How to Get the File Extension Using PowerShell
-
Get the File Extension Using
Split-Path
in PowerShell -
Get the File Extension Using the
Get-ChildItem
Cmdlet in PowerShell -
Get the File Extension Using
System.IO.Path
in PowerShell -
Get the File Extension Using
System.IO.FileInfo
in PowerShell - Conclusion
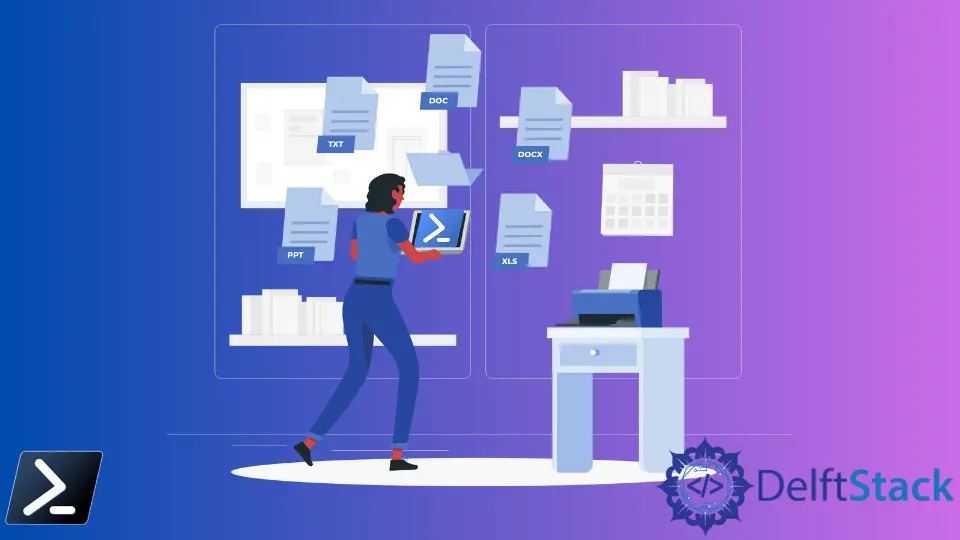
It is usually required to have a file name extracted from the full path while working on your PowerShell scripts. For example, your script received a full file path, and you want only to get the file extension.
This comprehensive guide delves into various methodologies for extracting file extensions using PowerShell, a task fundamental yet pivotal in numerous scripting scenarios. Each section of the article introduces a different approach, ranging from the simplicity of Split-Path
to the more sophisticated use of the .NET
framework and the System.IO.FileInfo
class.
Get the File Extension Using Split-Path
in PowerShell
The primary objective of using Split-Path -Leaf
in this context is to isolate the file name and its extension from a given path. This method is particularly effective when you’re working with just the file name or a relative path.
Once the file name is obtained, the extension can be easily extracted using string splitting methods.
Example Code:
# Define the file path
$filePath = "sample.txt"
# Extract the file extension
$extension = (Split-Path -Path $filePath -Leaf).Split(".")[1]
# Output the extension
Write-Output $extension
In this script, we start by defining a file name $filePath
. The Split-Path -Path $filePath -Leaf
command isolates the file name (sample.txt
).
We then split this string at the dot, resulting in an array where the first element is the file name without the extension (sample
), and the second element is the extension (txt
). By accessing the second element of this array with [1]
, we obtain the extension.
Finally, Write-Output
is used to display the extension.
Output:
Get the File Extension Using the Get-ChildItem
Cmdlet in PowerShell
The Get-ChildItem
command gets the items in one or more specified locations. For example, if the object is a container, it gets the things inside the said container, known as child items.
A location can be a file system, such as a directory, or a site exposed by a different Windows PowerShell provider. The Get-ChildItem
command gets the directories, subdirectories, and files in a file system drive.
Since the Get-ChildItem
cmdlet deals with files, it has a PowerShell property attribute that we can export to get the extension of the queried file. Unlike the Split-Path
cmdlet, this method can ship the extension properly even though there are dot characters in the filename.
Example Code:
Get-ChildItem 'sample.txt' | Select-Object Extension
In this script, Get-ChildItem 'sample.txt'
retrieves the file object for sample.txt
. The Get-ChildItem
cmdlet is typically used to list items in a directory, but when provided with a specific file name, it returns the file object for that file.
This object includes various properties, one of which is the file’s extension.
The output from Get-ChildItem
is then piped to Select-Object Extension
. This cmdlet filters the properties of the file object, outputting only the Extension
property.
Output:
Get the File Extension Using System.IO.Path
in PowerShell
The following approach is based on the .NET
framework classes. Though it is commonly discouraged to use .NET
framework classes on PowerShell scripts, especially if a native PowerShell command is available, it may be appropriate for this specific use case.
In the example below, if you are given the file name, we would use the GetExtension
static methods from the System.IO.Path
class:
Example Code:
[System.IO.Path]::GetExtension("sample.txt")
In the provided example, the .NET
class [System.IO.Path]
is used, and its static method GetExtension()
is called. We pass "sample.txt"
as the argument to the method.
This method analyzes the string and extracts the substring that represents the file’s extension. The beauty of this method lies in its simplicity and direct approach to handling file names and paths, as it doesn’t require the file to exist on the filesystem.
Output:
Get the File Extension Using System.IO.FileInfo
in PowerShell
The primary use of System.IO.FileInfo
in this context is to obtain detailed information about a specific file, such as its extension. This method is particularly useful in scripts where file metadata is crucial and there’s a need for more than just basic file handling.
Example Code:
# Create a FileInfo object for the specified file
$FileInfo = New-Object System.IO.FileInfo('sample.txt')
# Access the extension property of the FileInfo object
$Extension = $FileInfo.Extension
# Print the extension
Write-Output $Extension
In this script, we initiate the process by creating a System.IO.FileInfo
object for sample.txt
. This object encapsulates various details about the file, including its name, directory, size, and, importantly, its extension.
By accessing the .Extension
property of the FileInfo
object, we extract the extension of the file. This property returns the extension as a string, complete with the leading period (e.g., .txt
).
Output:
Conclusion
Navigating through the nuances of file extensions in PowerShell reveals a spectrum of techniques, each with its unique advantages and applications. This exploration has taken us from the straightforward usage of Split-Path
and Get-ChildItem
, ideal for simple and quick retrievals, to the deeper realms of the .NET
framework with System.IO.Path
and System.IO.FileInfo
, where a balance between complexity and capability is elegantly achieved.
Whether you are automating a routine task, performing bulk file operations, or developing sophisticated scripts for system management, understanding these methods enriches your PowerShell toolkit. The diverse approaches discussed here underscore PowerShell’s adaptability and robustness, empowering both novice and experienced scripters to tailor their solutions with precision and efficiency.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn