How to Remove Path and Extension From Filename in PowerShell
-
Use the
BaseName
Property to Remove Path and Extension From Filename in PowerShell -
Use the
System.IO.Path
Class to Remove Path and Extension From Filename in PowerShell - Conclusion
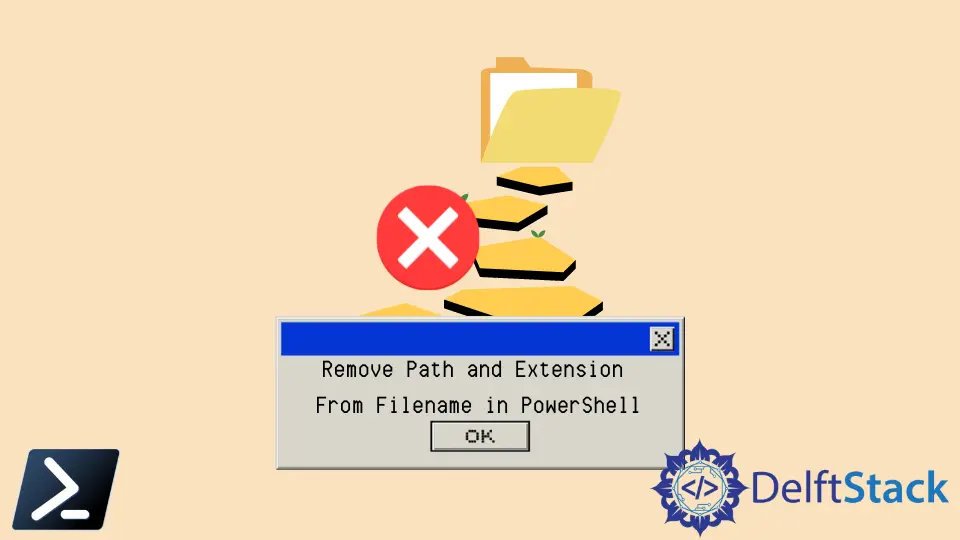
PowerShell supports the handling of various file operations in the system. You can perform tasks such as create, copy, move, rename, edit, delete, and view files in PowerShell.
There are different cmdlets in PowerShell that you can use to get the name full path and extension of a file. While working with files in the system, you might need to get the file name without a path and extension.
This tutorial will teach you to remove the path and extension from the filename in PowerShell.
Use the BaseName
Property to Remove Path and Extension From Filename in PowerShell
The Get-Item
cmdlet in PowerShell is a versatile tool used for retrieving the properties of items in your filesystem, like files and directories. One of these properties is .BaseName
, which is particularly useful for our purpose.
Example:
(Get-Item "sample.txt").BaseName
In this example, the script is assuming sample.txt
is a file in the current directory. If the file is in a different directory, you would include the full path, like C:\folder\sample.txt
.
When we execute (Get-Item "sample.txt").BaseName
, PowerShell performs two key actions.
First, Get-Item "sample.txt"
retrieves the file object corresponding to sample.txt
. Then, .BaseName
accesses the property of this file object that contains the filename without the extension.
Output:
If you want to remove the path and extension in multiple files, you can use the Get-ChildItem
cmdlet. The following command shows how to get multiple file names without a path and extension in PowerShell.
Example
(Get-ChildItem "*.txt").BaseName
This line of code will list the base names (filenames without extensions) of all .txt
files in the specified directory.
-
Get-ChildItem "*.txt"
: This command fetches all.txt
files from the specified directory. -
.BaseName
: This property is accessed for each file object returned byGet-ChildItem
, extracting just the filename without its extension.
Output:
You can also use the System.IO.FileInfo
class with the basename
property to get the file name without the extension.
System.IO.FileInfo
is a .NET
class that provides properties and instance methods for the creation, copying, deletion, moving, and opening of files. It also gives detailed information about a file, such as its name, path, size, etc.
Example:
([System.IO.FileInfo]"sample.txt").BaseName
This line assumes that sample.txt
is a file in the current directory. If the file is elsewhere, the full path should be specified, like C:\path\to\sample.txt
.
In this example, ([System.IO.FileInfo]"sample.txt")
creates a FileInfo
object for the file sample.txt
. This object contains detailed information about the file.
By accessing the .BaseName
property of this object, we retrieve the name of the file without its extension.
Output:
Use the System.IO.Path
Class to Remove Path and Extension From Filename in PowerShell
The System.IO.Path
class provides numerous methods for string manipulation of file paths. The GetFileNameWithoutExtension
method, in particular, is used to obtain the file name from a full path string without the extension.
Example:
[System.IO.Path]::GetFileNameWithoutExtension("sample.txt")
This example assumes that sample.txt
refers to a file name without any directory path. If the file is located in a different directory, you would include the full path, such as "C:\folder\sample.txt"
.
[System.IO.Path]::GetFileNameWithoutExtension("sample.txt")
, PowerShell utilizes the GetFileNameWithoutExtension
method from the System.IO.Path
class to process the string "sample.txt"
. This method intelligently parses the string, identifies the file extension, and removes it, returning only the base file name.
This method is highly efficient and does not require the actual file to exist, as it operates purely on the string level.
Output:
Conclusion
In conclusion, mastering file manipulation in PowerShell is integral for efficient system administration, and this article focused on removing the path and extension from filenames using diverse methods. Starting with the straightforward (Get-Item "sample.txt").BaseName
for individual files, we progressed to handling multiple files with (Get-ChildItem "C:\Users\rhntm\*.txt").BaseName
, a valuable approach for bulk operations.
The more object-oriented [System.IO.FileInfo]
class with ([System.IO.FileInfo]"sample.txt").BaseName
provided detailed file information, while the string manipulation prowess of [System.IO.Path]::GetFileNameWithoutExtension("sample.txt")
showcased the power of .NET
classes. These methods exemplify PowerShell’s versatility, and for those seeking to deepen their expertise, exploring related topics like working with full file paths and extracting file extensions is a logical progression, unlocking the full potential of PowerShell for comprehensive system scripting and automation tasks.