How to Select Multiple Patterns in a String Using PowerShell
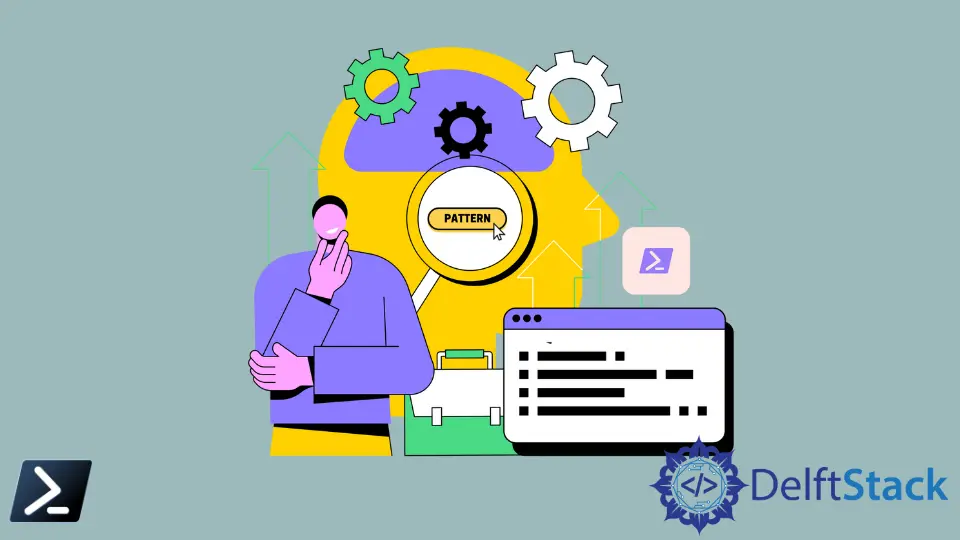
PowerShell has a similar command to Linux’s grep that looks for a string pattern and displays it as output in the command line.
This article discusses which cmdlet serves its function of searching for specific string patterns using Windows PowerShell.
Introduction to the Select-String
Command in Windows
The grep command in Linux is comparable to the Select-String
command in Windows. The cmdlet searches for the first match in each line by default and then displays the file name, line number, and text within the matched string.
The Select-String
command may also work with multiple file encodings, such as Unicode text, by determining the encoding format using the byte-order-mark (BOM). Select-String will think it’s a UTF8 file if the BOM is missing.
Parameters of Select-String
AllMatches
– Usually, theSelect-String
command will only look for the first match in each line; however, the cmdlet will search for more than one match using this parameter. The parameter will still emit a singleMatchInfo
object for each line, containing all matches found.CaseSensitive
– String matches are not case-sensitive by default. This parameter forces the cmdlet to look for matches precisely the input pattern.Context
– A parameter that we can define the number of lines before and after the match that the parameter will display. Appending this parameter modifies the emittedMatchInfo
object to include a new Context property containing the specified lines.Select-String
to anotherSelect-String
call, the context won’t be available since we are only searching on the single resultingMatchInfo
line property.Culture
– Used with theSimpleMatch
parameter, specifies a culture to be matched with the specified pattern. This parameter includes options such asen-US
,es
, orfr-FR
examples.
The Ordinal
and Invariant
choices are two more useful possibilities.
Ordinal
is used for non-linguistic binary comparisons, whileInvariant
is used for independent culture comparisons. This parameter debuted in PowerShell 7 and was not present in previous versions.
Note that this will use the system’s current culture, which we can determine using the Get-Culture
command.
-
Encoding
– Specifies the encoding of the target file to search, which default ofutf8NoBOM
. TheEncoding
option now takes numeric IDs of registered code pages, or string names, such as windows-1251, starting with PowerShell Core 6.2.ASCII
: This parameter uses the encoding for the ASCII (7-bit) character set.bigendianunicode
: This parameter encodes in UTF-16 format using the big-endian byte order.OEM
: This parameter uses the default encoding for MS-DOS and console programs.Unicode
: This parameter encodes in UTF-16 format using the little-endian byte order.utf7
: This parameter encodes in UTF-7 format.utf8
: This parameter encodes in UTF-8 format.utf8BOM
: This parameter encodes in UTF-8 format with Byte Order Mark (BOM)tf8NoBOM
: Encodes in UTF-8 format without Byte Order Mark (BOM)utf32
: Encodes in UTF-32 format.
-
Exclude
– With the Path parameter, exclude specific items using a pattern, such as*.txt
. -
Include
– TheInclude
parameter, like theExclude
parameter, will only include entries that match a pattern, such as*.csv
. -
List
– This parameter returns the first instance of matching text from each input file. This parameter is intended to be a fast and efficient way to retrieve a listing of files with matching contents. -
LiteralPath
tellsSelect-String
to use the values as input instead of interpreting values such as*
as a wildcard. If the path includes escaping characters, enclose them in single quotation marks to not analyze. -
NoEmphasis
– This parameter disables the highlighting of matches. TheEmphasis
parameter uses negative colors based on the background text colors. -
NotMatch
– This parameter looks for text that does not match the specified pattern. -
Path
– The path to the files to be searched is specified by this parameter. We can use wildcards, but we can’t just specify a directory.The local directory is the default.
-
Pattern
– This parameter specifies the pattern to search the input content or files based on regular expressions. -
SimpleMatch
– Instead of regular expressions, this parameter utilizes a basic match. Because no regular expression is used, the Matches field of the returnedMatchInfo
object is empty. -
Raw
– This parameter outputs the matching strings without aMatchInfo
object. This behavior is most similar to grep and not PowerShell’s more object-oriented nature. -
Quiet
– Only returns a Boolean value of$true
or$false
if the pattern is found.
Use Select-String
Cmdlet in Windows PowerShell
Let’s have examples and see how we can leverage Select-String
to make finding text matches easier. Starting with a straightforward example, let us look for John
in a handful of CSV files.
Example Code:
Select-String -Path "PS\*.csv" -Pattern "John"
Output:
PS\user1.csv:3:John,Doe,jdoe@test.com,Male
PS\user2.csv:5:John,Johnson,jjohnson@abccompany.com,Male
We can also look at the display properties of each output.
Example Code:
Select-String -Path "Users\*.csv" -Pattern "John" | Select-Object * -First 1
Output:
IgnoreCase : True
LineNumber : 3
Line : John,Doe,jdoe@test.com,Male
Filename : user1.csv
Path : C:\Temp\PS
Pattern : John
Context :
Matches : {2}
If we want to search for multiple patterns, we can separate the parameter values with a comma under the Pattern parameter.
Example Code:
Select-String -Path "Users\*.csv" -Pattern "John", "Henry", "Jonathan"
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell