How to Convert a Secure String to Plain Text in PowerShell
-
Use the
Marshal
Class to Convert a Secure String to Plain Text in PowerShell -
Use the
ConvertFrom-SecureString
Cmdlet to Convert a Secure String to Plain Text in PowerShell -
Use the
NetworkCredential
Class to Convert a Secure String to Plain Text in PowerShell -
Use the
GetNetworkCredential
Method to Convert a Secure String to Plain Text in PowerShell - Conclusion
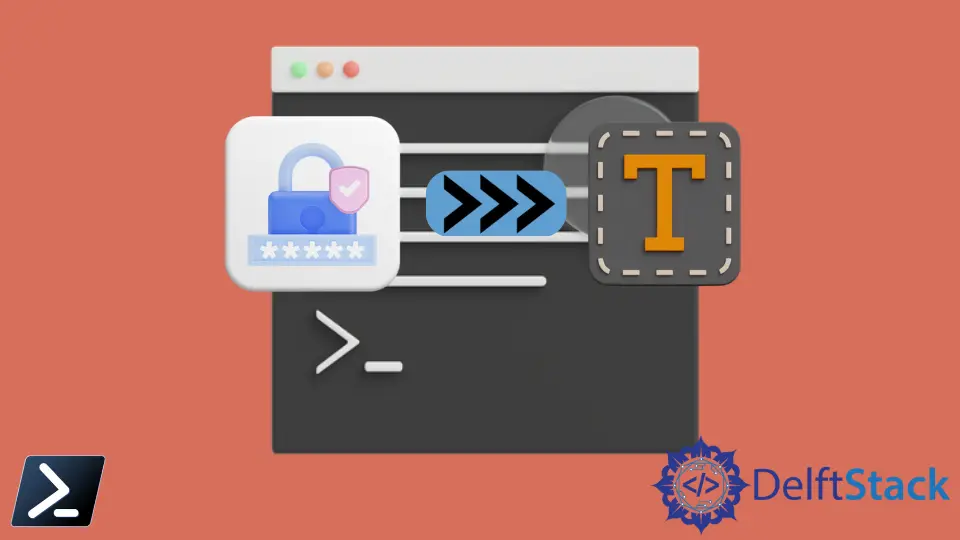
PowerShell offers various methods to handle SecureString
objects, which are designed to protect sensitive information in memory. However, there are scenarios where it becomes necessary to convert SecureString
to plain text despite security risks.
In this article, we explore different methods to achieve this conversion, including using the Marshal
class, the ConvertFrom-SecureString
cmdlet, the NetworkCredential
class, and the GetNetworkCredential()
method. Each method offers its advantages and considerations, providing flexibility in securely handling sensitive data within PowerShell scripts.
Use the Marshal
Class to Convert a Secure String to Plain Text in PowerShell
In PowerShell, the [System.Runtime.InteropServices.Marshal]
class provides methods to facilitate interoperation with unmanaged code and manipulate memory. Two of its methods, SecureStringToBSTR
and PtrToStringAuto()
, play a crucial role in converting SecureString
to plain text.
Example:
$secureString = ConvertTo-SecureString "MyPassword" -AsPlainText -Force
$secureStringPtr = [System.Runtime.InteropServices.Marshal]::SecureStringToBSTR($secureString)
$plainText = [System.Runtime.InteropServices.Marshal]::PtrToStringAuto($secureStringPtr)
$plainText
In this example, we first create a SecureString
object named $secureString
containing the password "MyPassword"
using the ConvertTo-SecureString
cmdlet. Then, we use [System.Runtime.InteropServices.Marshal]::SecureStringToBSTR($secureString)
to convert the SecureString
to a BSTR (Basic String) representation.
Finally, [System.Runtime.InteropServices.Marshal]::PtrToStringAuto($secureStringPtr)
is used to convert the BSTR representation to plain text and store it in the $plainText
variable.
Output:
Use the ConvertFrom-SecureString
Cmdlet to Convert a Secure String to Plain Text in PowerShell
The ConvertFrom-SecureString
cmdlet converts the secure string to an encrypted standard string. From PowerShell 7.0, a new parameter -AsPlainText
was added, which converts a secure string to a plain text string.
The following example requires PowerShell version 7.0 or later.
Example:
$secureString = ConvertTo-SecureString "MyPassword" -AsPlainText -Force
$plainText = ConvertFrom-SecureString -SecureString $secureString -AsPlainText
$plainText
In this example, we begin by creating a SecureString
object named $secureString
containing the password "MyPassword"
using the ConvertTo-SecureString
cmdlet. We then utilize the ConvertFrom-SecureString
cmdlet to convert $secureString
to its plain text representation, storing the result in the $plainText
variable.
Output:
Use the NetworkCredential
Class to Convert a Secure String to Plain Text in PowerShell
In PowerShell, the [System.Net.NetworkCredential]
class is a part of the .NET
Framework’s System.Net
namespace. It is primarily used for providing credentials for network operations, such as accessing web services, FTP servers, or network shares.
While its main purpose is authentication, it can also be utilized for converting a SecureString
to plaintext
in scenarios where plaintext
credentials are required.
Example:
$secureString = ConvertTo-SecureString "MyPassword" -AsPlainText -Force
$networkCredential = New-Object System.Net.NetworkCredential("", $secureString)
$plainText = $networkCredential.Password
$plainText
In this example, we create a SecureString
object named $secureString
containing the password "MyPassword"
using the ConvertTo-SecureString
cmdlet. Then, we instantiate a System.Net.NetworkCredential
object, $networkCredential
, providing an empty username (as it’s not required for our purpose) and the SecureString
containing the password as parameters.
Finally, we retrieve the plaintext
password from $networkCredential.Password
and store it in $plainText
.
Output:
Use the GetNetworkCredential
Method to Convert a Secure String to Plain Text in PowerShell
In PowerShell, the GetNetworkCredential()
method is part of the PSCredential
class, which is used to represent a set of user credentials. This method is primarily employed to extract the plaintext
representation of the password stored within a PSCredential
object.
Example:
$secureString = ConvertTo-SecureString "MyPassword" -AsPlainText -Force
$credential = New-Object System.Management.Automation.PSCredential("username", $secureString)
$plainText = $credential.GetNetworkCredential().Password
$plainText
In this example, we start by creating a SecureString
object named $secureString
containing the password "MyPassword"
using the ConvertTo-SecureString
cmdlet. Next, we create a PSCredential
object named $credential
with a dummy username and the $secureString
as the password.
Finally, we use the $credential.GetNetworkCredential().Password
method to extract the plaintext
password from the PSCredential
object.
Output:
Conclusion
In PowerShell scripting, converting SecureString
to plain text is a common requirement in various scenarios. By leveraging methods like the [System.Runtime.InteropServices.Marshal]
class, the ConvertFrom-SecureString
cmdlet, the [System.Net.NetworkCredential]
class, or the GetNetworkCredential()
method, users can securely handle sensitive information while also accommodating operational requirements.
Whether it’s interacting with external systems, legacy APIs, or network operations, these methods provide flexibility and convenience. However, it’s crucial to handle sensitive data with care and consider the security implications involved.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell