在 Python 中检查 NaN 值
-
使用
math.isnan()
函数检查 Python 中的nan
值 -
使用
numpy.isnan()
函数来检查 Python 中的nan
值 -
使用
pandas.isna()
函数检查 Python 中的nan
值 -
使用
obj != obj
检查 Python 中的nan
值
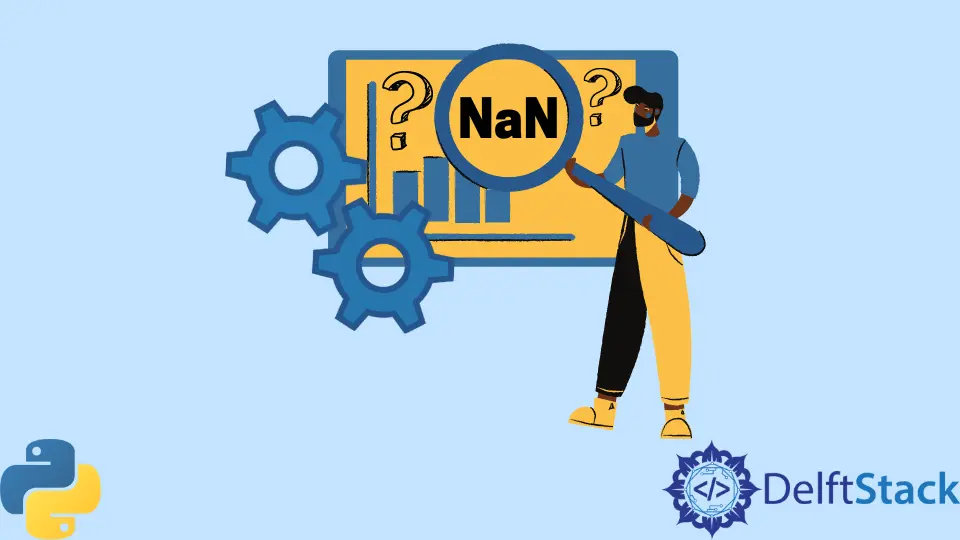
nan
是一个常数,表示给定的值不合法-Not a Number
。
注意,nan
和 NULL
是两个不同的东西。NULL
值表示不存在的东西,是空的。
在 Python 中,我们经常在不同的对象中处理这样的值。所以有必要检测这样的常量。
在 Python 中,我们有 isnan()
函数,它可以检测 nan
值。而这个函数在两个模块中可用-NumPy
和 math
。pandas
模块中的 isna()
函数也可以检查 nan
值。
使用 math.isnan()
函数检查 Python 中的 nan
值
math
库中的 isnan()
函数可用于检查浮点对象中的 nan
常数。它对遇到的每一个这样的值都返回 True
。比如说:
import math
import numpy as np
b = math.nan
print(np.isnan(b))
输出:
True
请注意,math.nan
常量代表一个 nan
值。
使用 numpy.isnan()
函数来检查 Python 中的 nan
值
numpy.isnan()
函数可以在不同的集合,如列表、数组等中检查 nan
值。它检查每个元素,并在遇到 nan
常量时返回带有 True
的数组。例如:
import numpy as np
a = np.array([5, 6, np.NaN])
print(np.isnan(a))
输出:
[False False True]
np.NaN()
常量也代表一个 nan
值。
使用 pandas.isna()
函数检查 Python 中的 nan
值
pandas
模块中的 isna()
函数可以检测 NULL
或 nan
值。它对所有遇到的此类值返回 True
。它还可以检查 DataFrame 或 Series 对象中的此类值。例如,
import pandas as pd
import numpy as np
ser = pd.Series([5, 6, np.NaN])
print(pd.isna(ser))
输出:
0 False
1 False
2 True
dtype: bool
使用 obj != obj
检查 Python 中的 nan
值
对于除 nan
以外的任何对象,表达式 obj == obj
总是返回 True
。例如:
print([] == [])
print("1" == "1")
print([1, 2, 3] == [1, 2, 3])
print(float("nan") == float("nan"))
因此,我们可以使用 obj != obj
来检查值是否为 nan
。如果返回值为 True
,则为 nan
。
import math
b = math.nan
def isNaN(num):
return num != num
print(isNaN(b))
输出:
True
然而,这个方法在 Python 的低版本中可能会失败 (<=Python 2.5)。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn