How to Reverse Pandas Dataframe
-
Use the
loc
Method to Reverse Data Frame Without an Error in Pandas - The Wrong Way to Reverse Pandas Data Frame in Python
-
Workflow of Data Fame in
reversed()
Function
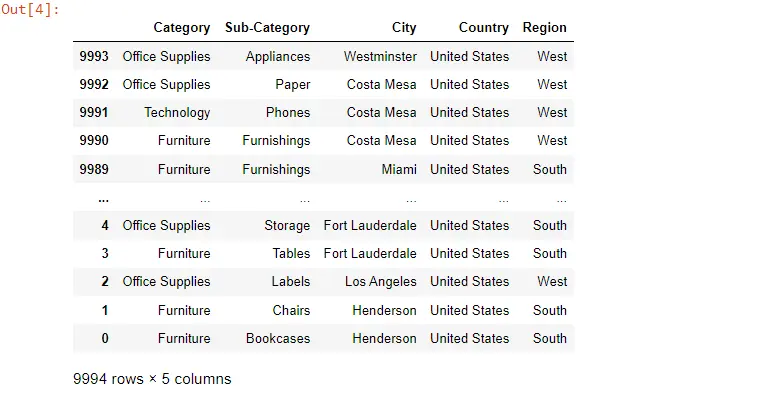
We will learn how to reverse the rows and columns and see how we can reset the index as well. We will also learn why some beginners get failed when they try to reverse data frames in Pandas.
Use the loc
Method to Reverse Data Frame Without an Error in Pandas
Now we will start jumping into the code and import the pandas
library and data set, a sample superstore data coming from an Excel file located in the same folder. If we check the sample superstore, we will see a huge amount of data with several columns.
import pandas as pd
Data = pd.read_excel("demo_Data.xls")
Data.head()
Now we will take a few columns so that it will be easy for us to understand and also so that it will make more sense. So, first of all, we will go ahead and select a few columns that are considered for data manipulation.
data = Data[["Category", "Sub-Category", "City", "Country", "Region"]]
data.head()
Our task is to reverse the rows and columns, and first, we check our total number of records and the total number of columns.
len(data), len(data.columns)
Output:
(9994, 5)
It is time for us to do the reverse data, so we will use the following piece of code to reverse the data frame.
data.loc[::-1]
We can see that the data is now reversed using the loc
method.
We know how to reverse the data frame, but our data frame index starts from 999, so we have to reset the index. To do that, we will use the reset_index()
method, and the drop
argument will be True
.
data.loc[::-1].reset_index(drop=True)
Now, if we execute it, we can see the index is reset and changed 9993 with 0, the first record earlier, and all records are the same.
If we go ahead and change the columns in descending order, the first column should be Region
, then Country
and so on. To do this, we will use the same code above but with a minor change.
data.loc[:, ::-1]
This is how we can reverse the column using the loc
method and after the comma, which means that we are reversing the column and before the comma, which means selecting all rows.
The Wrong Way to Reverse Pandas Data Frame in Python
Some beginners reverse data frames incorrectly and get an error because they have less knowledge of how to use the reversed()
function with a data frame.
Consider the following code.
data = Data[["Category", "Sub-Category", "City", "Country", "Region"]]
for i in reversed(data):
print(data["Category"], data["Country"])
Output:
Why do beginners use the above code snippet to reverse the data frame? Well, since the reversed()
function reverse the data types we put, they try to reverse data in this way.
Consider the following code.
data = ["Category", "Sub-Category", "City", "Country", "Region"]
for i in reversed(data):
print(i)
We can see that the above code perfectly reverses because the data type is a list, and the dataframe works differently.
Region
Country
City
Sub-Category
Category
Workflow of Data Fame in reversed()
Function
Behind the scene, some implementations happen when we supply dataframe in the reversed()
function.
reversed()
calls thelen()
function and put data frame inside it to get its length.- Call the
range()
function and put its length. - Start iteration in reversed order.
Using the reversed()
function, the control gets the length of the dataframe first, then puts it inside the range()
function and starts iterating in reversed order.
After that, the control gets the first element and is enclosed in a square bracket such as data[9993]
; this is where we get the error.
And, 9993 does not exist in the dataframe because 9993 is an index, not a column name. You can use the correct approach mentioned in the above section.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn