Python os.path.basename() Method
-
Syntax of Python
os.path.basename()
Method -
Example 1: Use the
os.path.basename()
Method in Python -
Example 2: Extract a File Name Using the
os.path.basename()
Method -
Example 3: Use
os.path.dirname()
With theos.path.basename()
Method -
Example 4: Set Path Address as Empty in the
os.path.basename()
Method
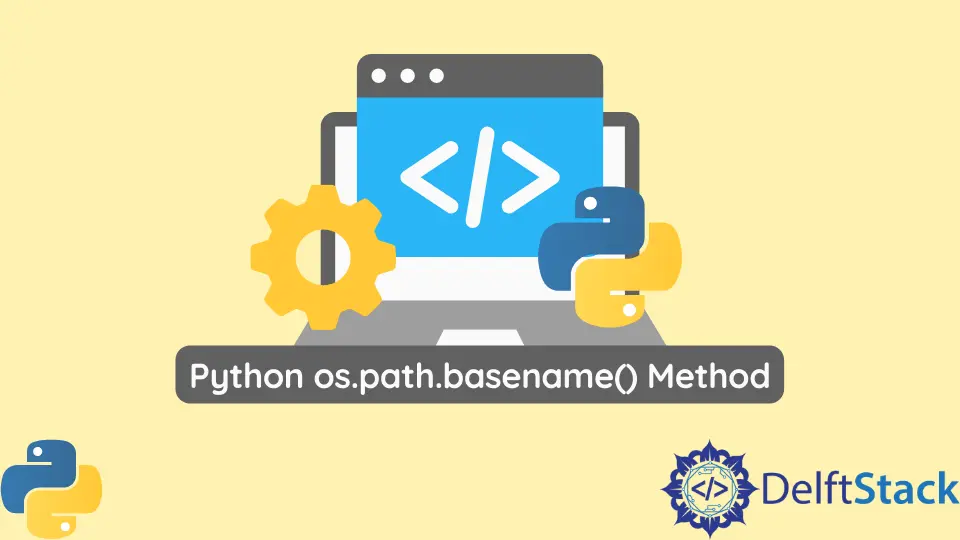
Python os.path.basename()
method is the most efficient way of finding the basename of a specified path. This method uses the os.path.split()
method to first split the path address into a pair of head
and tail
, then display the tail
part.
Syntax of Python os.path.basename()
Method
os.path.basename(path)
Parameters
path |
an address object of a file system path. The object can either be an str or bytes. |
Return
This method’s return type is a path string containing the file’s absolute address.
Example 1: Use the os.path.basename()
Method in Python
import os
path = "/home///user/Documents"
normalize = os.path.basename(path)
print(normalize)
path = "/home/./Desktop"
normalize = os.path.basename(path)
print(normalize)
Output:
Documents
Desktop
The os.path.basename()
is a simple method to interact with the operating system and retrieve the base name of any specific file.
Example 2: Extract a File Name Using the os.path.basename()
Method
import os
path = "/home/user/temp/../Downloads/File.txt"
normalize = os.path.basename(path)
print(normalize)
Output:
File.txt
Note that the os.path.basename
result is not similar to the value returned from the Unix basename program.
Example 3: Use os.path.dirname()
With the os.path.basename()
Method
import os
path = "home/user/File.txt"
head = os.path.dirname(path)
tail = os.path.basename(path)
rejoin = head + tail
print("The head of the path '%s': " % path, head)
print("The tail of the path '%s': " % path, tail)
print("The full path address is: ", rejoin)
Output:
The head of the path 'home/user/File.txt': home/user
The tail of the path 'home/user/File.txt': File.txt
The full path address is: home/userFile.txt
If we use the os.path.split()
method to split the path, then the head part is the same value returned by the os.path.dirname
.
Example 4: Set Path Address as Empty in the os.path.basename()
Method
import os
address = ""
directory = os.path.basename(address)
print(directory)
Output:
The os.path.basename()
doesn’t return anything as no path address is specified.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn