How to Filter Files and Folders Using PowerShell
-
Filter Files Using the
Get-ChildItem
Cmdlet in PowerShell -
the
Get-ChildItem
Alias -
Get All Files Inside Subdirectories Using
Get-ChildItem
Cmdlet in PowerShell -
Filter Files With a Specific Conditions Using
Get-ChildItem
Cmdlet in PowerShell
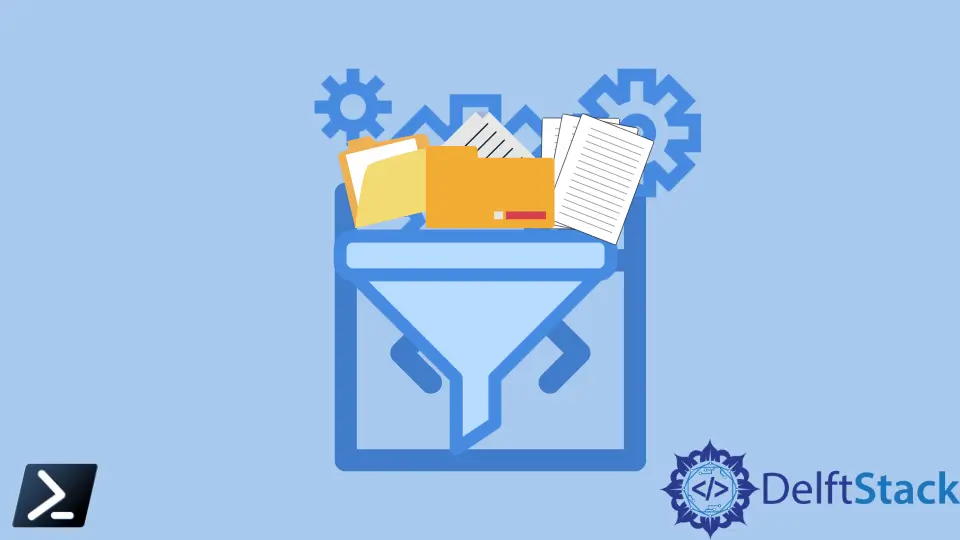
There will be situations wherein we need to check if files exist inside a specific location or directory. Though we can use the legacy command dir
, it wouldn’t be enough to only export specific files. We can use Windows PowerShell’s Get-ChildItem
cmdlet for this use case.
This article will discuss the PowerShell Get-ChildItem
cmdlet that we will use to get all items inside the directory and utilize its filter switch parameters.
The Windows PowerShell Get-ChildItem
cmdlet gets the child items in a specific location or directory. For example, the location specified in the cmdlet can be file system directory, registry, or certificate store. Also, the child items mentioned can either be another directory, subfolder, or file.
Get-ChildItem -Path C:\Temp
In the above command, Get-ChildItem
gets child items from the path specified using the -Path
parameter.
Get-ChildItem cmdlet when executed, display files, directories with their Mode
, LastWriteTime
, Length
(file size), and Name
properties on the PowerShell console.
Mode LastWriteTime Length Name
---- ------------ - ------ ----
d----l 18/01/2022 8:52 pm WindowsPowerShell
d----l 20/12/2021 3:36 pm Zoom
-a---l 30/12/2020 3:23 pm (151) backup phrase.txt
-a---l 17/06/2021 3:13 am (410049) CEF1750.pdf
-a---l 16/05/2020 3:32 am (677) default.cpu1
-a---l 21/08/2019 9:06 am (2240) Default.rdp
-a---l 26/05/2021 8:24 am (63399) e-sig.jpg
-a---l 09/03/2020 10:48 pm (143) fan config.sfsm
-a---l 19/09/2020 12:07 pm (279515089) MCSA.rar
Filter Files Using the Get-ChildItem
Cmdlet in PowerShell
We may use the -File
switch parameter to only return files inside a path or directory.
Example Code:
Get-ChildItem -Path C:\Temp -File
Output:
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---l 30/12/2020 3:23 pm (151) backup phrase.txt
-a---l 17/06/2021 3:13 am (410049) CEF1750.pdf
-a---l 16/05/2020 3:32 am (677) default.cpu1
-a---l 21/08/2019 9:06 am (2240) Default.rdp
-a---l 26/05/2021 8:24 am (63399) e-sig.jpg
-a---l 09/03/2020 10:48 pm (143) fan config.sfsm
-a---l 19/09/2020 12:07 pm (279515089) MCSA.rar
the Get-ChildItem
Alias
Windows PowerShell uses a default built-in alias gci
for the Get-ChildItem
cmdlet. Like the example snippet below, you may use the alias instead of the cmdlet for faster and seamless scripting.
Example Code:
gci -Path C:\Temp -File
Get All Files Inside Subdirectories Using Get-ChildItem
Cmdlet in PowerShell
If we want to get all files in directory and subdirectories, use the -Recurse
switch parameter.
Example Code:
gci -Path C:\Temp -Recurse - Force -File
-Force
parameter allows the cmdlet to get items that otherwise can’t be accessed by the user, such as system files or hidden files. The Force parameter doesn’t override security restrictions. Implementation varies among providers.Filter Files With a Specific Conditions Using Get-ChildItem
Cmdlet in PowerShell
Using the -Filter
parameter, we can filter out the results using a single expression. The -Filter
parameter doesn’t require the -Path
parameter as it will use your current working directory.
Example Code:
gci -Filter C:\Temp\* -Filter *.txt
We can also use the -Include
switch parameters, which accepts multiple conditions, an excellent advantage to the -Filter
parameter. However, remember that the -Include
parameter requires the -Path
parameter to be present in the expression.
Example Code:
gci -Path C:\Temp\* -File -Include CEF*.pdf, *.txt
Aside from accepting multiple conditions, the -Include
parameter works well with regex, thus making this a more versatile method than the -Filter
parameter.
Output:
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---l 30/12/2020 3:23 pm (151) backup phrase.txt
-a---l 17/06/2021 3:13 am (410049) CEF1750.pdf
*
) at the end when using the -Include
switch parameter. This wildcard denotes that you’re querying for all the path child items that have the specific extension defined in the -Include
parameter.Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedInRelated Article - PowerShell File
- How to Find a Specific File by Name Using PowerShell
- How to Loop Through Files and Folders in PowerShell
- PowerShell Copy File and Rename
- How to Split Large File in PowerShell
- How to Read CSV File Line by Line in PowerShell
- How to Unblock Files Using PowerShell