How to Get Full Path of the Files in PowerShell
-
Use
Get-ChildItem
to Get the Full Path of the Files in PowerShell -
Use
Select-Object
to Get the Full Path of the Files in PowerShell -
Use
Format-Table
to Get the Full Path of the Files in PowerShell -
Use the
foreach
Loop to Get the Full Path of the Files in PowerShell -
Use
Format-List
to Get the Full Path of the Files in PowerShell - Conclusion
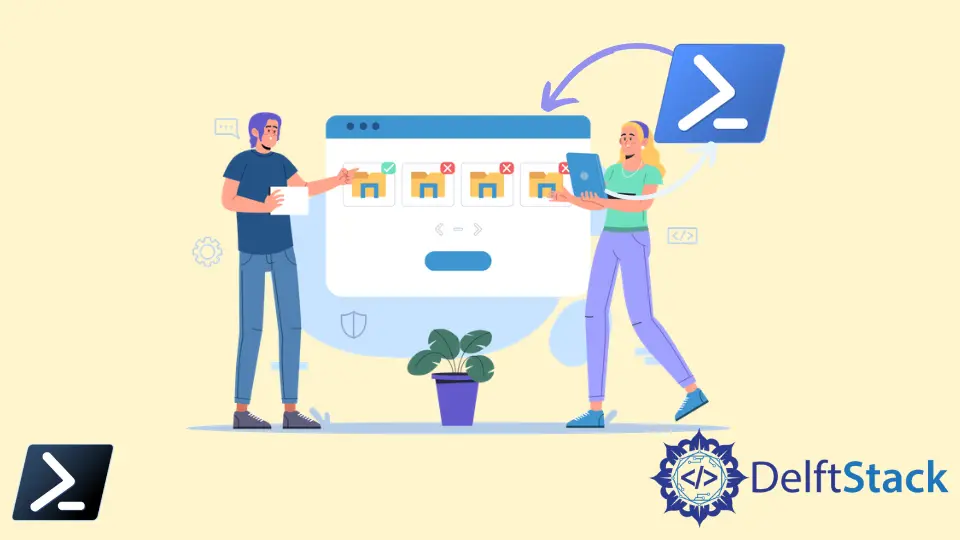
PowerShell has various cmdlets to manage the files on the system. You can create, copy, move, rename, and delete the files using PowerShell.
You can also search the files and check the existence of a file in PowerShell. A file path tells the location of the file on the system.
This tutorial will introduce different methods to get the full path of the files in PowerShell.
Use Get-ChildItem
to Get the Full Path of the Files in PowerShell
The Get-ChildItem
cmdlet displays a list of files and directories on the specified location. You can use the -Recurse
parameter to list all files and directories recursively.
It also shows the sub-directories and their files. It is helpful for recursive file search in PowerShell.
This approach is commonly used in scenarios where you need to perform operations on a set of files spread across a folder hierarchy, such as file searches, batch processing, or data organization tasks.
Example:
Get-ChildItem -Path C:\ExampleDirectory -Filter *.txt -Recurse
We start by designating our root directory with -Path C:\ExampleDirectory
. This choice establishes the starting point of our search, ensuring we’re looking in the right place.
Next, we introduce the -Filter *.txt
parameter. This is a strategic move on our part, as it significantly narrows down our search to only those files having .txt
extensions.
By doing so, we efficiently sidestep a myriad of unrelated files, making our script more focused and quicker in execution.
The real game-changer in our script is the -Recurse
parameter. This is where we tap into PowerShell’s ability to delve deep into directory structures.
With -Recurse
, we’re not just skimming the surface of C:\ExampleDirectory
; we’re exploring every nook and cranny, every subdirectory, no matter how deeply nested it is.
Output:
You can get the full path of the file by piping the command to %{$_.FullName}
.
Example:
Get-ChildItem -Path ExampleDirectory\ -Filter sample.txt -Recurse | % { $_.FullName }
Output:
Or, you can use this command to get all the files having the .txt
extension and their paths on the specified location.
Example:
Get-ChildItem -Path ExampleDirectory\ -Recurse | where { $_.extension -eq ".txt" } | % { $_.FullName }
Output:
Use Select-Object
to Get the Full Path of the Files in PowerShell
A similar method will be used, but we will use the Select-Object
cmdlet with the Get-ChildItem
command to get the full path of the files in PowerShell.
The Select-Object
cmdlet selects specified properties of an object or set of objects. This technique is particularly useful in scenarios where you need to audit file systems, manage data, or perform cleanup operations.
Example:
Get-ChildItem -Path ExampleDirectory\ -Filter *.txt -Recurse | Select-Object -ExpandProperty FullName
Through this script, we’re harnessing the combined power of Get-ChildItem
and Select-Object
to efficiently locate and list .txt
files. Starting our search from ExampleDirectory\
, we ensure that all relevant subdirectories are included.
The -Filter *.txt
is a crucial component, allowing us to zero in on only .txt
files, thus streamlining our search.
By employing -Recurse
, we’re not just scratching the surface; we’re delving deep into every subdirectory, ensuring no file is overlooked. The magic happens with | Select-Object -ExpandProperty FullName
, which refines our output to only show what’s essential – the full paths of the files.
Output:
Use Format-Table
to Get the Full Path of the Files in PowerShell
Similarly, you can use Format-Table
to get the full path of the files in PowerShell. The Format-Table
cmdlet formats the output as a table with the selected properties of an object.
The Get-ChildItem
cmdlet, when paired with Format-Table
, becomes a powerful tool for not only retrieving file paths but also presenting them in an organized, readable format. This method is particularly useful for those who need to quickly assess and report the structure of their file system.
Example:
Get-ChildItem -Path ExampleDirectory\ -Filter *.txt -Recurse | Format-Table FullName
In this script, we strategically utilize Get-ChildItem
to scour ExampleDirectory\
and its subdirectories for .txt
files. The -Filter *.txt
parameter is key, as it ensures that our search is focused and efficient, ignoring irrelevant file types.
The -Recurse
flag is crucial for a thorough search, ensuring no subdirectory is left unexplored.
Following the search, we employ | Format-Table FullName
to transform our findings into a well-organized table. This not only enhances readability but also provides a clear, structured view of the full paths.
Output:
Use the foreach
Loop to Get the Full Path of the Files in PowerShell
The foreach
loop is also known as the foreach
statement in PowerShell. It is a language construct for looping through a series of values in a collection of arrays, objects, strings, numbers, etc.
You can execute one or more commands against each item in an array within the foreach
loop.
Example:
Get-ChildItem -Path ExampleDirectory\*.txt -Recurse | foreach { "$_" }
In this script, we begin by deploying Get-ChildItem
to meticulously search ExampleDirectory\
for .txt
files.
The inclusion of -Recurse
ensures a comprehensive search through all layers of subdirectories. Each file encountered is then piped into a foreach
loop.
Within this loop, $_
acts as a placeholder for the current file. By enclosing $_
in quotes and outputting it, we instruct PowerShell to display the full path of each file.
This approach is not just about retrieving data; it’s a methodical walk through each file, offering the flexibility to add more complex operations within the loop.
Output:
The above command does not work with the -Filter
parameter. You can pipe to the Get-Item
cmdlet in the middle to use the -Filter
parameter.
Example:
Get-ChildItem -Path ExampleDirectory\ -Filter *.txt -Recurse | Get-Item | foreach { "$_" }
The addition of Get-Item
in the pipeline is a key step, as it allows the -Filter
parameter to be effectively utilized, ensuring that only .txt
files are processed.
Output:
Get-ChildItem
has built-in aliases ls
, dir
, and gci
. You can run all the above commands by using any of the handles in place of the Get-ChildItem
cmdlet.
Example:
gci -Path ExampleDirectory\ -Filter *.txt -Recurse | % { $_.FullName }
In using aliases, this command becomes much more concise while still maintaining its functionality. Users coming from different backgrounds (like Unix/Linux or older Windows command lines) might find ls
or dir
more familiar.
Output:
Use Format-List
to Get the Full Path of the Files in PowerShell
The Get-ChildItem
cmdlet is versatile for browsing and retrieving information about files and directories. When used with the -Filter
parameter, it can specifically target files of a certain type, like .txt
files.
Format-List
, on the other hand, displays the properties of objects in a list format. When combined with Get-ChildItem
, it can be used to present file paths in a clear, readable list.
Example:
Get-ChildItem -Path ExampleDirectory\ -Filter *.txt -Recurse | Format-List FullName
In our code, we use Get-ChildItem
to thoroughly search ExampleDirectory\
and its subdirectories (-Recurse
) for files with a .txt
extension (-Filter *.txt
). Each discovered file is then seamlessly transferred via a pipeline (|
) to the Format-List
cmdlet.
We focus on extracting the FullName
property of each file, representing its full path. This method is particularly effective for creating a well-organized and legible list of file paths.
Output:
Conclusion
To wrap up, PowerShell offers a plethora of cmdlets for proficient file management. In this article, we explored various methods to retrieve the full path of files using PowerShell, particularly leveraging the Get-ChildItem
cmdlet in combination with other cmdlets like Format-List
, Select-Object
, and Format-Table
.
These techniques are invaluable for tasks involving file system audits, data management, and batch processing. Each method we discussed provides a unique approach to handling file paths, whether it’s for filtering specific file types, listing paths in a formatted table, or iterating through files with a foreach
loop.
For readers looking to deepen their PowerShell expertise, the next steps could involve exploring more advanced file manipulation techniques, delving into PowerShell scripting for automation, or even integrating PowerShell commands with other tools and scripts for more complex workflows.