How to Plot Grouped Data in Pandas
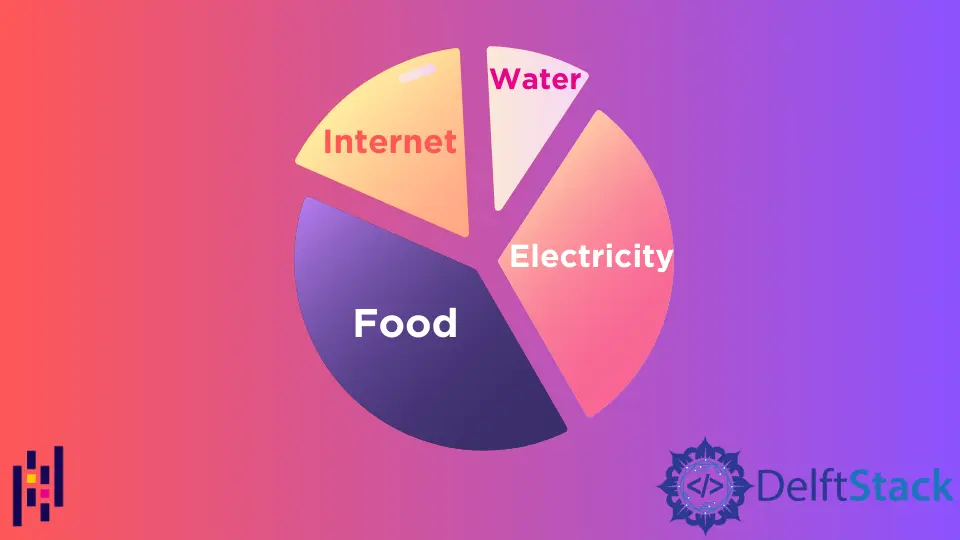
Pandas is an open-source data analysis library in Python. It provides many built-in methods to perform operations on numerical data.
groupby()
is one of the methods available in Pandas that divides the data into multiple groups according to some criteria. We can further plot the grouped data for a better data visualization using Matplotlib in Python.
This tutorial will demonstrate plotting the data of a grouped data in Pandas Python.
Plot Grouped Data Using a Bar Chart in Pandas
We have the students’ data in the Pandas data frame in the following example. First, we will group the data according to the department using the groupby('Department')
method.
Now, the data will be split into two groups. Then we will calculate the maximum marks achieved in each department using the max()
function on the grouped data.
Finally, we will display each department’s highest score using the plot()
function and specify the type of graph as a bar chart in the argument. As an output, we will get a bar chart showing the maximum score of each department.
Example Code:
# Python 3.x
import pandas as pd
import matplotlib.pyplot as plt
df = pd.DataFrame(
{
"Name": ["Robert", "Sam", "Alia", "Jhon", "Smith"],
"Department": ["CS", "SE", "SE", "SE", "CS"],
"Marks": [60, 70, 59, 51, 80],
"Age": [20, 21, 20, 22, 23],
}
)
display(df)
df.groupby("Department")["Marks"].max().plot(kind="bar", legend=True)
plt.show()
Output:
Plot Grouped Data Using a Pie Chart in Pandas
In this example, we have the students’ data with their marks in each subject. First, we will group the data according to the names, calculate each student’s average marks, and plot them using a pie chart.
Every color or sector of the pie chart indicates the mean marks of each student.
Example Code:
# Python 3.x
import pandas as pd
import matplotlib.pyplot as plt
df = pd.DataFrame(
{
"Name": ["Robert", "Robert", "Alia", "Alia", "Smith", "Smith"],
"Subject": ["Java", "Python", "Java", "Python", "Java", "Python"],
"Marks": [60, 70, 59, 51, 80, 70],
}
)
display(df)
df.groupby("Name")["Marks"].mean().plot(kind="pie")
plt.show()
Output:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn