How to Check for NaN Values in Python
-
Use the
math.isnan()
Function to Check fornan
Values in Python -
Use the
numpy.isnan()
Function to Check fornan
Values in Python -
Use the
pandas.isna()
Function to Check fornan
Values in Python -
Use the
obj != obj
to Check fornan
Values in Python
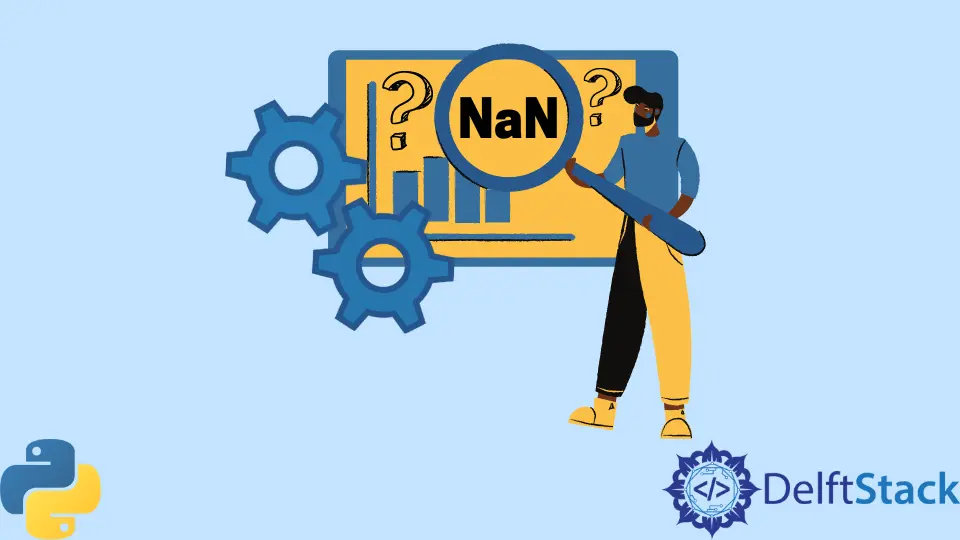
The nan
is a constant that indicates that the given value is not legal - Not a Number
.
Note that nan
and NULL
are two different things. NULL
value indicates something that doesn’t exist and is empty.
In Python, we deal with such values very frequently in different objects. So it is necessary to detect such constants.
In Python, we have the isnan()
function, which can check for nan
values. And this function is available in two modules- NumPy
and math
. The isna()
function in the pandas
module can also check for nan
values.
Use the math.isnan()
Function to Check for nan
Values in Python
The isnan()
function in the math
library can be used to check for nan
constants in float objects. It returns True
for every such value encountered. For example:
import math
import numpy as np
b = math.nan
print(np.isnan(b))
Output:
True
Note that the math.nan
constant represents a nan
value.
Use the numpy.isnan()
Function to Check for nan
Values in Python
The numpy.isnan()
function can check in different collections like lists, arrays, and more for nan
values. It checks each element and returns an array with True
wherever it encounters nan
constants. For example:
import numpy as np
a = np.array([5, 6, np.NaN])
print(np.isnan(a))
Output:
[False False True]
np.NaN()
constant represents also a nan
value.
Use the pandas.isna()
Function to Check for nan
Values in Python
The isna()
function in the pandas
module can detect NULL
or nan
values. It returns True
for all such values encountered. It can check for such values in a DataFrame or a Series object as well. For example,
import pandas as pd
import numpy as np
ser = pd.Series([5, 6, np.NaN])
print(pd.isna(ser))
Output:
0 False
1 False
2 True
dtype: bool
Use the obj != obj
to Check for nan
Values in Python
For any object except nan
, the expression obj == obj
always returns True
. For example,
print([] == [])
print("1" == "1")
print([1, 2, 3] == [1, 2, 3])
print(float("nan") == float("nan"))
Therefore, we could use obj != obj
to check if the value is nan
. It is nan
if the return value is True
.
import math
b = math.nan
def isNaN(num):
return num != num
print(isNaN(b))
Output:
True
This method however, might fail with lower versions of Python (<=Python 2.5).
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn