How to Find Median of a List in Python
- Using Python’s Built-in Functions
- Using NumPy for Median Calculation
- Custom Function to Calculate Median
- Handling Edge Cases
- Conclusion
- FAQ
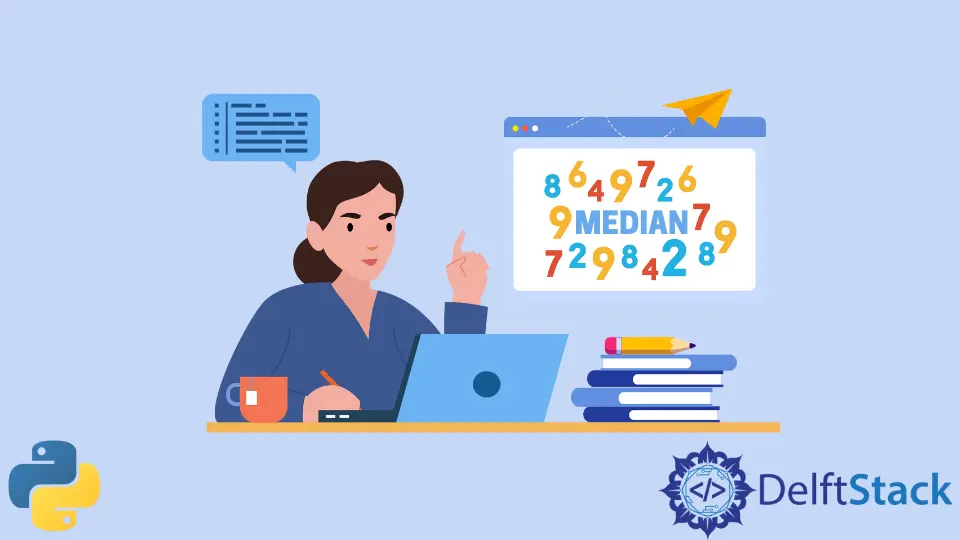
Calculating the median of a list in Python is a fundamental task that can come in handy in various data analysis scenarios. The median, which represents the middle value of a dataset when arranged in order, is crucial for understanding central tendencies without being skewed by outliers.
This tutorial will guide you through different methods to calculate the median of a list in Python, ensuring you have a solid grasp of how to implement these techniques effectively. We will explore built-in functions, custom functions, and libraries that simplify the process. Whether you’re a beginner or an experienced programmer, this guide will enhance your Python skills and improve your data manipulation capabilities.
Using Python’s Built-in Functions
Python provides a straightforward way to calculate the median using its built-in libraries. The most commonly used library for this purpose is the statistics
module, which contains a median
function specifically designed to perform this calculation. Here’s how you can use it:
import statistics
data = [3, 1, 4, 2, 5]
median_value = statistics.median(data)
print(median_value)
Output:
3
In this example, we first import the statistics
module, which comes pre-installed with Python. We then define a list called data
containing some integers. The median
function from the statistics
module calculates the median of the list. Finally, we print the median value. This method is efficient and easy to read, making it a go-to choice for many developers.
Using NumPy for Median Calculation
If you’re working with larger datasets or performing more complex calculations, the NumPy library is an excellent choice. NumPy is widely used in data science and provides optimized functions for numerical operations, including median calculation. Here’s how you can use NumPy to find the median:
import numpy as np
data = [3, 1, 4, 2, 5]
median_value = np.median(data)
print(median_value)
Output:
3.0
Here, we import the NumPy library and define our list of numbers. The np.median()
function computes the median. Unlike the statistics
module, NumPy returns a float value, which is particularly useful when dealing with non-integer datasets. This method is not only efficient but also scales well with larger arrays, making it ideal for data analysis tasks.
Custom Function to Calculate Median
While using built-in libraries is convenient, understanding how to implement your own median function can deepen your knowledge of Python. Creating a custom function involves sorting the list and finding the middle value. Here’s a simple implementation:
def calculate_median(data):
sorted_data = sorted(data)
n = len(sorted_data)
mid = n // 2
if n % 2 == 0:
return (sorted_data[mid - 1] + sorted_data[mid]) / 2
else:
return sorted_data[mid]
data = [3, 1, 4, 2, 5]
median_value = calculate_median(data)
print(median_value)
Output:
3
In this custom function, calculate_median
, we first sort the input list. The length of the list is stored in n
, and the middle index is calculated. If the length of the list is even, the function returns the average of the two middle values. If it’s odd, it simply returns the middle value. This method enhances your understanding of how median calculation works and can be easily modified for different data types or structures.
Handling Edge Cases
When calculating the median, it’s essential to consider edge cases, such as empty lists or lists with a single element. Here’s how you can modify our custom function to handle these scenarios:
def calculate_median(data):
if not data:
return None
sorted_data = sorted(data)
n = len(sorted_data)
mid = n // 2
if n % 2 == 0:
return (sorted_data[mid - 1] + sorted_data[mid]) / 2
else:
return sorted_data[mid]
data_empty = []
data_single = [5]
median_empty = calculate_median(data_empty)
median_single = calculate_median(data_single)
print(median_empty)
print(median_single)
Output:
None
5
In this modified version of the calculate_median
function, we first check if the input list is empty. If it is, the function returns None
. This prevents errors when attempting to sort or access elements in an empty list. For a single-element list, the function correctly returns that element as the median. This approach ensures that your function is robust and can handle various input scenarios gracefully.
Conclusion
Calculating the median of a list in Python can be accomplished in multiple ways, from using built-in libraries like statistics
and NumPy
to creating custom functions. Each method has its advantages, whether it be simplicity, efficiency, or the ability to handle complex datasets. Understanding these techniques not only enhances your programming skills but also prepares you for more advanced data analysis tasks. So, whether you’re analyzing data for a project or just honing your Python skills, knowing how to find the median is a valuable tool in your programming toolkit.
FAQ
-
What is the median in statistics?
The median is the middle value of a dataset when it is ordered from least to greatest. If the dataset has an even number of values, the median is the average of the two middle numbers. -
Can I calculate the median of a list with non-numeric values?
No, the median calculation requires numeric values. If you have non-numeric values, you’ll need to filter or convert them before calculating the median. -
What happens if the list is empty?
If the list is empty, the median function should returnNone
or raise an error, depending on how it’s implemented. -
Is NumPy better than the statistics module for calculating the median?
NumPy is generally faster and more efficient for larger datasets, while the statistics module is simpler and sufficient for smaller lists. -
Can I use the median function on a list of strings?
No, the median function is designed for numeric data. You need to convert the strings to numbers before calculating the median.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn