在 PowerShell 中使用 FTP 上傳檔案
-
在 PowerShell 中使用
System.Net.WebClient
物件通過 FTP 上傳檔案 -
在 PowerShell 中使用
FtpWebRequest
物件通過 FTP 上傳檔案 - 在 PowerShell 中使用 FTP 上傳多個檔案
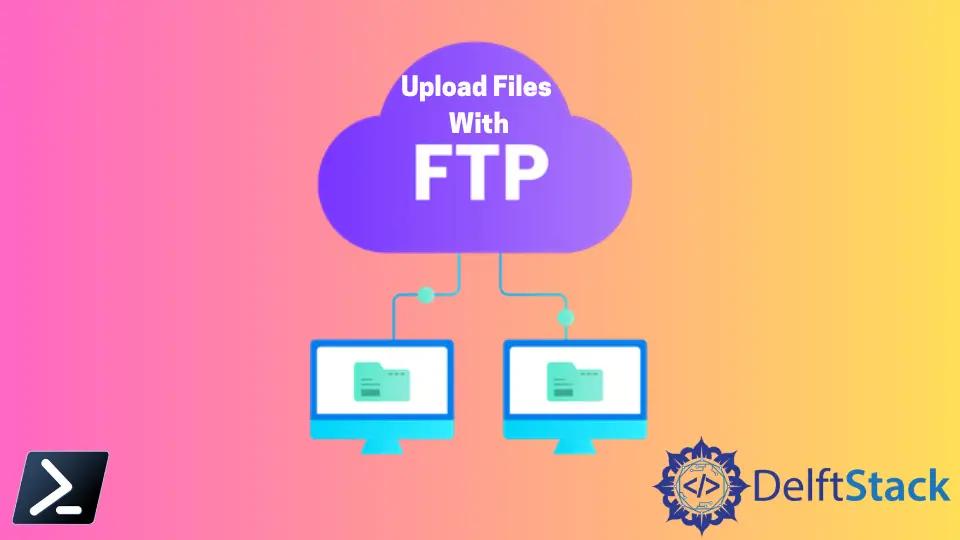
FTP(檔案傳輸協議)是一種標準網路協議,用於在計算機網路上的客戶端和伺服器之間傳輸檔案。FTP 伺服器是一種常見的解決方案,用於促進跨 Internet 的檔案傳輸。
我們可以將檔案上傳到 FTP 伺服器,也可以從 FTP 伺服器下載檔案。PowerShell 是一個指令碼工具,可以自動執行 FTP 上傳和下載任務。
本教程將教你使用 PowerShell 將檔案上傳到 FTP 伺服器。
在 PowerShell 中使用 System.Net.WebClient
物件通過 FTP 上傳檔案
我們可以使用 System.Net.WebClient
物件將檔案上傳到 FTP 伺服器。它從由 URI 標識的任何本地、Intranet 或 Internet 資源傳送或接收資料。
首先,我們需要建立一個 System.Net.WebClient
物件的例項並將其分配給一個變數 $WebClient
。New-Object
cmdlet 建立一個 .NET Framework 或 COM 物件例項。
$WebClient = New-Object System.Net.WebClient
現在,讓我們指定一個檔案和 FTP URI。為伺服器使用有效的使用者名稱和密碼。因此,用你的 FTP 詳細資訊替換 $FTP
中的值。
$File = "C:\New\car.png"
$FTP = "ftp://ftp_user:ftp_password@ftp_host/car.png"
之後,建立 System.URI
物件並將其分配給一個變數,如下所示。
$URI = New-Object System.Uri($FTP)
最後一步是使用 UploadFile
方法。然後檔案將被上傳到 FTP 伺服器。
$WebClient.UploadFile($URI, $File)
完整的指令碼如下所示。
$WebClient = New-Object System.Net.WebClient
$File = "C:\New\car.png"
$FTP = "ftp://ftp_user:ftp_password@ftp_host/car.png"
$URI = New-Object System.Uri($FTP)
$WebClient.UploadFile($URI, $File)
在 PowerShell 中使用 FtpWebRequest
物件通過 FTP 上傳檔案
此方法將建立一個 FtpWebRequest
物件的例項,以將檔案上傳到 PowerShell 中的 FTP 伺服器。
首先,定義 FTP 和檔案詳細資訊。
$username = "ftp_user"
$password = "ftp_password"
$local_file = "C:\New\test.txt"
$remote_file = "ftp://ftp_host/test.txt"
然後建立一個 FtpWebRequest
物件並配置它,如下所示。Credentials
屬性用於指定連線到 FTP 伺服器的憑據。
UseBinary
屬性設定一個布林值來指定檔案傳輸的資料型別。True
表示要傳輸的資料是 binary
,false
表示要傳輸的資料是 text
。
此方法使用二進位制傳輸模式傳輸檔案,無需修改或轉換。結果,源計算機上的相同檔案將被上傳到伺服器。
$FTPRequest = [System.Net.FtpWebRequest]::Create("$remote_file")
$FTPRequest = [System.Net.FtpWebRequest]$FTPRequest
$FTPRequest.Method = [System.Net.WebRequestMethods+Ftp]::UploadFile
$FTPRequest.Credentials = New-Object System.Net.NetworkCredential($username, $password)
$FTPRequest.UseBinary = $true
$FTPRequest.UsePassive = $true
# Read the file for upload
$FileContent = gc -en byte $local_file
$FTPRequest.ContentLength = $FileContent.Length
# Get stream request by bytes
$run = $FTPRequest.GetRequestStream()
$run.Write($FileContent, 0, $FileContent.Length)
# Cleanup
$run.Close()
$run.Dispose()
當使用 FtpWebRequest
物件將檔案上傳到伺服器時,我們必須將檔案內容寫入呼叫 GetRequestStream
方法獲得的請求流中。
我們必須在傳送請求之前寫入流並關閉它。
在 PowerShell 中使用 FTP 上傳多個檔案
有時,我們可能需要將多個檔案上傳到 FTP 伺服器。一個一個地上傳檔案會花費很多時間。
因此,我們建立了這個 PowerShell 指令碼來一次性將多個檔案上傳到 FTP 伺服器。
首先,建立一個 System.Net.WebClient
物件並將其分配給 $WebClient
變數。
$WebClient = New-Object System.Net.WebClient
指定要上傳的所有檔案所在的目錄。
$Dir="C:\New"
然後指定 FTP 伺服器的 URI。
$FTP = "ftp://ftp_user:ftp_password@ftp_host/docs/"
之後,使用 foreach
迴圈對 $Dir
中的每個專案執行命令。以下程式碼將 $Dir
中的所有 .txt
檔案上傳到 FTP 伺服器 $FTP
。
foreach($item in (Get-ChildItem $Dir "*.txt")){
"Uploading $item..."
$URI = New-Object System.Uri($FTP+$item.Name)
$WebClient.UploadFile($URI, $item.FullName)
}
我們希望本文能幫助你在 PowerShell 中使用 FTP 伺服器上傳檔案。