How to Calculate the Slope in Python
- Use the User-Defined Function to Calculate the Slope of a Given Line in Python
-
Use the
SciPy
Module to Calculate the Slope of a Given Line in Python -
Use the
NumPy
Module to Calculate the Slope of a Given Line in Python - Conclusion
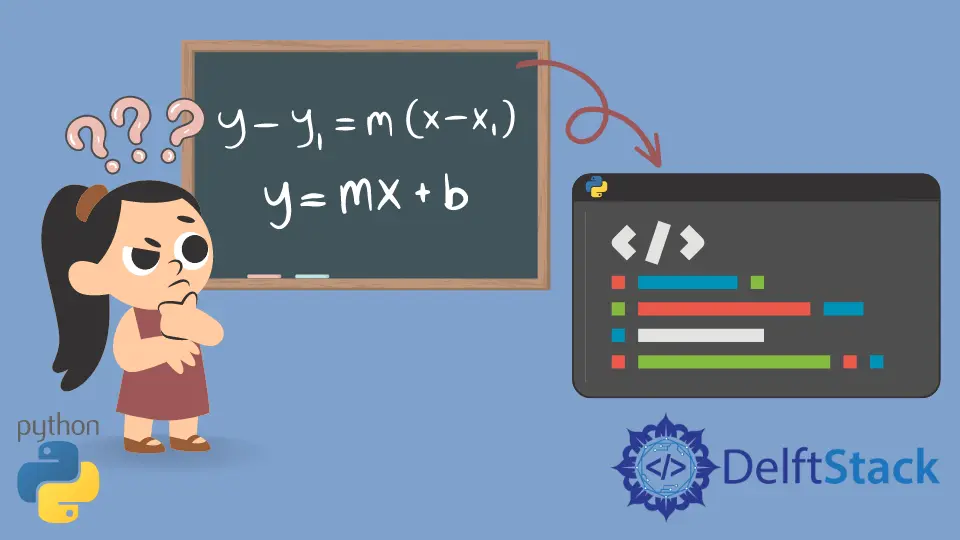
In Mathematics, the slope of a given line is a value that computes its steepness. It also helps in characterizing the direction of a given line. Fortunately, Python provides several methods to calculate the slope of a line, making it a versatile tool for mathematical computations.
This article will demonstrate the different methods available to calculate the slope of a given line in Python.
Use the User-Defined Function to Calculate the Slope of a Given Line in Python
The mathematical formula for the slope of a given line is shown below.
m = (y2-y1)/(x2-x1)
Syntax:
def slope(x1, y1, x2, y2):
x = (y2 - y1) / (x2 - x1)
return x
In the syntax, we define a Python function named slope
that calculates the slope between two points (x1, y1)
and (x2, y2)
using the (y2 - y1) / (x2 - x1)
formula and returns the result.
The syntax defines a function named slope
with four parameters:
x1
: This parameter represents the x-coordinate of the first point.y1
: This parameter represents the y-coordinate of the first point.x2
: This parameter represents the x-coordinate of the second point.y2
: This parameter represents the y-coordinate of the second point.
These four parameters are used to calculate the slope of the line passing through the two points (x1, y1)
and (x2, y2)
using the (y2 - y1) / (x2 - x1)
formula.
In the example below, we create a custom function in Python to compute the slope of a line given two sets of coordinates (x1, y1)
and (x2, y2)
. The following code uses a user-defined function slope
to calculate the slope of a given line in Python.
def slope(x1, y1, x2, y2):
x = (y2 - y1) / (x2 - x1)
return x
print(slope(4, 5, 8, 10))
We define a function named slope
that takes four arguments: x1
, y1
, x2
, and y2
. Then, we calculate the slope using the (y2 - y1) / (x2 - x1)
formula.
Next, we return the calculated slope (x
) as the result of the function. Lastly, the print(slope(4, 5, 8, 10))
line calls the slope
function with the arguments (4
, 5
, 8
, 10
) and then prints the result and these values represent the coordinates of two points (x1, y1) = (4, 5)
and (x2, y2) = (8, 10)
.
Output:
1.25
The output is the result of calling the slope
function with the provided arguments. We compute the slope of the line going through the points (4
, 5
) and (8
, 10
) and return the value 1.25
.
Use the SciPy
Module to Calculate the Slope of a Given Line in Python
SciPy
, an abbreviation for Scientific Python
, is a scientific computing of data in Python that offers powerful tools for various mathematical operations. Additionally, SciPy
is heavily dependent on the NumPy
library.
Apart from NumPy
, SciPy
contains a lot more modules used for stats, linear algebra, image processing, and optimization.
Syntax:
from scipy.stats import linregress
x = [4, 8]
y = [5, 10]
slope, intercept, r_value, p_value, std_err = linregress(x, y)
In the syntax, we import the linregress
function from the scipy.stats
module, calculates linear regression statistics, including the slope, for the provided data points in lists x
and y
, and stores the result in the variables slope
, intercept
, r_value
, p_value
, and std_err
.
The syntax involves calling the linregress
function from the scipy.stats
module with two parameters:
x
: This parameter represents the independent variable, typically a list or array of numeric values. In the context of linear regression, it represents the x-coordinates of data points.y
: This parameter represents the dependent variable, typically a list or array of numeric values. It corresponds to the y-coordinates of data points associated with the independent variablex
.
The linregress
function calculates linear regression statistics, including the slope, intercept, correlation coefficient (r_value
), p-value (p_value
), and standard error (std_err
), based on the relationship between the x
and y
data provided as input.
In the following code, we used the linregress()
method of the SciPy
module to calculate the slope of a given line in Python.
from scipy.stats import linregress
x = [4, 8]
y = [5, 10]
slope, intercept, r_value, p_value, std_err = linregress(x, y)
print(slope)
In the code above, we import the linregress
function from the scipy.stats
module. Then, we define two lists containing data points, x = [4, 8]
and y = [5, 10]
.
Next, we use linregress
to calculate linear regression statistics, including the slope(slope, intercept, r_value, p_value, std_err = linregress(x, y)
). Lastly, we print the calculated slope.
Output:
1.25
In the output, we print the result of the calculated slope(1.25
). The code calculates the linear regression statistics for the data points (4, 5
) and (8, 10
).
Use the NumPy
Module to Calculate the Slope of a Given Line in Python
NumPy
is a library provided by Python that deals with arrays and gives functions for operating on these arrays. The np.polyfit()
function, contained within the NumPy
library, can be utilized to find and return the slope and intercept of the given particular line with the set of coordinates of a line defined as arrays.
Syntax:
import numpy as np
x = [4, 8]
y = [5, 10]
slope, intercept = np.polyfit(x, y, 1)
In the syntax, we use NumPy
to perform linear regression and calculate the slope and intercept for the provided data points in lists x
and y
.
The syntax involves calling the np.polyfit
function from the NumPy
library with three parameters:
x
: This parameter represents the independent variable, typically a list or array of numeric values. It corresponds to the x-coordinates of data points.y
: This parameter represents the dependent variable, typically a list or array of numeric values. It corresponds to the y-coordinates of data points associated with the independent variablex
.deg
: This parameter specifies the degree of the polynomial to fit the data. In the provided syntax, the parameterdeg
is explicitly specified as1
.
The function returns the polynomial coefficients that best suit the data, including the slope and intercept. In the syntax, the calculated slope is assigned to the variable slope
, and the calculated intercept is assigned to the variable intercept
.
In the following code, we use the np.polyfit()
function to calculate the slope of a given line in Python.
import numpy as np
x = [4, 8]
y = [5, 10]
slope, intercept = np.polyfit(x, y, 1)
print(slope)
In the code above, we import the numpy
library and alias it as np
. Then, we define two lists containing data points, x = [4, 8]
and y = [5, 10]
.
Next, we use the np.polyfit()
function to perform linear regression and calculate the slope and intercept. Lastly, we print the calculated slope.
Output:
1.2499999999999993
In the output, we print the calculated slope(1.2499999999999993
), which we get from calculating the linear regression coefficients for the data points (4, 5
) and (8, 10
).
Conclusion
Calculating the slope of a line is an essential mathematical operation, and Python provides multiple approaches to achieve this. Whether we prefer to create a custom function, use the SciPy
library, or leverage the power of NumPy
, Python offers versatile tools for solving mathematical problems.
Understanding how to calculate the slope is a useful skill that may be used in a variety of scientific and engineering applications.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook