How to Add a New Column to Existing DataFrame With Default Value in Pandas
-
pandas.DataFrame.assign()
to Add a New Column in Pandas DataFrame - Access the New Column to Set It With a Default Value
-
pandas.DataFrame.insert()
to Add a New Column in Pandas DataFrame
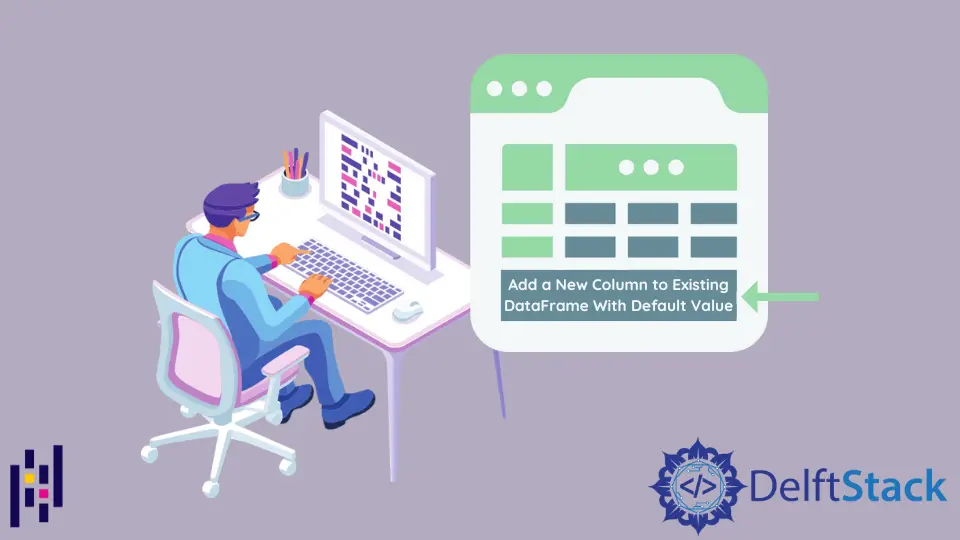
We could use assign()
and insert()
methods of DataFrame
objects to add a new column to the existing DataFrame with default values. We can also directly assign a default value to the column of DataFrame to be created.
We will use the below dataframe as an example in the following sections.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13"]
fruits = ["Apple", "Papaya", "Banana", "Mango"]
prices = [3, 1, 2, 4]
df = pd.DataFrame({"Date": dates, "Fruit": fruits, "Price": prices})
print(df)
Output:
Date Fruit Price
0 April-10 Apple 3
1 April-11 Papaya 1
2 April-12 Banana 2
3 April-13 Mango 4
pandas.DataFrame.assign()
to Add a New Column in Pandas DataFrame
We can use pandas.DataFrame.assign()
method to add a new column to the existing DataFrame and assign the newly created DataFrame
column with default values.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13"]
fruits = ["Apple", "Papaya", "Banana", "Mango"]
prices = [3, 1, 2, 4]
df = pd.DataFrame({"Date": dates, "Fruit": fruits, "Price": prices})
new_df = df.assign(Profit=6)
print(new_df)
Output:
Date Fruit Price Profit
0 April-10 Apple 3 6
1 April-11 Papaya 1 6
2 April-12 Banana 2 6
3 April-13 Mango 4 6
The code creates a new column Profit
in the DataFrame and sets the entire column’s values to be 6
.
Access the New Column to Set It With a Default Value
We can use DataFrame indexing to create a new column in DataFrame and set it to default values.
Syntax:
df[col_name] = value
It creates a new column col_name
in DataFrame df
and sets the default value for the entire column to value
.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13"]
fruits = ["Apple", "Papaya", "Banana", "Mango"]
prices = [3, 1, 2, 4]
df = pd.DataFrame({"Date": dates, "Fruit": fruits, "Price": prices})
df["Profit"] = 5
print(df)
Output:
Date Fruit Price Profit
0 April-10 Apple 3 5
1 April-11 Papaya 1 5
2 April-12 Banana 2 5
3 April-13 Mango 4 5
pandas.DataFrame.insert()
to Add a New Column in Pandas DataFrame
pandas.DataFrame.insert()
allows us to insert a column in a DataFrame at specified location.
Syntax:
DataFrame.insert(loc, column, value, allow_duplicates=False)
It creates a new column with the name column
at location loc
with default value value
. allow_duplicates=False
ensures there is only one column with the name column
in the dataFrame.
import pandas as pd
dates = ["April-10", "April-11", "April-12", "April-13"]
fruits = ["Apple", "Papaya", "Banana", "Mango"]
prices = [3, 1, 2, 4]
df = pd.DataFrame({"Date": dates, "Fruit": fruits, "Price": prices})
df.insert(2, "profit", 4, allow_duplicates=False)
print(df)
Output:
Date Fruit profit Price
0 April-10 Apple 4 3
1 April-11 Papaya 4 1
2 April-12 Banana 4 2
3 April-13 Mango 4 4
Here, a column with the name profit
is inserted at index 2
with the default value 4
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInRelated Article - Pandas DataFrame Column
- How to Get Pandas DataFrame Column Headers as a List
- How to Delete Pandas DataFrame Column
- How to Convert Pandas Column to Datetime
- How to Get the Sum of Pandas Column
- How to Change the Order of Pandas DataFrame Columns
- How to Convert DataFrame Column to String in Pandas