How to Calculate the Inverse Tangent in Python
-
Use the
arctan()
Function From theNumPy
Library to Calculate the Inverse Tangent in Python -
Use the
atan()
Function From theSymPy
Library to Calculate the Inverse Tangent in Python -
Use the
atan()
Function From theMath
Library to Calculate the Inverse Tangent in Python
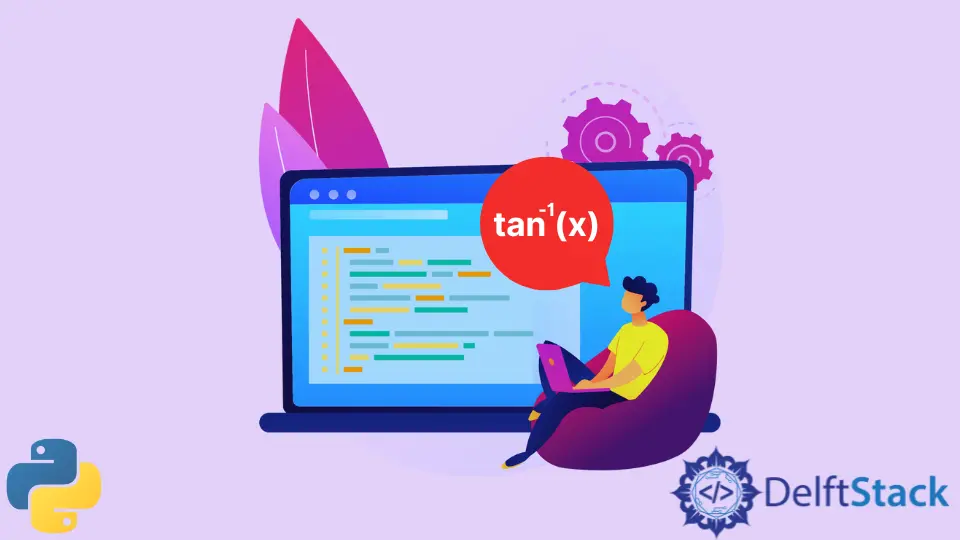
In Python, one can perform many kinds of tasks, including mathematical operations like add, subtract, divide, and deal with matrices. Besides all these operations, you can also perform complex mathematical functions like trigonometric operations using many libraries and modules provided by Python.
This tutorial will show you methods of how to calculate the inverse tangent in Python.
Use the arctan()
Function From the NumPy
Library to Calculate the Inverse Tangent in Python
The NumPy
library is widely used in Python. It helps you deal with arrays, matrices, linear algebra, and Fourier transforms. Additionally, the term NumPy
stands for Numerical Python
.
The NumPy
library includes an operation known as the arctan()
function, which is a mathematical function used to calculate the inverse tangent of elements in an array.
Example:
import numpy as np
array = [-1, 0, 0.5, 1]
print("Array: ", array)
Inv_Tan = np.arctan(array)
print("Inverse Tangent values of elements in the given Array : ", Inv_Tan)
Output:
Array: [-1, 0, 0.5, 1]
Inverse Tangent values of elements in the given Array : [-0.78539816 0. 0.46364761 0.78539816]
This function calculates the inverse tangent values of every element present in the input array.
Use the atan()
Function From the SymPy
Library to Calculate the Inverse Tangent in Python
The SymPy
library of Python is an open-source library of Python used for symbolic mathematics and computer algebra.
The atan()
function of the SymPy
library is used for calculating the inverse tangent value of a given input value in Python. Check the example code below.
from sympy import *
inv_tan1 = atan(0)
inv_tan2 = atan(0.5)
print(inv_tan1)
print(inv_tan2)
Output:
0
0.463647609000806
Use the atan()
Function From the Math
Library to Calculate the Inverse Tangent in Python
Python’s Math
library helps in performing complex mathematical problems by providing different mathematical constants and functions in Python.
The atan()
function of the Math
library is also used for calculating the inverse tangent value (between -PI/2 and PI/2) of a given input value in Python.
Example:
import math
print(math.atan(0))
print(math.atan(0.5))
Output:
0.0
0.46364760900080615
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn