How to Upload Files With FTP in PowerShell
-
Use the
System.Net.WebClient
Object to Upload Files With FTP in PowerShell -
Use the
FtpWebRequest
Object to Upload Files With FTP in PowerShell - Upload Multiple Files With FTP in PowerShell
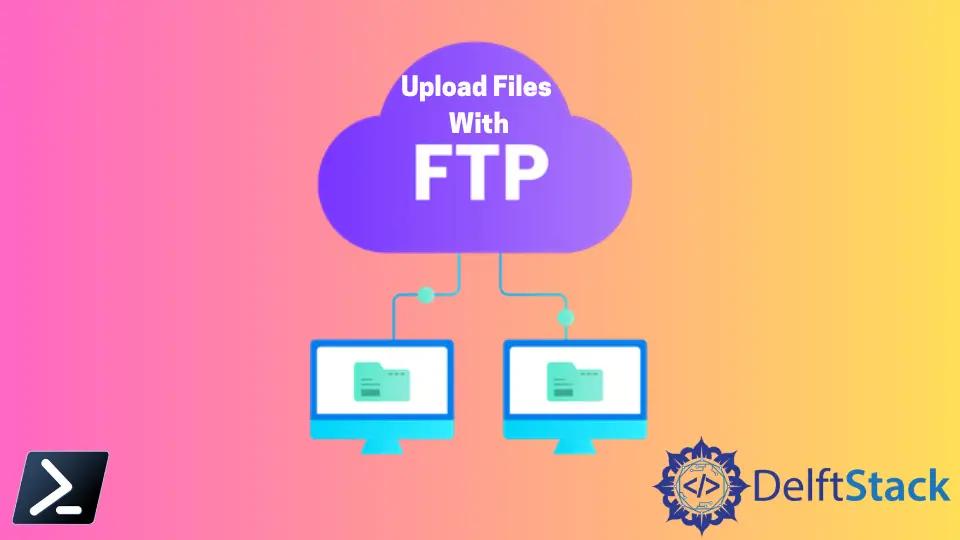
FTP (File Transfer Protocol) is a standard network protocol used to transfer files between a client and server on a computer network. An FTP server is a common solution to facilitate file transfers across the internet.
We can upload files to an FTP server and download files from an FTP server. PowerShell is a scripting tool that can automate FTP upload and download tasks.
This tutorial will teach you to upload files to an FTP server using PowerShell.
Use the System.Net.WebClient
Object to Upload Files With FTP in PowerShell
We can use the System.Net.WebClient
object to upload files to an FTP server. It sends or receives data from any local, intranet, or Internet resource identified by a URI.
First, we need to create an instance of a System.Net.WebClient
object and assign it to a variable $WebClient
. The New-Object
cmdlet creates a .NET Framework or COM object instance.
$WebClient = New-Object System.Net.WebClient
Now, let’s specify a file and FTP URI. Use a valid user name and password for the server. So, replace the value in $FTP
with your FTP details.
$File = "C:\New\car.png"
$FTP = "ftp://ftp_user:ftp_password@ftp_host/car.png"
After that, create the System.URI
object and assign it to a variable, as shown below.
$URI = New-Object System.Uri($FTP)
The final step is to use the UploadFile
method. Then the file will be uploaded to the FTP server.
$WebClient.UploadFile($URI, $File)
The full script is shown below.
$WebClient = New-Object System.Net.WebClient
$File = "C:\New\car.png"
$FTP = "ftp://ftp_user:ftp_password@ftp_host/car.png"
$URI = New-Object System.Uri($FTP)
$WebClient.UploadFile($URI, $File)
Use the FtpWebRequest
Object to Upload Files With FTP in PowerShell
This method will create an instance of a FtpWebRequest
object to upload files to the FTP server in PowerShell.
At first, define the FTP and file details.
$username = "ftp_user"
$password = "ftp_password"
$local_file = "C:\New\test.txt"
$remote_file = "ftp://ftp_host/test.txt"
Then create a FtpWebRequest
object and configure it as shown below. The Credentials
property is used to specify the credentials for connecting to an FTP server.
The UseBinary
property sets a Boolean value to specify the data type for file transfers. True
indicates that the data to be transferred is binary
, and false
indicates that the data to be transferred is text
.
This method uses a binary transfer mode to transfer files without modification or conversion. As a result, the same file on the source computer will be uploaded to the server.
$FTPRequest = [System.Net.FtpWebRequest]::Create("$remote_file")
$FTPRequest = [System.Net.FtpWebRequest]$FTPRequest
$FTPRequest.Method = [System.Net.WebRequestMethods+Ftp]::UploadFile
$FTPRequest.Credentials = New-Object System.Net.NetworkCredential($username, $password)
$FTPRequest.UseBinary = $true
$FTPRequest.UsePassive = $true
# Read the file for upload
$FileContent = gc -en byte $local_file
$FTPRequest.ContentLength = $FileContent.Length
# Get stream request by bytes
$run = $FTPRequest.GetRequestStream()
$run.Write($FileContent, 0, $FileContent.Length)
# Cleanup
$run.Close()
$run.Dispose()
When using a FtpWebRequest
object to upload a file to a server, we must write the file content to the request stream obtained by calling the GetRequestStream
method.
We must write to the stream and close it before sending the request.
Upload Multiple Files With FTP in PowerShell
Sometimes, we might need to upload multiple files to an FTP server. Uploading files one by one will take a lot of time.
So, we have created this PowerShell script to upload multiple files to an FTP server in one go.
First, create a System.Net.WebClient
object and assign it to a $WebClient
variable.
$WebClient = New-Object System.Net.WebClient
Specify the directory where all files that you want to upload are present.
$Dir = "C:\New"
Then specify the URI of your FTP server.
$FTP = "ftp://ftp_user:ftp_password@ftp_host/docs/"
After that, use the foreach
loop to run the command against each item in the $Dir
. The following code uploads all .txt
files in the $Dir
to an FTP server $FTP
.
foreach ($item in (Get-ChildItem $Dir "*.txt")) {
"Uploading $item..."
$URI = New-Object System.Uri($FTP + $item.Name)
$WebClient.UploadFile($URI, $item.FullName)
}
We hope this article helped you upload files with an FTP server in PowerShell.