在 Python 中查詢列表的中位數
Manav Narula
2023年1月30日
-
在 Python 中使用
statistics
模組查詢列表的中位數 -
在 Python 中使用
numpy.percentile
函式尋找一個列表的中位數 - 在 Python 中使用自定義程式碼查詢一個列表的中位數
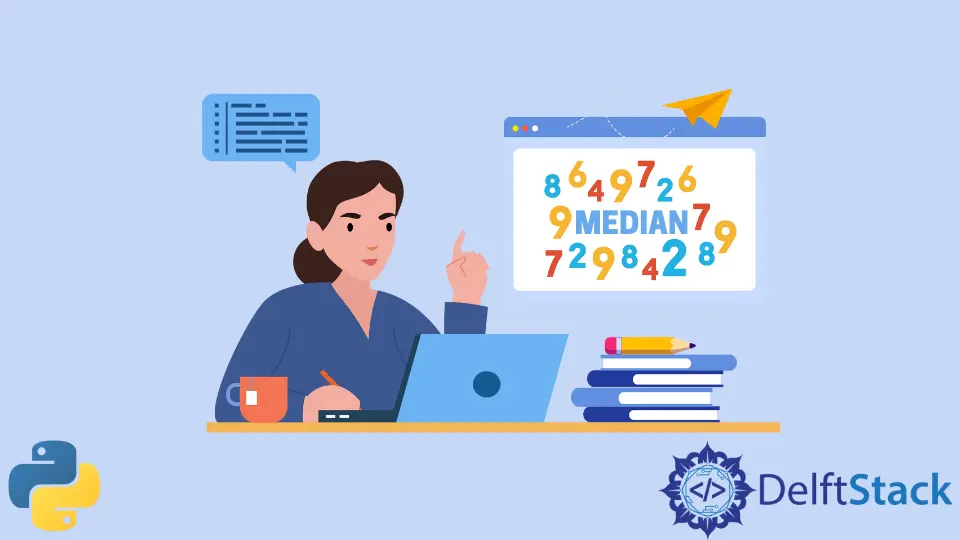
在統計學和概率的世界裡,一組給定觀測值的中值是中間元素。當元素總數是奇數和偶數時,它的計算方法是不同的。
Python 經常被用於資料和統計分析。本教程將介紹如何用 Python 計算列表的中位數。
在 Python 中使用 statistics
模組查詢列表的中位數
在 Python 中,我們有 statistics
模組,它有不同的函式和類來從一組資料中找到不同的統計值。這個庫中的 median()
函式可以用來尋找一個列表的中位數。
由於中位數基於排序的資料列表上的,所以 median()
函式會自動對其進行排序,並返回中位數。例如
import statistics
lst = [7, 8, 9, 5, 1, 2, 2, 3, 4, 5]
print(statistics.median(lst))
輸出:
4.5
在 Python 中使用 numpy.percentile
函式尋找一個列表的中位數
在 NumPy
模組中,我們有一些函式可以從一個陣列中找到百分位值。資料的中位數是第 50 個百分位值。為了找到這個值,我們可以使用 NumPy
模組中的 percentile()
函式,計算出第 50 個百分位值。請看下面的程式碼。
import numpy as np
a = np.array([7, 8, 9, 5, 1, 2, 2, 3, 4, 5])
median_value = np.percentile(a, 50)
print(median_value)
輸出:
4.5
在 Python 中使用自定義程式碼查詢一個列表的中位數
我們還可以使用 Python 公式來查詢資料的中位數,並建立使用者定義的函式。例如,
lst = [7, 8, 9, 5, 1, 2, 2, 3, 4, 5]
def median(l):
half = len(l) // 2
l.sort()
if not len(l) % 2:
return (l[half - 1] + l[half]) / 2.0
return l[half]
print(median(lst))
輸出:
4.5
如果列表的長度是奇數,則列表的中位數是排序列表的中間元素;否則,它是兩個中間元素的平均值。
作者: Manav Narula
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn