How to Replace a Text in File Using PowerShell
-
Use
Get-Content
andSet-Content
to Replace Every Occurrence of a String in a File With PowerShell -
Use
File
Class to Replace Every Occurrence of a String in a File With PowerShell - Replace Every Occurrence of a String in Multiple Files With PowerShell
- Conclusion
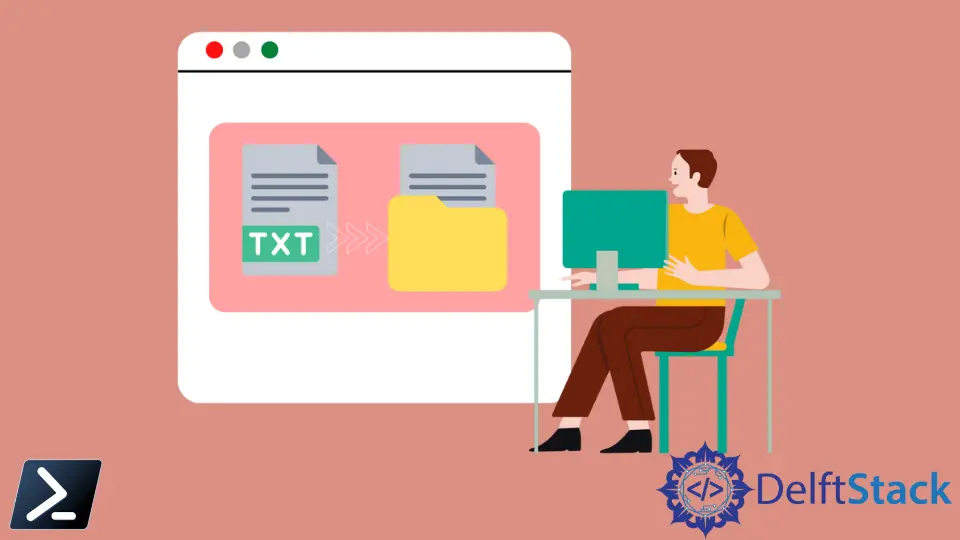
PowerShell is a powerful tool for managing files and folders. It allows you to create, copy, delete, move, rename, and view files and folders on the system.
This tutorial will introduce different methods to replace every string occurrence in a file with PowerShell.
Use Get-Content
and Set-Content
to Replace Every Occurrence of a String in a File With PowerShell
The 'Get-Content'
command retrieves the content of an item in the supplied path, such as the text in a file. The 'Set-Content'
cmdlet is a string-processing tool that allows you to add new content to a file or replace existing content.
You can use the Get-Content
and Set-Content
cmdlet to replace every string occurrence in a file.
Syntax:
(Get-Content -Path 'Path\to\Your\File.txt') -Replace 'OldString', 'NewString' | Set-Content -Path 'Path\to\Your\File.txt'
In the syntax, we replace 'OldString'
with the string we want to replace and 'NewString'
with the string we want to put in its place. Make sure to replace 'Path\to\Your\File.txt'
with the actual path to your file.
Let’s give an example. We have a text file (test.txt
) in the directory (C:\New
) with the following content.
Get-Content C:\New\test.txt
Output:
Welcome to Linux tutorials.
Linux is free.
Linux is powerful.
Now, let’s replace every occurrence of a string 'Linux'
with 'PowerShell'
in a test.txt
file with the help of the -Replace
parameter. The parentheses ()
are required around the Get-Content
.
(Get-Content C:\New\test.txt) -Replace 'Linux', 'PowerShell' | Set-Content C:\New\test.txt
Then, view the content of a test.txt
file to verify the changes.
Get-Content C:\New\test.txt
As you can see, Linux
has been successfully replaced with PowerShell
.
Output:
Welcome to PowerShell tutorials.
PowerShell is free.
PowerShell is powerful.
This method uses a string array to find and replace strings in a file because the Get-Content
cmdlet returns an array. It is easier to replace strings if the Get-Content
returns a single string.
We can use the -Raw
parameter as shown below. The -Raw
parameter is used to ensure that the content of 'C:\New\test.txt'
is read as a single string, allowing for efficient replacement of 'Linux'
with 'PowerShell'
throughout the entire file.
(Get-Content C:\New\test.txt -Raw) -Replace 'Linux', 'PowerShell' | Set-Content C:\New\test.txt
In this code, we read the content of a file located at 'C:\New\test.txt'
as a single string. Then, we use the -Replace
operator to find and replace all occurrences of 'Linux'
with 'PowerShell'
within that string.
Finally, we save the modified content back to the same file, effectively updating it with the new text. This code is a straightforward way to perform text replacements in a file using PowerShell.
Use File
Class to Replace Every Occurrence of a String in a File With PowerShell
The File
class provides static methods for common operations such as creating, copying, moving, opening, deleting, and appending to a single file. Use the File
class’ Replace()
method to replace the contents of a specified file.
Syntax:
$string = [System.IO.File]::ReadAllText("FilePath")
$string = $string.Replace("OldString", "NewString")
[System.IO.File]::WriteAllText("FilePath", $string)
Parameters:
"FilePath"
: This should be replaced with the actual file path."OldString"
: This is the string you want to replace."NewString"
: This is the string you want to replace it with.
The ReadAllText()
method opens a text file, reads all the text in that file, and then closes the file.
The WriteAllText()
method creates a new file, writes the specific string to the file, and closes the file. It is overwritten if the target file already exists in the location.
Let’s give an example. We have a text file (python.txt
) in the directory (C:\New
) with the following content.
Get-Content C:\New\python.txt
Output:
Find the best Python tutorials and learn Python easily from DelftStack.
Now, let’s replace every occurrence of a string in a file using the File
class method.
$string = [System.IO.File]::ReadAllText("C:\New\python.txt").Replace("Python", "JavaScript")
[System.IO.File]::WriteAllText("C:\New\python.txt", $string)
In the code above, we start by creating a variable called $string
, which will store the modified content of the 'C:\New\python.txt'
file after we perform text replacement. Using [System.IO.File]::ReadAllText("C:\New\python.txt")
, we read the entire file’s content and save it to $string
.
In the next step, we use the Replace()
method to locate all instances of "Python"
within the content and replace them with "JavaScript"
, effectively updating the text. Finally, we use [System.IO.File]::WriteAllText("C:\New\python.txt", $string)
to write the modified content back to the same file, overwriting the original text and preserving our changes.
Verify the changes made in C:\New\python.txt
.
Get-Content C:\New\python.txt
Output:
Find the best JavaScript tutorials and learn JavaScript easily from DelftStack.
In the output, we can see that "Python"
was replaced with the word "JavaScript"
.
Replace Every Occurrence of a String in Multiple Files With PowerShell
The above methods replace a string in a single file. Sometimes, you might need to replace the same string in multiple files.
In that case, you can use the command below to replace every occurrence of a specified string in multiple files.
Get-ChildItem 'C:\New\*.txt' | foreach {
(Get-Content $_) | foreach { $_ -Replace 'weekly', 'monthly' } | Set-Content $_
}
In the code above, we start by using Get-ChildItem
to collect a list of '.txt'
files in the 'C:\New'
directory. The asterisk *
wildcard specifies all files with the filename extension .txt
.
We then loop through each file, read its content with Get-Content
, and apply a replacement operation, changing 'weekly'
to 'monthly'
, using the -Replace
operator. The modified content is then written back to the same file with Set-Content
.
This process repeats for every file in the directory, efficiently replacing 'weekly'
with 'monthly'
in multiple files.
We can also use the -Recurse
parameter to replace a string in files in the specified directory and its subdirectories.
Get-ChildItem 'C:\New\*.txt' -Recurse | foreach {
(Get-Content $_) | foreach { $_ -Replace 'weekly', 'monthly' } | Set-Content $_
}
In this code, we begin by using Get-ChildItem
to assemble a list of '.txt'
files within 'C:\New'
and its subdirectories. The -Recurse
option ensures that we search for these files not only in the specified directory but also in its subdirectories.
We then use a pair of ForEach
loops to access each file’s content. Within the inner loop, we use the -Replace
operator to substitute 'weekly'
with 'monthly'
in the text.
Finally, we save the adjusted content back to the same file. This process runs through all the files in 'C:\New'
and its subfolders, allowing us to effectively replace 'weekly'
with 'monthly'
across numerous files and nested directories.
Conclusion
In this article, we’ve looked at different ways to replace strings in a single file using the Get-Content
and Set-Content
cmdlets, offering both a basic and more efficient -Raw
parameter method. Subsequently, we harnessed the power of the File
class to replace text within files, demonstrating a more advanced technique.
Furthermore, we extended our knowledge by replacing text across multiple files in a directory and its subdirectories. These methods make it easy for people to edit text in their files and show how useful PowerShell can be for tasks like this.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell