PowerShell Copy File and Rename
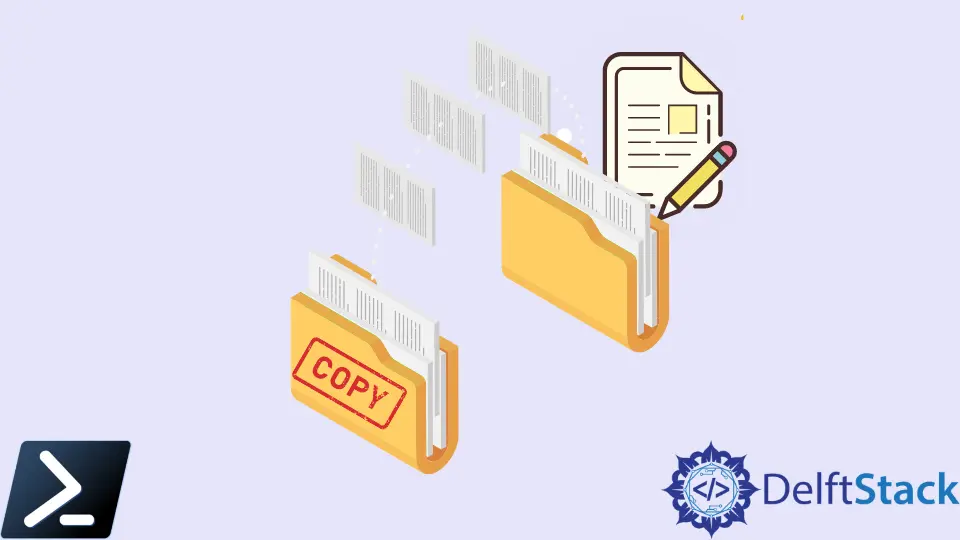
The main aim of this article is to demonstrate the most basic of IO operation, copying a file and then pasting it into another directory or location with a different name.
PowerShell Copy File and Rename
While dealing with CSV files, it can be the case that it is required to copy a specific CSV file and paste it somewhere else with its filename extracted from the CSV.
Consider the following CSV:
iid firstname lastname profession oldfilepath newfilename
-- --------- -------- ---------- ----------- -----------
100 Annecorinne Fitzsimmons doctor D:\\old.csv new2.csv
101 Mallory Dunkin firefighter D:\\old.csv new3.csv
102 Carlie Torray firefighter D:\\old.csv new4.csv
103 Marleah Boycey worker D:\\old.csv new5.csv
104 Lusa Corabella worker D:\\old.csv new6.csv
105 Shandie Chesna worker D:\\old.csv new7.csv
106 Melisent Ochs doctor D:\\old.csv new8.csv
107 Cathie Hurley firefighter D:\\old.csv new9.csv
108 Kerrin Regan doctor D:\\old.csv new10.csv
109 Alie Lewes police officer D:\\old.csv new11.csv
110 Jsandye Gemini police officer D:\\old.csv new12.csv
111 Marguerite Myrilla police officer D:\\old.csv new13.csv
112 Florie Dichy developer D:\\old.csv new14.csv
113 Krystle Gaulin doctor D:\\old.csv new15.csv
114 Odessa Nerita developer D:\\old.csv new16.csv
Now we need to copy this CSV and paste it to another directory with a custom name, which comes from a column from the CSV file. In this case, the column is named newfilename
.
Consider the following code:
$csv = "D:\\old.csv"
Import-Csv $csv | % { Write-Host "Copying $($_.oldfilepath) to D:\TEST\$($_.newfilename)..." | Copy-Item $_.oldfilepath -Destination "D:\TEST\$($_.newfilename)" }
After which, we can verify that file has been copied using the following:
> .\script.ps1
> ls D://TEST
Directory: D:\TEST
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a--- 11/1/2022 10:27 PM 861 new10.csv
-a--- 11/1/2022 10:27 PM 861 new11.csv
-a--- 11/1/2022 10:27 PM 861 new12.csv
-a--- 11/1/2022 10:27 PM 861 new13.csv
-a--- 11/1/2022 10:27 PM 861 new14.csv
-a--- 11/1/2022 10:27 PM 861 new15.csv
-a--- 11/1/2022 10:27 PM 861 new16.csv
-a--- 11/1/2022 10:27 PM 861 new2.csv
-a--- 11/1/2022 10:27 PM 861 new3.csv
-a--- 11/1/2022 10:27 PM 861 new4.csv
-a--- 11/1/2022 10:27 PM 861 new5.csv
-a--- 11/1/2022 10:27 PM 861 new6.csv
-a--- 11/1/2022 10:27 PM 861 new7.csv
-a--- 11/1/2022 10:27 PM 861 new8.csv
-a--- 11/1/2022 10:27 PM 861 new9.csv
Using the above code, we can extract the file to be copied from one of the columns and the name of the new file to be saved.
The overall flow of the program is as follows:
- The path of the CSV file to extract the path and filename from is stored in a variable named
csv
. The$
symbol is mainly used for declaring and accessing/setting variables in PowerShell. - The
Import-csv
command extracts the data from the CSV file. - After the relevant data has been extracted, it is pipelined to the following command using the
|
operator. - The
%
operator (an alias for theforeach
loop) is used to extract data from the columnsoldfilepath iteratively
andnewfilename
, which are then passed to theCopy-Item
command to copy the file from the given source to the destination (not specified in CSV in our case).
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn