Pandas groupby() and diff()
- Understanding the Pandas groupby() Function
- Exploring the Pandas diff() Function
- Combining groupby() and diff() for Advanced Analysis
- Conclusion
- FAQ
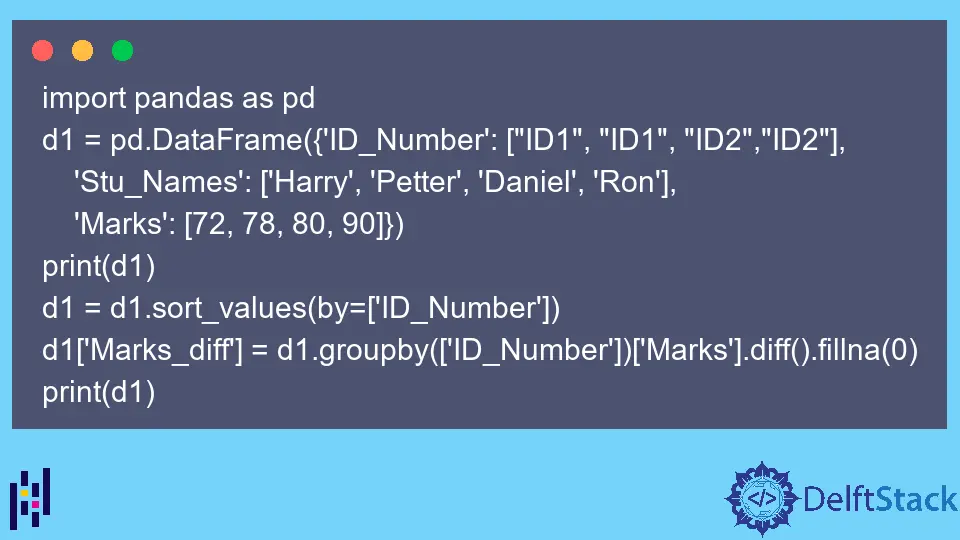
Data analysis often involves grouping data into categories and applying functions to those categories. In Python, the Pandas library provides powerful tools for performing these tasks efficiently. Two essential functions in Pandas are groupby()
and diff()
. The groupby()
function allows you to group your data based on one or more keys, while diff()
calculates the difference between consecutive values in a grouped dataset.
In this article, we will explore both functions in detail, providing clear examples and explanations to help you understand how to use them effectively in your data analysis projects.
Understanding the Pandas groupby() Function
The groupby()
function in Pandas is a versatile tool that allows you to segment your data into groups based on specific criteria. Once the data is grouped, you can perform various operations on each group, such as aggregation, transformation, or filtering. This is particularly useful when you want to analyze large datasets by breaking them down into manageable parts.
To illustrate how groupby()
works, let’s consider a simple example where we have a DataFrame containing sales data for different products across various regions. We will group the data by the product category and calculate the total sales for each category.
import pandas as pd
data = {
'Product': ['A', 'B', 'A', 'B', 'C', 'A', 'C', 'B'],
'Sales': [200, 150, 300, 200, 250, 400, 300, 200],
'Region': ['North', 'South', 'East', 'West', 'North', 'East', 'South', 'West']
}
df = pd.DataFrame(data)
grouped_sales = df.groupby('Product')['Sales'].sum().reset_index()
print(grouped_sales)
Output:
Product Sales
0 A 900
1 B 550
2 C 550
In this example, we first create a DataFrame with sales data. We then use the groupby()
function to group the data by the ‘Product’ column and calculate the sum of sales for each product. The result is a new DataFrame that displays total sales for each product category. This method allows for quick insights into which products are performing well.
Exploring the Pandas diff() Function
Once you have grouped your data, you may want to analyze how values change within those groups. This is where the diff()
function comes in handy. The diff()
function calculates the difference between consecutive values in a DataFrame or Series. When used after groupby()
, it can reveal trends and variations within each group.
Let’s extend our previous example to see how we can use diff()
to find the differences in sales for each product category over time.
sales_data = {
'Product': ['A', 'A', 'A', 'B', 'B', 'B', 'C', 'C'],
'Sales': [200, 300, 400, 150, 200, 250, 250, 300],
'Month': ['January', 'February', 'March', 'January', 'February', 'March', 'January', 'February']
}
df_sales = pd.DataFrame(sales_data)
df_sales['Sales_Diff'] = df_sales.groupby('Product')['Sales'].diff()
print(df_sales)
Output:
Product Sales Month Sales_Diff
0 A 200 January NaN
1 A 300 February 100.0
2 A 400 March 100.0
3 B 150 January NaN
4 B 200 February 50.0
5 B 250 March 50.0
6 C 250 January NaN
7 C 300 February 50.0
In this example, we create a new DataFrame with monthly sales data for different products. After grouping the data by ‘Product’, we apply the diff()
function to the ‘Sales’ column. The result is a new column, ‘Sales_Diff’, which shows the difference in sales from one month to the next for each product. This information can be crucial for identifying trends and making informed business decisions.
Combining groupby() and diff() for Advanced Analysis
The real power of Pandas comes when you combine the groupby()
and diff()
functions for more advanced data analysis. By first grouping your data and then calculating the differences, you can gain deeper insights into your dataset.
For instance, let’s say we want to analyze the percentage change in sales for each product category over time. We can achieve this by using both functions together.
df_sales['Sales_Percent_Change'] = df_sales.groupby('Product')['Sales'].pct_change()
print(df_sales)
Output:
Product Sales Month Sales_Diff Sales_Percent_Change
0 A 200 January NaN NaN
1 A 300 February 100.0 1.5
2 A 400 March 100.0 1.333
3 B 150 January NaN NaN
4 B 200 February 50.0 0.333
5 B 250 March 50.0 0.25
6 C 250 January NaN NaN
7 C 300 February 50.0 0.2
In this code snippet, we utilize the pct_change()
function, which computes the percentage change between the current and a prior element. This gives us a new column, ‘Sales_Percent_Change’, that indicates how sales are changing in percentage terms for each product. This is extremely useful for financial analysis, as it allows businesses to understand growth trends and make strategic decisions.
Conclusion
In summary, the Pandas groupby()
and diff()
functions are indispensable tools for data analysis in Python. By grouping your data and calculating differences, you can uncover valuable insights that drive informed decision-making. Whether you’re analyzing sales data, customer behavior, or any other dataset, mastering these functions will significantly enhance your analytical capabilities. As you continue to explore the Pandas library, you’ll find that these functions can be applied in a variety of contexts, making them essential components of your data analysis toolkit.
FAQ
-
what is the purpose of the groupby() function in Pandas?
The groupby() function is used to group data based on one or more keys, allowing for aggregation and analysis of subsets of data. -
how does the diff() function work in Pandas?
The diff() function calculates the difference between consecutive values in a DataFrame or Series, helping to identify trends and variations. -
can I apply multiple functions after using groupby()?
Yes, you can apply multiple aggregation functions after using groupby() by using the agg() method. -
what is the difference between diff() and pct_change()?
The diff() function calculates the absolute difference between consecutive values, while pct_change() computes the percentage change. -
how can I visualize the results of groupby() and diff()?
You can use libraries like Matplotlib or Seaborn to create visualizations based on the results of groupby() and diff() operations.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn