How to Find NTH Root of X Value in Python
-
Find the NTH Root of X Value in Python Using NumPy
power()
- Find the NTH Root of X Value in Python Using the Power Operator
-
Find the NTH Root of X Value in Python Using
math.pow()
-
Find the NTH Root of X Value in Python Using
math.exp()
andmath.log()
- Conclusion
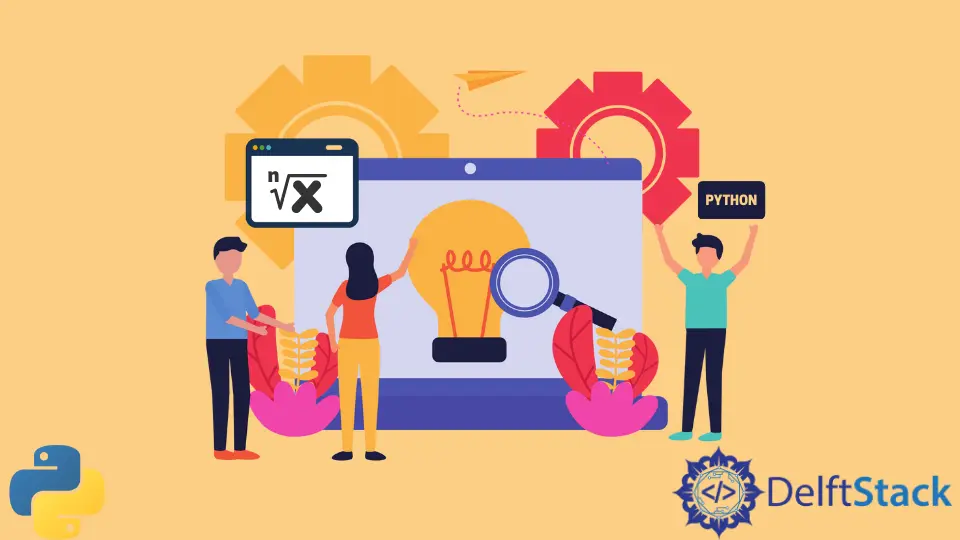
The nth root of a number x refers to finding a value y that, when raised to the power of n, results in x. In mathematical terms, for a given positive integer n, the nth root of x is denoted as y = x^(1/n)
.
Understanding and calculating nth roots are fundamental concepts in mathematics, engineering, computer science, and various scientific disciplines. It’s essential for solving equations, analyzing data, and conducting a wide range of computations.
Python, being a versatile programming language, provides several methods and libraries to efficiently calculate the nth root of a given value x. The cube root (3rd root) of x can easily be found using the numpy.cbrt()
function, and similarly, the square root (2nd root) of (x) can easily be determined using the numpy.sqrt()
built-in function.
In this article, we will explore the methods to calculate the nth root of x in Python using the exponentiation operator, the math
module, and the numpy
library.
Find the NTH Root of X Value in Python Using NumPy power()
NumPy is a powerful library in Python for numerical computing. The power()
function in NumPy is used to raise the elements of an array to a specified power, but it can also be used for a single value.
The syntax is as follows:
result = np.power(base, exponent)
Here, base
is the number you want to raise to a power, and exponent
is the power to which you want to raise the base.
To find the nth root of a number x, we can use the power()
function from NumPy by raising x to the power of (1 / n). The formula for finding the nth root is as follows:
nth_root = np.power(x, 1 / n)
Here, x
is the value for which we want to find the nth root, and n
is the degree of the root.
Let’s illustrate this with an example:
import numpy as np
def find_nth_root(x, n):
nth_root = np.power(x, 1 / n)
return nth_root
x = 16
n = 4
result = find_nth_root(x, n)
print(f"The {n}th root of {x} is: {result}")
Output:
The 4th root of 16 is: 2.0
Here, we begin by importing the NumPy library using import numpy as np
. Next, we defined a function find_nth_root
, which takes two arguments: x
representing the number for which we want to find the nth root, and n
indicating the degree of the root we seek.
Within the function, we used the NumPy power()
function, taking x
and 1 / n
as arguments. This calculates the nth root of x
by raising x
to the power of 1 / n
.
The result is assigned to the nth_root
variable and then returned.
For the example usage, x
is assigned the value 16, representing the number for which we want to calculate the nth root. n
is set to 4, indicating that we’re aiming to find the 4th root of 16.
The find_nth_root
function is invoked with these parameters, and the calculated nth root is stored in the variable result
.
Lastly, we used a formatted string to present the result.
Find the NTH Root of X Value in Python Using the Power Operator
The power operator in Python is denoted by **
. It raises a number to a specified power.
The syntax is as follows:
result = base ** exponent
Here, base
is the number you want to raise to a power, and exponent
is the power to which you want to raise the base.
To find the nth root of a number x, we can use the power operator by raising x to the power of (1 / n). The formula for finding the nth root is as follows:
nth_root = x ** (1 / n)
Here, x
is the value for which we want to find the nth root, and n
is the degree of the root.
Let’s illustrate this with an example:
def find_nth_root(x, n):
nth_root = x ** (1 / n)
return nth_root
x = 16
n = 4
result = find_nth_root(x, n)
print(f"The {n}th root of {x} is: {result}")
Output:
The 4th root of 16 is: 2.0
The provided code defines a Python function find_nth_root(x, n)
that calculates the nth root of a given number x
using the power operator. Inside the function, the nth root is computed by raising x
to the power of 1/n
, effectively finding the nth root.
The code demonstrates an example usage, where x
is set to 16 and n
is set to 4, indicating that we want to find the 4th root of 16. The find_nth_root
function is then invoked with these parameters, and the calculated nth root is stored in the variable result
.
Finally, a formatted string is used to display The 4th root of 16 is: 2.0
.
Find the NTH Root of X Value in Python Using math.pow()
The math.pow()
function in Python is part of the math
module. It takes two arguments, the base and the exponent, and returns the result of raising the base to the power of the exponent.
The syntax is as follows:
result = math.pow(base, exponent)
Here, base
is the number you want to raise to a power, and exponent
is the power to which you want to raise the base.
To find the nth root of a number x, we can use the math.pow()
function by raising x to the power of (1 / n). The formula is as follows:
nth_root = math.pow(x, 1 / n)
Here, x
is the value for which we want to find the nth root, and n
is the degree of the root.
Let’s illustrate this with an example:
import math
def find_nth_root(x, n):
nth_root = math.pow(x, 1 / n)
return nth_root
x = 16
n = 4
result = find_nth_root(x, n)
print(f"The {n}th root of {x} is: {result}")
Output:
The 4th root of 16 is: 2.0
The provided code begins by importing the math
module, which contains math.pow()
that we utilized to calculate the nth root. The find_nth_root
function is defined, taking two arguments: x
(the number for which we want to find the nth root) and n
(the degree of the root we’re seeking).
Inside this function, math.pow(x, 1 / n)
is employed to calculate the nth root of x
by raising x
to the power of 1 / n
. This value is assigned to nth_root
, which is then returned.
In the example usage, x
is set to 16, representing the number for which we want to calculate the nth root. n
is set to 4, indicating that we’re looking for the 4th root of 16.
The find_nth_root
function is called with these parameters, and the calculated nth root is stored in the variable result
.
Finally, a formatted string is used to display the result, showcasing the 4th root of 16.
Find the NTH Root of X Value in Python Using math.exp()
and math.log()
The formula for finding the nth root of a number x can also be represented in terms of the exponential and logarithmic functions as:
nth root value = exp(log(x) / n)
Here, exp
is the exponential function, and log
is the natural logarithm function.
In Python, the math
module provides the necessary functions exp()
and log()
to implement this formula and calculate the nth root of a value x
.
Let’s go through an example code and understand how to use this formula effectively.
import math
def nth_root(x, n):
nth_root_value = math.exp(math.log(x) / n)
return nth_root_value
x = 64
n = 3
nth_root_value = nth_root(x, n)
print(f"The cube root of {x} is: {nth_root_value}")
Output:
The cube root of 64 is: 4.0
Here, we begin by importing the math
module, which provides the exp()
and log()
functions needed for our calculation.
Then, we define a function nth_root
that takes two parameters, x
(the value for which we want to find the nth root) and n
(the desired root). Inside the function, we calculate the nth root using the formula exp(log(x) / n)
and return the result.
As an example, we set the value of x
to 64 and the desired root n
to 3 (e.g., cube root). We then call the nth_root
function with these values and store the result in nth_root_value
.
Finally, we print the result, showing the nth root of x
(here, the cube root of 64).
Conclusion
Calculating the nth root of a number is a common operation in various mathematical and scientific applications. In Python, we have multiple ways to achieve this, including using the exponentiation operator, the math
module, or the numpy
library.
Depending on the requirements and the nature of the project, you can choose the most suitable method for finding the nth root of x
in Python.
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn