder/MyFile.txt")
print(directory)
print(filename)
Output:
The above code uses Python’s os.path.split()
function to extract the directory and filename components from a file path.
First, the os.path.split()
function is imported from the os
module. The function is then applied to the file path MyFolder/MySubFolder/MyFile.txt
.
The result is assigned to the directory
and filename
variables.
The directory
variable will hold the path MyFolder/MySubFolder
, which represents the directory component of the file path. The filename
variable will store the string MyFile.txt
, which is the extracted filename.
Finally, the directory and filename are printed separately using the print()
function. This allows us to see the extracted directory and filename components of the given file path.
Use the os.path.basename()
Function to Get Filename From Path in Python
The os.path.basename()
function in Python is a handy tool for extracting the filename component from a given file path. It is part of the os.path
module and allows us to retrieve the last component of a path, which is typically the filename.
Syntax:
import os
filename = os.path.basename(path)
Parameters: The os.path.basename()
function accepts a single parameter.
path
: This parameter represents the file path from which we want to extract the filename. It can be a string containing the absolute or relative path of the file.
Return Value: The function returns a string representing the extracted filename from the given path.
While both os.path.basename()
and os.path.split()
can extract filenames from paths, they differ in their approach and the information they provide. The os.path.basename()
function focuses solely on the filename component and returns it as a string.
It is a more straightforward function, ideal for only the filename and not the directory part. The following code snippet demonstrates how we can get a file name from a path in Python using the os.path.split()
function.
import os
filename = os.path.basename("MyFolder/MySubFolder/MyFile.txt")
print(filename)
Output:
The above code demonstrates the usage of the os.path.basename()
function in Python to extract the filename from a file path.
First, the os.path.basename()
function is imported from the os
module. Then, the function is applied to the file path MyFolder\MySubFolder\MyFile.txt
.
The result, which is the filename MyFile.txt
, is assigned to the variable filename
and printed.
Use the ntpath
Library to Get Filename From Path in Python
Python’s ntpath
library provides path manipulation functionalities specifically for Windows paths. It includes the ntpath.basename()
function, which allows us to extract the filename component from a given file path.
This function operates similarly to os.path.basename()
but is designed specifically for Windows paths.
Syntax:
import ntpath
filename = ntpath.basename(path)
Parameters: The ntpath.basename()
function accepts a single parameter.
path
: This parameter represents the file path from which we want to extract the filename. It can be a string containing the absolute or relative path of the file.
Return Value: The function returns a string representing the extracted filename from the given path.
Compared to the previous methods, the ntpath.basename()
and os.path.basename()
functions serve the same purpose: retrieving the filename component from a path. However, ntpath.basename()
is specific to Windows paths, while os.path.basename()
is cross-platform and can handle paths from any operating system.
The following code snippet demonstrates how we can get a file name from a path in Python using the ntpath.basename()
function.
import ntpath
filename = ntpath.basename("MyFolder/MySubFolder/MyFile.txt")
print(filename)
Output:
The above code demonstrates using the ntpath.basename()
function from the ntpath
library in Python to extract the filename from a file path, specifically for Windows paths.
First, the ntpath.basename()
function is imported from the ntpath
library. Then, the function is applied to the file path MyFolder/MySubFolder/MyFile.txt
.
The result, which is the filename MyFile.txt
, is assigned to the variable filename
and printed.
Use the pathlib
Module to Get Filename From Path in Python
The pathlib
module in Python provides an object-oriented approach to working with file paths. It offers the Path
class, which encapsulates path-related operations and allows us to extract the filename from a given file path easily.
The pathlib.Path
class allows us to navigate directories, manipulate file paths, and retrieve specific components such as filenames.
The Path
class does not require any specific parameters. It takes the file path as a string argument when creating an instance of the Path
class.
While the os.path.basename()
function can also extract the filename from a path, the pathlib
module provides a more modern and convenient approach. With pathlib.Path
, we can directly access the filename using the .name
attribute of a Path
object, simplifying the code.
The following code snippet demonstrates how we can get a file name from a path in Python using the pathlib
module.
from pathlib import Path
filename = Path("MyFolder/MySubFolder/MyFile.txt").name
print(filename)
Output:
The above code demonstrates the usage of the pathlib
module in Python to extract the filename from a file path.
First, the pathlib
module is imported, specifically the Path
class. Then, the Path
class creates a Path
object representing the file path MyFolder/MySubFolder/MyFile.txt
.
The .name
attribute is applied to the Path
object, which directly retrieves the filename component from the path. The extracted filename is then assigned to the variable filename
and printed.
Conclusion
We have explored 4 different methods in Python to extract the filename from a file path: os.path.split()
, os.path.basename()
, ntpath.basename()
, and pathlib.Path.name
. Each method has advantages and disadvantages, making them suitable for different scenarios.
The os.path.split()
method can split a path into its directory and filename components. This granularity allows for more control over the path but requires additional code to access just the filename. However, it works across different operating systems, making it versatile.
On the other hand, os.path.basename()
offers a simpler approach by directly extracting the filename from the path. It is compatible with various platforms and provides a straightforward solution. However, it only returns the filename as a string.
For Windows-specific paths, ntpath.basename()
is designed to handle these paths. It directly extracts the filename without the need for additional code. However, it is limited to Windows paths and lacks cross-platform compatibility.
Lastly, pathlib.Path.name
provides an object-oriented approach for working with paths. It allows further path manipulation and integrates well with other pathlib
functionalities. However, it introduces some complexity for simple filename extraction and requires importing the pathlib
module.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn