How to Check if Set Is Empty in Python
-
Use the
len()
Function to Check if Set Is Empty in Python -
Use the
bool()
Function to Check if Set Is Empty in Python -
Use the
set()
Method to Check if Set Is Empty in Python -
Use the
not
Operator to Check if Set Is Empty in Python - Conclusion
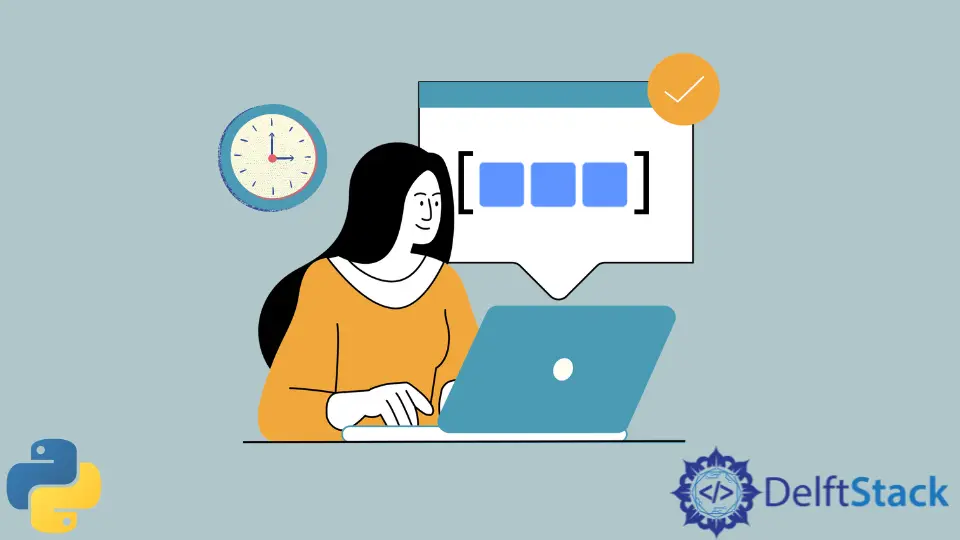
Sets are unordered collections of unique elements in Python that provide efficient membership checking and eliminate duplicates. At times, we may encounter situations where we must determine whether a set is empty.
The ability to check if a set is empty is important in various programming scenarios as it enables us to make informed decisions and avoid program errors. It allows us to efficiently handle situations where we need to determine if a set contains any elements and perform appropriate actions based on that.
Consider a scenario where we are developing a website that will allow users to create and manage their task lists. We can use the set empty check to ensure that a user’s task list is not empty before displaying it.
If the set representing the user’s tasks is empty, we can display a message like No tasks found
or provide alternative content to guide the user in creating new tasks.
This tutorial will discuss various methods to check if a set is empty in Python.
Use the len()
Function to Check if Set Is Empty in Python
One effective approach to determine whether a set is empty is by utilizing the len()
function, which returns the number of elements in a set.
Basic Syntax:
len(my_set)
Parameter:
iterable(my_set)
: The iterable (e.g., set, list, string, etc.) for which you want to find the length. It is the object you want to measure.
Using the len()
function, we can conveniently check the size of a set and ascertain whether it is empty or contains elements. This technique provides a straightforward and concise solution for checking whether a set is empty in Python, enabling us to handle different scenarios and make informed decisions in our programs.
To check if a set is empty, we can check whether its length is zero. The example code below demonstrates how to check if a set is empty using Python’s len()
function.
def is_empty(a):
return len(a) == 0
a = set("a")
b = set()
print(is_empty(a))
print(is_empty(b))
In this code, we define a function is_empty(a)
that takes a single argument a
. This function checks if the length of the set a
is equal to 0
and returns True
if it is empty and False
if it’s not.
We then create two sets, a
and b
. Set a
contains the single element "a"
, while set b
is empty.
When we call the is_empty
function with a
and b
as arguments and print the results, here’s what happens:
-
is_empty(a)
checks the seta
and finds that it’s not empty because it contains one element ("a"
). Therefore, it returnsFalse
, and we printFalse
. -
is_empty(b)
checks the setb
, which is empty, with a length of0
. It returnsTrue
because the set is empty, and we printTrue
.
Output:
False
True
In the output, the first call to is_empty(a)
returns False
because the set a
is not empty. The second call to is_empty(b)
returns True
because the set b
is empty.
Use the bool()
Function to Check if Set Is Empty in Python
Another efficient way to check if a set is empty in Python is by utilizing the bool()
function. The bool()
function can evaluate an object’s truthiness, and it returns a Boolean value.
Basic Syntax:
bool(expression)
Parameter:
expression
: This is the value or expression that you want to evaluate as a Boolean. The function returnsTrue
if the expression is considered “truthy” andFalse
if it is considered “falsy”.
When applied to a set, the bool()
function will return False
if the set is not empty (contains elements) and True
if the set is empty. This approach provides a straightforward and intuitive solution for checking the emptiness of a set in Python, allowing us to perform conditional checks.
Compared to the previously discussed len()
method, the bool()
function offers a more direct and intuitive way of checking emptiness. It avoids the need to compare the set’s length with zero explicitly.
However, it’s important to note that both methods achieve the same result, so their choice depends on personal preference and coding style. The example code below demonstrates how to check if a set is empty using Python’s bool()
function.
def is_empty(a):
return not bool(a)
a = set("a")
b = set()
print(is_empty(a))
print(is_empty(b))
In this code, we define a function is_empty(a)
that takes a single argument a
.
This function checks if the set a
is empty by converting it to a Boolean value and negating it. It returns True
if the set is empty and False
if it’s not.
We then create two sets, a
and b
. Set a
contains the single element "a"
, while set b
is empty.
When we call the is_empty
function with a
and b
as arguments and print the results, here’s what happens:
-
is_empty(a)
checks the seta
and finds that it’s not empty because it contains one element ("a"
). It returnsFalse
after negating, and we printFalse
. -
is_empty(b)
checks the setb
, which is empty, and returnsTrue
after negating because the set is empty. We printTrue
.
Output:
False
True
In the output, the first call to is_empty(a)
returns False
because the set a
is not empty. The second call to is_empty(b)
returns True
because the set b
is empty.
Use the set()
Method to Check if Set Is Empty in Python
The set()
method initializes an empty set. So if the given set is equal to set()
, it is empty.
Basic Syntax:
my_set = set(iterable)
Parameter:
my_set
: This is the variable that will hold the resulting set.iterable
: The iterable (e.g., a list, tuple, string, etc.) that you want to convert into a set.
Unlike the len()
method, which requires explicit comparison to zero, and the bool()
method, which involves negating the Boolean result, the set()
method directly converts the set, simplifying the code and making it more readable. It provides a straightforward and elegant solution for checking the emptiness of a set in Python.
The example code below demonstrates how to check if a set is empty using Python’s set()
method.
def is_empty(a):
return a == set()
a = set("a")
b = set()
print(is_empty(a))
print(is_empty(b))
In this code, we define a function is_empty(a)
that takes a single argument a
.
This function checks if the set a
is equal to an empty set (i.e., set()
). It returns True
if the set is empty and False
if it’s not.
We then create two sets, a
and b
. Set a
contains the single element "a"
, while set b
is empty.
When we call the is_empty
function with a
and b
as arguments and print the results, here’s what happens:
-
is_empty(a)
checks the seta
and finds that it’s not empty because it contains one element ("a"
). It returnsFalse
, and we printFalse
. -
is_empty(b)
checks the setb
, which is empty, and returnsTrue
because the set is equal to an empty set. We printTrue
.
Output:
False
True
In the output, the first call to is_empty(a)
returns False
because the set a
is not empty. The second call to is_empty(b)
returns True
because the set b
is empty, which is equivalent to an empty set.
Use the not
Operator to Check if Set Is Empty in Python
In Python, the not
operator provides a concise and intuitive approach to checking if a set is empty. Applying the not
operator to a set evaluates to True
if the set is empty and False
if it contains any elements.
This method offers a direct way to determine the emptiness of a set without requiring additional function calls or comparisons.
When comparing the not
operator to the previously discussed len()
, bool()
, and set()
methods, it stands out as a simple and straightforward solution. It avoids the need for explicit length checks, function invocations, or set conversions.
The not
operator provides a concise and elegant way to check the emptiness of a set in Python. The example code below demonstrates how to check if a set is empty using Python’s not
operator.
def is_empty(a):
return not a
a = set("a")
b = set()
print(is_empty(a))
print(is_empty(b))
In this code, we define a function is_empty(a)
that takes a single argument a
.
This function checks if the set a
is empty by directly evaluating it as a Boolean expression. It returns True
if the set is empty (falsy) and False
if it’s not.
We then create two sets, a
and b
. Set a
contains the single element "a"
, while set b
is empty.
When we call the is_empty
function with a
and b
as arguments and print the results, here’s what happens:
is_empty(a)
checks the seta
and finds that it’s not empty because it contains one element ("a"
). It returnsFalse
after negating the Boolean expression, and we printFalse
.
is_empty(b)
checks the setb
, which is empty, and returnsTrue
after negating the Boolean expression because the set is empty. We printTrue
.
Output:
False
True
In the output, the first call to is_empty(a)
returns False
because the set a
is not empty. The second call to is_empty(b)
returns True
because the set b
is empty.
Conclusion
In conclusion, we have explored four different methods to check if a set is empty in Python: len()
, bool()
, set()
, and the not
operator. Each method has its advantages and disadvantages, and the task’s specific requirements determine the method chosen.
The len()
method offers a straightforward approach but requires an explicit comparison to zero. It is suitable when there is a need to know the exact size of the set and check for emptiness.
The bool()
method provides a concise way to check emptiness directly, but it involves negating the Boolean result. It is suitable when the sole focus is determining emptiness without needing the set’s size.
The set()
method simplifies the code by comparing it to an empty set, but it may create an unnecessary duplicate set object. It is suitable when readability and simplicity are the primary concerns.
The not
operator offers a concise and intuitive approach but directly converts the set to its Boolean equivalent. It is suitable when emphasizing a concise and direct check for emptiness.