How to Create an Empty Column in Pandas DataFrame
- Create Empty Column Pandas With the Simple Assignment
-
pandas.DataFrame.reindex()
Method to Add an Empty Column in Pandas -
pandas.DataFrame.assign()
to Add an Empty Column in Pandas DataFrame -
pandas.DataFrame.insert()
to Add an Empty Column to a DataFrame
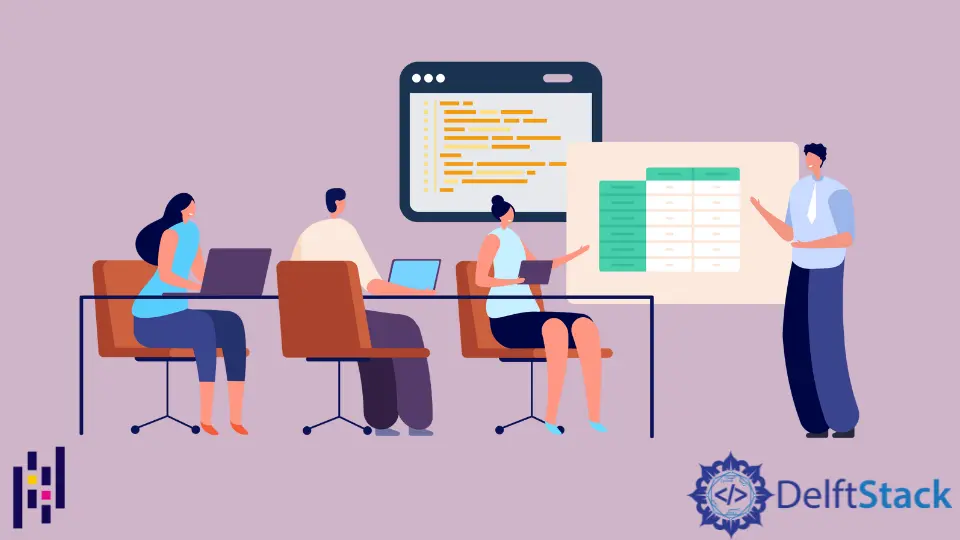
We could use reindex()
, assign()
and insert()
methods of DataFrame
object to add an empty column to DataFrame in Pandas. We can also directly assign an empty value to the column of DataFrame to create an empty column in Pandas.
Create Empty Column Pandas With the Simple Assignment
We can directly assign columns of DataFrame to empty string, NaN
value, or empty Pandas Series
to create an empty column in Pandas.
import pandas as pd
import numpy as np
dates = ["April-20", "April-21", "April-22", "April-23", "April-24", "April-25"]
income = [10, 20, 10, 15, 10, 12]
expenses = [3, 8, 4, 5, 6, 10]
df = pd.DataFrame({"Date": dates, "Income": income, "Expenses": expenses})
df["Empty_1"] = ""
df["Empty_2"] = np.nan
df["Empty_3"] = pd.Series()
print(df)
Output:
Date Income Expenses Empty_1 Empty_2 Empty_3
0 April-20 10 3 NaN NaN
1 April-21 20 8 NaN NaN
2 April-22 10 4 NaN NaN
3 April-23 15 5 NaN NaN
4 April-24 10 6 NaN NaN
5 April-25 12 10 NaN NaN
It creates three empty columns in df
. The column Empty_1
is assigned with the empty string, Empty_2
is assigned with NaN
values, and Empty_3
is assigned with an empty Pandas Series
which also results in NaN
values of the entire Empty_3
column.
pandas.DataFrame.reindex()
Method to Add an Empty Column in Pandas
We can use pandas.DataFrame.reindex()
method to add multiple empty columns to a DataFrame in Pandas.
import pandas as pd
import numpy as np
dates = ["April-20", "April-21", "April-22", "April-23", "April-24", "April-25"]
income = [10, 20, 10, 15, 10, 12]
expenses = [3, 8, 4, 5, 6, 10]
df = pd.DataFrame({"Date": dates, "Income": income, "Expenses": expenses})
column_names = ["Empty_1", "Empty_2", "Empty_3"]
df = df.reindex(columns=column_names)
print(df)
Output:
Empty_1 Empty_2 Empty_3
0 NaN NaN NaN
1 NaN NaN NaN
2 NaN NaN NaN
3 NaN NaN NaN
4 NaN NaN NaN
5 NaN NaN NaN
The code creates new columns Empty_1
, Empty_2
, Empty_3
in df
with all NaN
values while all the old information is lost.
To add multiple new columns while preserving the initial, we could write code as follows:
import pandas as pd
import numpy as np
dates = ["April-20", "April-21", "April-22", "April-23", "April-24", "April-25"]
income = [10, 20, 10, 15, 10, 12]
expenses = [3, 8, 4, 5, 6, 10]
df = pd.DataFrame({"Date": dates, "Income": income, "Expenses": expenses})
df = df.reindex(columns=df.columns.tolist() + ["Empty_1", "Empty_2", "Empty_3"])
print(df)
Output:
Date Income Expenses Empty_1 Empty_2 Empty_3
0 April-20 10 3 NaN NaN NaN
1 April-21 20 8 NaN NaN NaN
2 April-22 10 4 NaN NaN NaN
3 April-23 15 5 NaN NaN NaN
4 April-24 10 6 NaN NaN NaN
5 April-25 12 10 NaN NaN NaN
This adds empty columns Empty_1
, Empty_2
, and Empty_3
to the df
with preserving the initial information.
pandas.DataFrame.assign()
to Add an Empty Column in Pandas DataFrame
We can use pandas.DataFrame.assign()
method to add an empty column to DataFrame in Pandas.
import pandas as pd
import numpy as np
dates = ["April-20", "April-21", "April-22", "April-23", "April-24", "April-25"]
income = [10, 20, 10, 15, 10, 12]
expenses = [3, 8, 4, 5, 6, 10]
df = pd.DataFrame({"Date": dates, "Income": income, "Expenses": expenses})
df = df.assign(Empty_1="", Empty_2=np.nan)
print(df)
Output:
Date Income Expenses Empty_1 Empty_2
0 April-20 10 3 NaN
1 April-21 20 8 NaN
2 April-22 10 4 NaN
3 April-23 15 5 NaN
4 April-24 10 6 NaN
5 April-25 12 10 NaN
It creates an empty column named Empty_1
and Empty_2
containing only NaN values in the df
.
pandas.DataFrame.insert()
to Add an Empty Column to a DataFrame
pandas.DataFrame.insert()
allows us to insert a column in a DataFrame at specified location. We can use this method to add an empty column to a DataFrame.
Syntax:
DataFrame.insert(loc, column, value, allow_duplicates=False)
It creates a new column with the name column
at location loc
with default value value
. allow_duplicates=False
ensures there is only one column with the name column
in the dataFrame. If we pass an empty string or NaN
value as a value parameter, we can add an empty column to the DataFrame.
import pandas as pd
import numpy as np
dates = ["April-20", "April-21", "April-22", "April-23", "April-24", "April-25"]
income = [10, 20, 10, 15, 10, 12]
expenses = [3, 8, 4, 5, 6, 10]
df = pd.DataFrame({"Date": dates, "Income": income, "Expenses": expenses})
df.insert(3, "Empty_1", "")
df.insert(4, "Empty_2", np.nan)
print(df)
Output:
Date Income Expenses Empty_1 Empty_2
0 April-20 10 3 NaN
1 April-21 20 8 NaN
2 April-22 10 4 NaN
3 April-23 15 5 NaN
4 April-24 10 6 NaN
5 April-25 12 10 NaN
It creates Empty_1
column in df
with all empty values at index 3
and Empty_2
at index 4
with all NaN
values.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInRelated Article - Pandas DataFrame Column
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Get the Sum of Pandas Column
- How to Change the Order of Pandas DataFrame Columns
- How to Convert DataFrame Column to String in Pandas