How to Loop Through Files and Folders in PowerShell
-
Loop Through Files and Folders Using PowerShell
Get-ChildItem
-
Loop Through Files and Folders Using the PowerShell
Get-ChildItem
andForEach
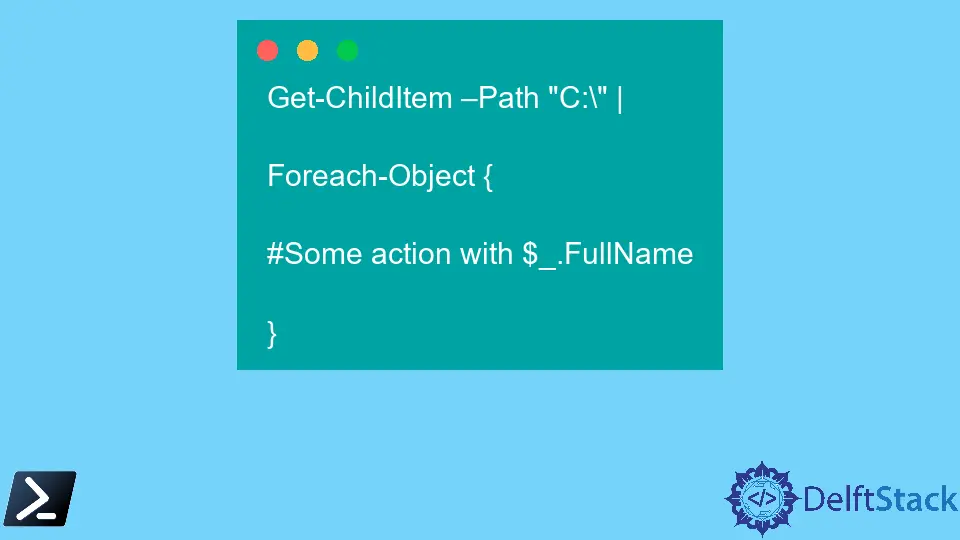
This article illustrates how we can write a PowerShell script to loop through files and folders. We will use the Get-ChildItem
and ForEach
statements to create different PowerShell scripts. Let’s jump right in.
Loop Through Files and Folders Using PowerShell Get-ChildItem
We use the PowerShell Get-ChildItem
cmdlet to display a list of files and folders in a specified location. We use the -path
attribute to specify the path to the desired directory.
The Get-ChildItem
also uses gci
as an alias.
For example, if we want to get a list of the files and folders in our C:/
drive, we can run the following:
Get-ChildItem -Path 'C:\'
The command above will only display the files and folders at the root of our drive. To get the content of the child directories (subfolders), we can add the -Recurse
parameter, as illustrated below.
Get-ChildItem -Path 'C:\' -Recurse
PowerShell will display the content of all the subfolders located in our drive.
Loop Through Files and Folders Using the PowerShell Get-ChildItem
and ForEach
We use the PowerShell ForEach
loop to act as each object in a collection of objects. Here is the general structure when using the Get-ChildItem
cmdlet and the ForEach
loop:
Get-ChildItem –Path "C:\" |
ForEach-Object {
#Some action with $_.FullName
}
Let’s look at some example scripts using the two PowerShell utilities.
Let’s say we want to delete all .csv
files in our C:\Users\pc
folder. How do we go about this?
As illustrated below, we can use the Get-ChildItem
cmdlet and the ForEach
loop to perform this task.
Get-ChildItem –Path "C:\Users\pc" -Recurse -Filter *.csv
|
ForEach-Object {
Remove-Item $_.FullName -WhatIf
}
The script above uses the PowerShell Get-ChildItem
cmdlet with the -Recurse
parameter to get all the files in our C:\Users\pc
folder. We then use the Filter
parameter to fetch files with only a .csv
file extension.
We pipe the results to the ForEach
loop, where the files are removed. However, since we do not want to delete our files, we have added the -WhatIf
parameter. Below is the output of the script above.
Our script has found six files with a .csv
file extension. The files have not yet been deleted since we used the -WhatIf
parameter.
We can also create a script that deletes files older than a certain date. This can come in handy when cleaning up temporary log files from our system.
Below is an example script that deletes files older than 7 days in our pc
directory.
Get-ChildItem C:\Users\pc\ -Recurse
|
Where-Object { $_.CreationTime -lt ($(Get-Date).AddDays(-7)) } |
ForEach-Object { Remove-Item $_.FullName –WhatIf }
This Get-ChildItem
with the Recurse
parameter will fetch all the files in our pc
folder and pie them down to our ForEach
loop. The loop will delete all files older than seven days.
Since we do not want to do away with the files in our folder, we have added the -WhatIf
parameter.
In conclusion, we can use the PowerShell Get-ChildItem
and the ForEach
loop to create scripts that loop through files and folders.
The ForEach
loop will perform a specified action against all the files piped down from the Get-ChildItem
cmdlet. There are different ways we can use the two PowerShell utilities.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn