How to Get the Length of List in Python Pandas Dataframe
- Creating a Sample DataFrame
-
Using the
map()
Function with len - Adding the Length Column Using apply()
- Summary of Methods
- Conclusion
- FAQ
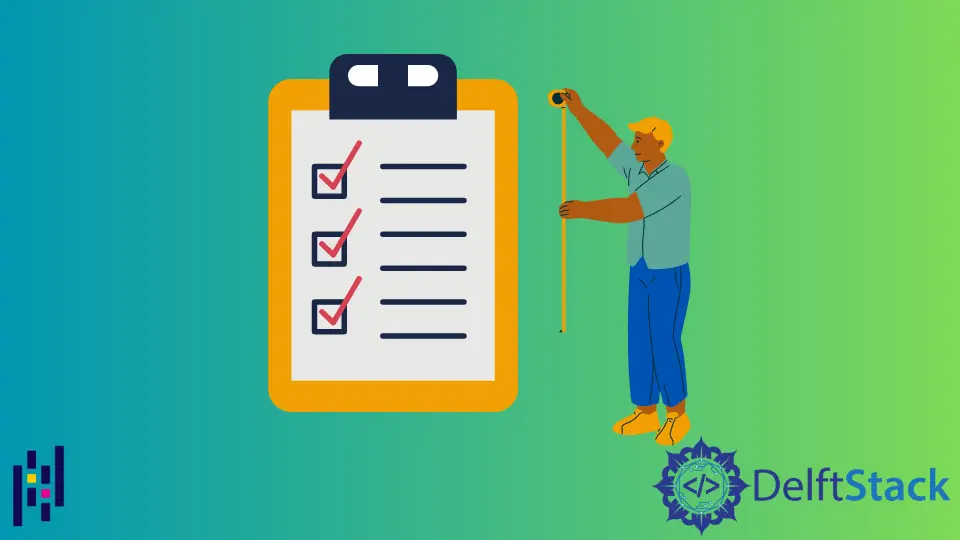
In the world of data manipulation, Python’s Pandas library stands out as a powerful tool for handling data structures like DataFrames. One common task you may encounter is determining the length of lists contained within a DataFrame. This is particularly useful when you’re dealing with lists in cells and want to analyze or visualize their sizes.
In this article, we will guide you through the process of creating a DataFrame, using the map()
function alongside the len
method to compute the length of lists, and ultimately displaying the DataFrame with an added length column. By the end, you’ll have a solid understanding of how to efficiently get the length of lists in your DataFrame.
Creating a Sample DataFrame
To begin, we need to create a sample DataFrame that includes lists. This will serve as our working example. We will use the Pandas library to create a DataFrame with a single column containing lists of varying lengths.
import pandas as pd
data = {
'Lists': [[1, 2, 3], [4, 5], [6], [], [7, 8, 9, 10]]
}
df = pd.DataFrame(data)
print(df)
Output:
Lists
0 [1, 2, 3]
1 [4, 5]
2 [6]
3 []
4 [7, 8, 9, 10]
In this code, we first import the Pandas library. We then create a dictionary called data
, where the key ‘Lists’ maps to a list of lists. Finally, we convert this dictionary into a DataFrame using pd.DataFrame(data)
and print the DataFrame to visualize its structure. The DataFrame consists of one column named ‘Lists’, which contains lists of different lengths.
Using the map()
Function with len
Now that we have a DataFrame with lists, the next step is to compute the length of each list. The map()
function is an efficient way to apply a function to each element in a Series or DataFrame column. Here, we will use it to apply the len
function to each list in the ‘Lists’ column.
df['Length'] = df['Lists'].map(len)
print(df)
Output:
Lists Length
0 [1, 2, 3] 3
1 [4, 5] 2
2 [6] 1
3 [] 0
4 [7, 8, 9, 10] 4
In this snippet, we create a new column named ‘Length’ in our DataFrame df
. We use the map()
function to apply the len
function to each list in the ‘Lists’ column. The result is a new column that contains the lengths of the corresponding lists. This method is straightforward and efficient, allowing you to quickly assess the size of the lists in your DataFrame.
Adding the Length Column Using apply()
Another method to calculate the length of lists in a DataFrame is by using the apply()
function. This function is versatile and can be used to apply a function along an axis of the DataFrame. In our case, we will apply the len
function to the ‘Lists’ column to create the length column.
df['Length'] = df['Lists'].apply(len)
print(df)
Output:
Lists Length
0 [1, 2, 3] 3
1 [4, 5] 2
2 [6] 1
3 [] 0
4 [7, 8, 9, 10] 4
Using apply()
is another effective way to achieve the same result as the map()
function. In this example, we call apply(len)
on the ‘Lists’ column, which computes the length of each list and stores it in the new ‘Length’ column. The output remains the same, demonstrating that both methods are equally valid for this task. The choice between map()
and apply()
often comes down to personal preference or specific use cases.
Summary of Methods
In summary, we have explored two primary methods for determining the length of lists in a Pandas DataFrame: using map()
and apply()
. Both methods are efficient and produce the same output, allowing you to choose based on your coding style or specific needs. The map()
function is typically faster for simple functions like len
, while apply()
offers more flexibility for more complex operations. Understanding these methods will enhance your data manipulation skills in Python and make your work with DataFrames more efficient.
Conclusion
In this article, we have delved into how to get the length of lists in a Pandas DataFrame. We created a sample DataFrame, then utilized both the map()
and apply()
functions to compute the length of lists contained within it. By adding a new column to display these lengths, we enhanced our DataFrame’s usability for further analysis. Mastering these techniques will not only streamline your data operations but also empower you to tackle more complex data manipulation tasks in the future.
FAQ
-
What is a Pandas DataFrame?
A Pandas DataFrame is a two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes (rows and columns). -
How do I install the Pandas library?
You can install the Pandas library using pip by running the commandpip install pandas
in your terminal. -
Can I calculate the length of other data types in a DataFrame?
Yes, you can calculate the length of strings, tuples, and other iterable types using similar methods. -
What is the difference between map() and apply() in Pandas?
Themap()
function is primarily used for element-wise operations on Series, whileapply()
can be used on both Series and DataFrames for more complex operations. -
Can I use lambda functions with map() or apply()?
Yes, bothmap()
andapply()
can accept lambda functions for custom operations.