String Interpolation in PowerShell
- What Are Variables in PowerShell?
- String Interpolation in Windows PowerShell
- String Interpolation With Environment Variables in PowerShell
- String Interpolation With Escape Characters in PowerShell
- String Interpolation Using Variables With Special Characters in PowerShell
- String Interpolation Using a Subexpression Operator in PowerShell
- Conclusion
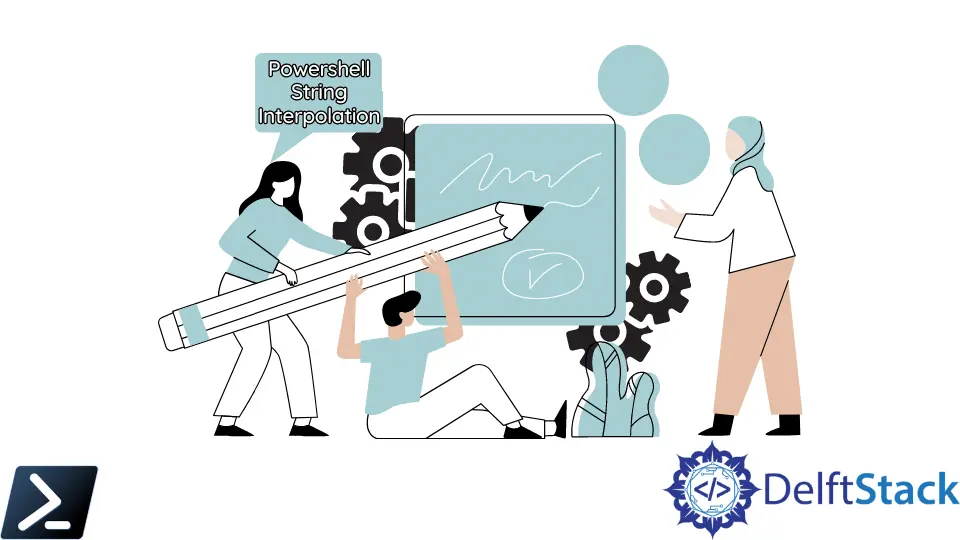
String interpolation in PowerShell is a powerful feature that facilitates dynamic string formatting by embedding variable values, expressions, and commands within strings. This comprehensive guide delves deeply into the intricacies of string interpolation, offering detailed insights, examples, and practical applications of this feature.
String interpolation replaces the value of a variable with placeholders in a string. Also, the process of string interpolation displays the value of the variable in the string.
Windows PowerShell string interpolation provides a more readable, easier-to-read, and convenient syntax to create a formatted string. This article will discuss using variables during string interpolation in Windows PowerShell.
What Are Variables in PowerShell?
Before discussing Windows PowerShell string interpolation, we should first discuss one of the critical requirements to achieve this: the Windows PowerShell variables.
Variables in PowerShell serve as containers for storing data, allowing users to store information and manipulate it throughout the script’s execution. They act as symbolic names representing a specific value or an object, providing a way to reference and work with data within PowerShell scripts.
Key aspects of variables in PowerShell:
Declaration and Naming
Declaration:
Variables in PowerShell are declared using the $
symbol followed by a name.
Example:
$my_var = "Hello World!"
Naming Rules:
- Must start with a letter or an underscore.
- Can contain letters, numbers, and underscores.
- Case-insensitive (
$variable
and$Variable
refer to the same thing).
Data Types
Dynamic Typing:
PowerShell uses dynamic typing, allowing variables to hold different types of data without explicit type declaration.
Example:
$number = 10
$text = "Sample text"
Scope
Variables can have different scopes, determining their visibility and accessibility within a script.
Local: Limited to the current scope or function.
Script: Visible throughout the current script.
Global: Accessible across the entire PowerShell session.
Assignment and Modification
Assignment:
Variables are assigned values using the assignment operator (=
).
Example:
$myVariable = "New value"
Modification:
Variables can be modified by assigning new values or altering existing ones.
Example:
$number = $number + 5
We will use these variables to perform String Interpolation in Windows PowerShell.
String Interpolation in Windows PowerShell
String interpolation refers to the process of embedding variables, expressions, or commands directly within strings to generate dynamic content.
Common Syntax:
- Double-quoted Strings (
"..."
): Strings enclosed in double quotes allow for string interpolation. - Interpolation Syntax:
$variable
,$($expression)
, and$(command)
Interpolating Variables
Let’s understand with an example of connecting string using string interpolation in Windows PowerShell.
Example Code:
$company = "XYZ Company"
Write-Output "Welcome to $company"
In the above PowerShell script, the $company
variable contains the XYZ Company
string value. Inside double quotation marks ""
, Windows PowerShell interpolates the string variable name and displays the result below in the following statement.
Output:
Welcome to XYZ Company
If we put a variable name in a single quotation mark, ''
, it will display the variable name instead, as shown below.
Example Code:
$company = "XYZ Company"
Write-Output 'Welcome to $company'
Output:
Welcome to $company
This output happens because anything enclosed with a single quotation mark treats anything inside as a literal expression. So, make sure that you are using double quotation marks when interpolating a string.
Interpolating Expressions
$age = 25
Write-Host "In five years, I'll be $($age + 5) years old."
The string interpolation, denoted by $()
, allows the inclusion of variable values or expressions within a string. In this case, it dynamically calculates the future age by adding 5
to the value stored in the $age
variable, making the output dynamic and reflecting the expected age after five years.
Interpolating Commands
$currentDate = Get-Date
Write-Host "The current date is $(Get-Date)."
The result will show the current date and time at the instant the Write-Host
line is executed, dynamically incorporating the updated value from the Get-Date
cmdlet within the string via interpolation.
String Interpolation With Environment Variables in PowerShell
Environment variables are dynamic named values stored in the operating system, holding information about the system environment, user preferences, and configuration settings.
Environment variables, depicted by the Env:
variable in Windows PowerShell, store the operating system environment and programs. This information details include the operating system path, location of the Windows installation directory, number of processes used by the operating system, and so much more.
Common Environment Variables:
$env:USERNAME
: Represents the current user’s username.$env:COMPUTERNAME
: Stores the name of the computer.$env:TEMP
: Specifies the temporary files directory.$env:PATH
: Contains the system’s executable file paths.
In Windows PowerShell, environment variables can be interpolated inside a string like other standard variables.
Example Code:
Write-Output "My computer name: $Env:COMPUTERNAME"
In the PowerShell script above, $Env: COMPUTERNAME
displays a variable value in double quotation marks and concatenates it with a string.
Output:
My computer name: WINDOWS-PC01
String Interpolation With Escape Characters in PowerShell
Despite discussing that we should use double quotation marks during string interpolation, certain cases where putting a variable inside double quotation marks doesn’t provide results as expected. One example of this is escape characters.
An escape character is a kind of character that invokes an alternative interpretation of the following characters in a character sequence. Escape characters are common among programming languages as certain symbols are used when compiled programmatically.
Escape characters in PowerShell are special sequences used to represent characters that may have special meanings within strings. They allow the inclusion of special characters or control formatting within strings.
Common Escape Characters:
- Backtick (`): Used to escape characters that have special meanings.
- Double Quotation (
"
): Enables interpolation and expression evaluation within double-quoted strings. - Newline (``n`): Represents a newline character.
- Tab (``t`): Inserts a horizontal tab.
- Carriage Return (``r`): Moves the cursor to the beginning of the line.
For example, in Windows PowerShell, a dollar sign $
is one of the most common operators used to define a variable. We will use the dollar sign as an example below.
Example Code:
$price = 99
Write-Output "Discounted Price: $$price"
In the Windows PowerShell script above, $price
inside double quotation marks doesn’t give the expected output because the scripting environment interprets double dollar signs $$
differently.
Output:
Discounted Price: Discounted Price: $$priceprice
Use Windows PowerShell escape character for string interpolation below to get the desired output.
Example Code:
$price = 99
Write-Output "Discounted Price: `$$price"
Output:
Discounted Price: $99
String Interpolation Using Variables With Special Characters in PowerShell
As mentioned, variable names that begin with a dollar sign $
can include alphanumeric characters and special characters. Variables in PowerShell may contain special characters such as backticks (`), dollar signs ($
), or quotes ("
).
The best practice for defining variables is only to include alphanumeric characters and the underscore _
character. Variable names that include other special characters, including spaces, are difficult to use and should be avoided.
However, we are not saying that using variables with special characters is impossible. If the situation can not avoid this, we can use the dollar sign and curly brackets ${}
to escape all special characters, including spaces, in a variable name.
Example Code:
${this is a variable!} = "Hello"
Write-Output "${this is a variable!} World!"
Output:
Hello World!
The ${this is a variable!}
variable, despite its unconventional name, is created and assigned the value "Hello"
.
The string interpolation within Write-Output
concatenates the value of the variable with the text " World!"
, resulting in the output "Hello World!"
.
String Interpolation Using a Subexpression Operator in PowerShell
The subexpression operator ($()
) in PowerShell allows the evaluation of expressions, commands, or sub-scripts within a string.
In Windows PowerShell, we can use the subexpression operator $()
to run an expression within an expression like string interpolation. We do this by enclosing whichever expression we run with a dollar sign and parentheses ()
.
Example Code:
$num1 = 10
$num2 = 5
Write-Output "$num1 + $num2 = $($num1+$num2)"
Output:
10 + 5 = 15
The string interpolation includes the values of $num1
and $num2
as text (10
and 5
, respectively) alongside the result of the arithmetic operation (15
) obtained by adding $num1
and $num2
.
Conclusion
The article explores PowerShell’s string interpolation, enabling dynamic string formatting by embedding variable values, expressions, and commands within strings. It covers:
- Variables in PowerShell: These containers hold various data types without explicit type declaration and follow specific naming rules.
- String Interpolation Basics: It involves embedding
$variables
,$($expressions)
, and$(commands)
within double-quoted strings for dynamic content. - Usage Examples:
- Utilizing variables for dynamic content within strings.
- Incorporating environment variables (
$Env:VARIABLE_NAME
) for system-related information. - Employing escape characters (`) to handle special characters or formatting in strings.
- Creating and using variables with special characters or spaces using
${}
syntax. - Leveraging the subexpression operator
$()
for complex calculations within strings.
Understanding these techniques in string interpolation provides PowerShell users with powerful tools for dynamic string creation, aiding readability and flexibility in script formatting.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell