How to Query Active Directory Users in PowerShell
- Install the Active Directory Module
-
Use the
Get-ADUser
Cmdlet to Query Active Directory Users in PowerShell
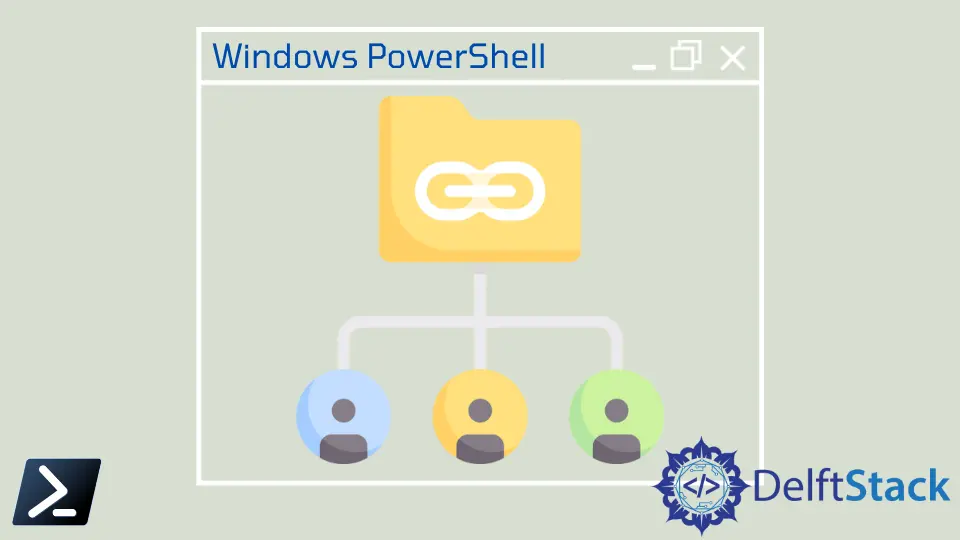
Windows PowerShell makes managing any Active Directory (AD) components effortless. We can handle any AD features, including managing active directory objects such as users, computers, and groups.
This article will discuss how to explore working one of the active directory objects and use Windows PowerShell Active Directory Module to manage active directory users.
Install the Active Directory Module
Usually, running the Windows PowerShell command, Install-Module
should bring the package from a remote CDN and install it on our machine.
Still, with the Active Directory Module, we must establish a pre-requisite package called the RSAT or the Remote Server Administration Tools for this to be successful.
To install RSAT on your computer or the server, we may run the PowerShell scripts below.
Install RSAT for Windows 10 onwards:
Add-WindowsCapability -Name Rsat.ActiveDirectory.DS-LDS.Tools~~~~0.0.1.0 -Online
Install RSAT for Windows Server (Multiple Builds from Windows Server 2008 onwards):
Install-WindowsFeature -Name "RSAT-AD-PowerShell" -IncludeAllSubFeature
Installing the RSAT feature on our computer will also install the Active Directory Module (AD Module) for PowerShell, enabling us to manage our Active Directory Domain Services.
Use the Get-ADUser
Cmdlet to Query Active Directory Users in PowerShell
Once the active directory module is installed, we can now import the active directory module with the following syntax. The Import-Module
command has an alias called ipmo
, and we can also use it as a great alternative when importing modules.
ipmo activedirectory
The Get-ADUser
command is a command that will provide as many options and information as possible to find domain users. We can use the Identity
parameter if we already know the NT ID or the username to search.
Below we can see some examples of finding a user account using various identifiers.
Example Code:
Get-ADUser -Identity jdoe101
Output:
DistinguishedName : CN=John Doe,OU=IT,DC=pureorg,DC=local
Enabled : False
GivenName : John
Name : John Doe
ObjectClass : user
ObjectGUID : b98fd0c4-3d5d-4239-8245-b04145d6a0db
SamAccountName : jdoe101
SID : S-1-5-21-4117810001-3432493942-696130396-3142
Surname : Doe
UserPrincipalName : jdoe101@pureorg.local
Filter AD User Accounts
If we need to find more than one domain user or don’t know an identifier, use a filter.
Each filter parameter allows us to perform conditional statements that narrow the search query. In this case, when this condition is met, the Get-ADUser
command will display user accounts matching that search condition.
The parameter to filter users is called the Filter
parameter. The Filter
parameter, from its literal name, allows us to create conditions that are very similar to the Windows PowerShell Where-Object
cmdlet filter syntax.
The example below is an excellent example of the Filter
parameter. This example will display an active directory attribute (givenName
) and define it as part of the conditional statement.
The filter only allows users to return if they have a givenName
equal to Albert
.
Example Code:
Get-ADUser -Filter "givenName -eq 'Albert'"
Output:
DistinguishedName : CN=AlBernard,OU=Accounting,DC=mylab,DC=local
Enabled : False
GivenName : Albert
Name : Bernard
ObjectClass : user
ObjectGUID : 8ec5ef2a8-1fdb-42ab-9706-b1e6356dd456
SamAccountName : AlBernard
SID : S-1-5-21-4117810001-3432493942-696130396-3163
Surname : Bernard
UserPrincipalName : AlBernard
Search Users in an Organizational Unit
PowerShell returns all domain users matching the criteria by providing an identity or filter. It does not limit to an Organizational Unit or OU.
We will need to set up a conditional statement for Get-ADUser
to filter by organizational unit using the SearchBase
parameter.
The SearchBase
parameter gives us the functionality to begin searching for a user account in a specific OU. The SearchBase
parameter takes an Organizational Unit’s distinguished name (DN).
For example, we could find all users in the Users
OU, as shown below. Using the Filter
of *
means to match all user accounts.
Example Code:
Get-ADUser -Filter * -SearchBase 'OU=Users,DC=domain,DC=local'
Perhaps we only want to find user accounts in a single OU and exclude child OUs. In that case, we could use the SearchBase
and SearchScope
parameters.
The SearchScope
parameter defines how deep we would like to search in the OU hierarchy.
For example, if we would like to find all user accounts in an organizational unit and all child organizational units, we would use 1
for the SearchScope
value. On the other hand, if we would want to search through all child and grandchildren OUs, we would use 2
.
The example below searches for user accounts inside the Users
Organizational Unit and all child OUs underneath it.
Example Code:
Get-ADUser -Filter * -SearchBase 'OU=Users,DC=domain,DC=local' -SearchScope 2
Get the Properties of a User
When we run Get-ADUser
, you’ll immediately see only a few attributes are returned. You’ll also notice that even when the output is piped to Select-Object -Property *
, all details aren’t still returned.
We can use the Property
parameter to get active directory user attributes from Windows PowerShell. This parameter accepts one or more comma-delimited features to show with the output.
The example snippet of code below uses the command Get-ADUser
to find all properties for all user accounts with a givenName
of Adam
. Unfortunately, the output is snipped, but you’ll see other familiar attributes like email address, password properties, etc.
Example Code:
Get-AdUser -Filter "givenName -eq 'Adam'" -Properties *
Output:
AccountExpirationDate :
accountExpires : 9223372036854775807
AccountLockoutTime :
AccountNotDelegated : False
AllowReversiblePasswordEncryption : False
AuthenticationPolicy : {}
AuthenticationPolicySilo : {}
BadLogonCount : 0
badPasswordTime : 0
badPwdCount : 0
CannotChangePassword : False
CanonicalName : mylab.local/Accounting/ADBertram
<SNIP>
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn