How to Find the Position of Substring in PowerShell
- String and Substring in PowerShell
-
Use the
IndexOf
Method to Find the Position of Substring in PowerShell -
Use the
LastIndexOf
Method to Find the Position of Substring in PowerShell -
Use the
Select-String
Cmdlet to Find the Position of Substring in PowerShell - Conclusion
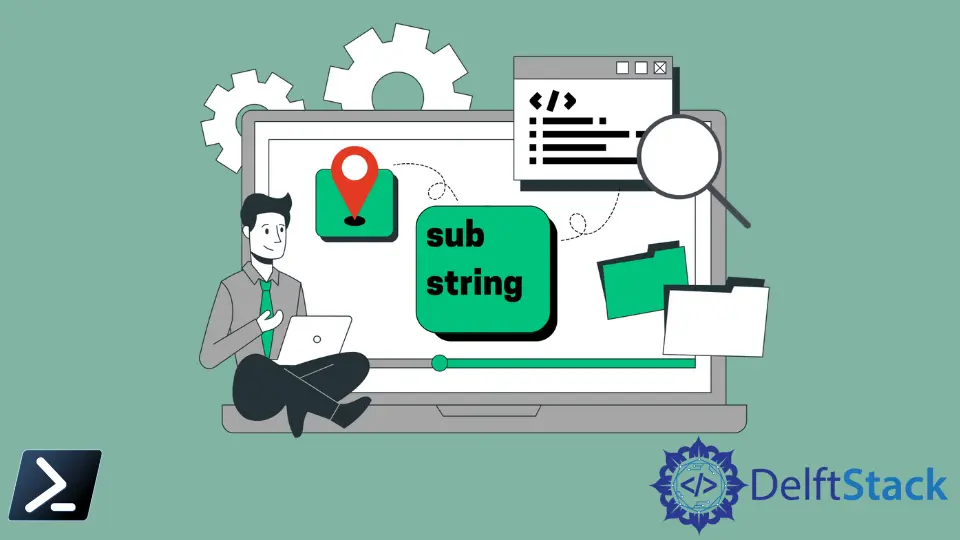
In PowerShell scripting, the ability to locate the position of a substring within a string is a fundamental requirement for various tasks, ranging from text processing to data manipulation. PowerShell offers multiple methods and cmdlets to efficiently find the position of substrings within strings.
This article explores and details diverse techniques to accomplish this task. Each method provides unique functionalities, catering to different scenarios and requirements.
From using simple string manipulation methods to harnessing the power of regular expressions, these approaches offer versatility and flexibility for substring searches in PowerShell scripts and commands.
By understanding and leveraging these methods, PowerShell users can effectively handle substring searches within strings, empowering them to manipulate, extract, or process data more efficiently.
This comprehensive guide aims to explore, explain, and demonstrate the implementation of various approaches to find the position of substrings within strings in PowerShell.
String and Substring in PowerShell
A string is a common data type used in PowerShell. It is the sequence of characters to represent texts.
You can define a string in PowerShell by using single or double quotes. For example, "ABC"
or 'ABC'
.
A substring is any specific text inside a string. If ABC
is a string, B
is its substring.
Sometimes, there might be a condition when you need to find a certain part of a string or the position of a substring in a text file. The IndexOf
method and LastIndexOf
mainly report the index position of a specified substring in PowerShell.
Use the IndexOf
Method to Find the Position of Substring in PowerShell
The IndexOf
method in PowerShell is used to determine the position (index) of the first occurrence of a specified substring within a given string. This method offers flexibility and ease of use, allowing users to perform precise substring searches within strings.
Syntax:
$position = $string.IndexOf($substring)
$string
: Represents the original string where the search is performed.$substring
: Specifies the substring to be located within$string
.$position
: Stores the position (index) of the first occurrence of$substring
within$string
.
In this method, you can specify the string, the starting search position in the string object, the number of characters to search, and the type of rules to use for the search.
The example below finds the position of the first occurrence of a substring to
in the given string.
"Welcome to PowerShell Tutorial".IndexOf("to")
Output:
8
The IndexOf
method counts the index from zero, which prints the output 8
.
In the following code, 9
is specified as the starting search position in the string.
"Welcome to PowerShell Tutorial".IndexOf("to", 9)
When you specify the starting search position, the character before that position is ignored, so it finds the position of the first occurrence of a substring to
after index 9
.
Output:
24
The IndexOf
method also accounts for scenarios where the substring is not present within the string, returning -1
in such cases.
For example, searching for a substring py
in the given string prints the result -1
.
"Welcome to PowerShell Tutorial".IndexOf("py")
Output:
-1
Advanced Usage: Case Sensitivity
The IndexOf
method in PowerShell is case-sensitive by default. However, it can be modified to perform a case-insensitive search using the StringComparison
parameter.
$string = "PowerShell is versatile and powerful"
$substring = "VERSATILE"
$position = $string.IndexOf($substring, [System.StringComparison]::InvariantCultureIgnoreCase)
Write-Host "Position of substring '$substring' (case-insensitive) is $position"
Output:
Position of substring 'VERSATILE' (case-insensitive) is 14
Explanation:
$string
: Holds the original string.$substring
: Contains the substring to be found (in a different case).$string.IndexOf($substring, [System.StringComparison]::InvariantCultureIgnoreCase)
: Utilizes theIndexOf
method with an additional parameter[System.StringComparison]::InvariantCultureIgnoreCase
to perform a case-insensitive search.- The resulting position is stored in
$position
and displayed usingWrite-Host
.
Use the LastIndexOf
Method to Find the Position of Substring in PowerShell
You can also use the LastIndexOf
method to find the index of a substring in PowerShell. The LastIndexOf
method finds the position of the last occurrence of a certain character inside a string.
The LastIndexOf
method in PowerShell serves as a potent means to identify the position (index) of the last occurrence of a specified substring within a given string. By enabling users to search from the end of the string, this method grants the flexibility to locate the final instance of a substring.
Syntax:
$position = $string.LastIndexOf($substring)
$string
: Represents the original string where the search is conducted.$substring
: Specifies the substring to be located within$string
.$position
: Stores the position (index) of the last occurrence of$substring
within$string
.
The following command finds the index of the last occurrence of a substring to
in the given string.
"Welcome to PowerShell Tutorial".LastIndexOf("to")
As shown, it prints the position of the last appearance of a substring to
.
Output:
24
Let’s see another example with a string variable $hello
.
$hello = "HelloHelloHello"
If you use the LastIndexOf
method to find the substring He
in $hello
, it returns 10
because it is the last index where He
occurs.
$hello.LastIndexOf("He")
Output:
10
The LastIndexOf
method returns -1
if the substring is not found within the string.
For example:
$hello.LastIndexOf("Hi")
Output:
-1
Advanced Usage: Case Sensitivity
Similar to the IndexOf
method, LastIndexOf
supports a case-insensitive search using the StringComparison
parameter.
$string = "PowerShell is versatile and powerful"
$substring = "POWERFUL"
$position = $string.LastIndexOf($substring, [System.StringComparison]::InvariantCultureIgnoreCase)
Write-Host "Position of the last occurrence of substring '$substring' (case-insensitive) is $position"
Output:
Position of the last occurrence of substring 'POWERFUL' (case-insensitive) is 28
Explanation:
$string
: Contains the original string.$substring
: Holds the substring to be found (in a different case).$string.LastIndexOf($substring, [System.StringComparison]::InvariantCultureIgnoreCase)
: Utilizes theLastIndexOf
method with an additional parameter[System.StringComparison]::InvariantCultureIgnoreCase
to perform a case-insensitive search.- The resulting position is stored in
$position
and displayed usingWrite-Host
.
Use the Select-String
Cmdlet to Find the Position of Substring in PowerShell
The Select-String
cmdlet in PowerShell is designed for performing string searches based on regular expressions (regex) or simple strings. It facilitates powerful pattern-matching capabilities, enabling users to search for specific substrings within strings and extract relevant information.
Syntax:
$match = $string | Select-String -Pattern $substring -AllMatches
$string
: Represents the original string where the search is conducted.$substring
: Specifies the substring to be located within$string
.$match
: Stores the result of the search operation, including the position (index) of the substring within the string.
The Select-String
cmdlet searches for texts in strings and files. It is similar to grep
in UNIX.
By default, it finds the first match in each line and displays all text containing the match.
"HelloHelloHello" | Select-String "He"
Output:
HelloHelloHello
The Matches
property of Select-String
finds the match between the specified string and the input string or file. The position of the matching substring is stored in the Index
property.
The following example shows how you can use the Matches.Index
property to find the position of a substring inside a string.
("HelloHelloHello" | Select-String "He").Matches.Index
It prints only the index 0
because Select-String
stops after the first match in each line of text.
Output:
0
You can use the -AllMatches
parameter to search for more than one match in each line.
("HelloHelloHello" | Select-String "He" -AllMatches).Matches.Index
As shown, it prints all the positions of the specified substring.
Output:
0
5
10
The following example finds all the positions of a specified substring in a text file test.txt
. The test.txt
contains the string "Use the Select-String Cmdlet to Find the Position of Substring in PowerShell"
.
(Get-Content test.txt | Select-String "the" -AllMatches).Matches.Index
Output:
4
37
This method is the best option to find all the characters’ positions in a string.
Select-String
returns no matches ($null
) if the substring is not found within the string.
$string = "PowerShell is versatile and powerful"
$substring = "Python"
$match = $string | Select-String -Pattern $substring -AllMatches
if (-not $match) {
Write-Host "Substring '$substring' not found in the string."
}
else {
$position = $match.Matches[0].Index
Write-Host "Position of substring '$substring' is $position"
}
Output:
Substring 'Python' not found in the string.
Explanation:
$string
: Contains the original string.$substring
: Represents the substring to be located.$string | Select-String -Pattern $substring -AllMatches
: Conducts a search for$substring
within$string
usingSelect-String
.- An
if
condition checks if the match result is$null
(-not $match
), displaying a message if the substring is not found.
Conclusion
The ability to locate the position of a substring within a string is vital for numerous text processing and data manipulation tasks in PowerShell. PowerShell offers several methods and cmdlets, such as IndexOf
, LastIndexOf
, and Select-String
, to efficiently accomplish this task.
The IndexOf
and LastIndexOf
methods provide straightforward means to find the position of substrings within strings. These methods offer functionalities to perform case-sensitive and case-insensitive searches, allowing users to pinpoint the exact positions of substrings within strings.
On the other hand, the Select-String
cmdlet harnesses the power of regular expressions for substring searches. This cmdlet offers extensive pattern-matching capabilities, including the ability to find all occurrences of a substring and extract their positions within strings.
Understanding and leveraging these methods and cmdlets empower PowerShell users to manipulate and process strings efficiently. By using these tools appropriately, users can handle various substring search scenarios, extract precise information, and streamline their PowerShell scripts and commands for effective data manipulation.
Related Article - PowerShell String
- Array of Strings in PowerShell
- How to Check if a File Contains a Specific String Using PowerShell
- How to Extract a PowerShell Substring From a String
- How to Extract Texts Using Regex in PowerShell
- How to Generate Random Strings Using PowerShell
- How to Escape Single Quotes and Double Quotes in PowerShell