How to Create Directory Using Windows PowerShell
-
Using the
[System.IO]
Namespace to Create Directory in PowerShell - Using the File System Object to Create Directory in PowerShell
-
Using the
New-Item
Cmdlet to Create Directory in PowerShell -
Using the
md
Legacy Command to Create Directory in PowerShell
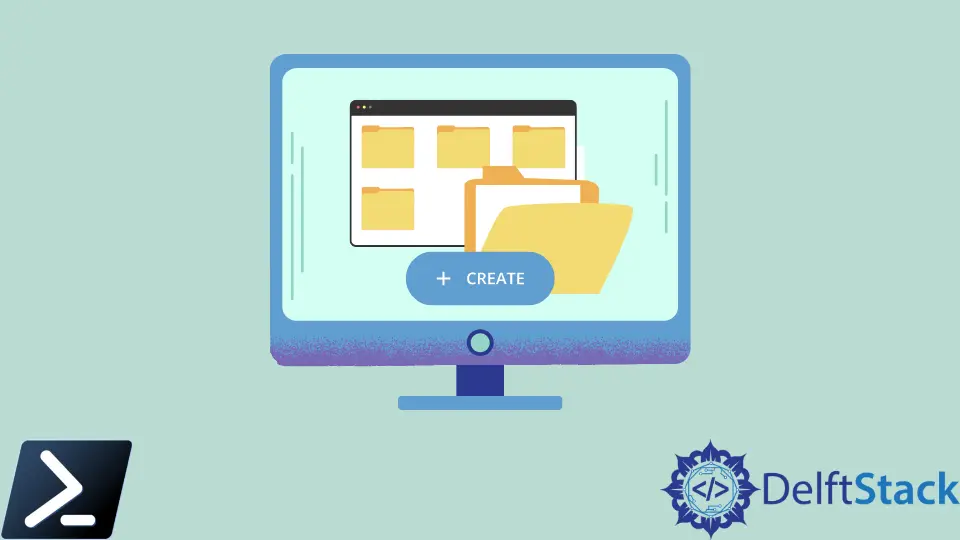
In Windows PowerShell, there are multiple ways to create file directories or folders that we can fully integrate into our scripts. This article will show how to create them using Windows PowerShell.
Using the [System.IO]
Namespace to Create Directory in PowerShell
Using the Directory .NET
Framework class from the system.io
namespace is possible. To use the Directory
class, use the CreateDirectory
static method and supply a path to the new folder’s location.
This technique is shown here.
[System.IO.Directory]::CreateDirectory("C:\test\newfolder")
When the command above runs, it returns a DirectoryInfo
class. However, we do not necessarily recommend this approach, but it is currently working and available.
Using the File System Object to Create Directory in PowerShell
Another way to create a directory is to use the Scripting.FileSystemObject
object from Windows PowerShell.
This method is the same object that VBScript and other scripting languages used to work with the file system. It is swift and relatively easy to use.
After the object is created, Scripting.FileSystemObject
exposes a CreateFolder
method. The CreateFolder
method accepts a string representing the path to create the folder.
After running, an object contains the path and other information about the newly-created directory.
$fso = New-Object -ComObject Scripting.FileSystemObject
$fso.CreateFolder('C:\test1')
Using the New-Item
Cmdlet to Create Directory in PowerShell
The native Windows PowerShell commands are also available to create directories. One way is to use the New-Item
cmdlet.
New-Item -Force -Path c:\test3 -ItemType Directory
When used, the -Force
switch parameter will overwrite existing folders that have been already created. Instead, we can use the Test-Path
cmdlet to check if the directory exists.
In the example below, we can create a script block to check if the directory exists. If the directory exists, it will not create the directory.
This syntax is one way of avoiding the -Force
switch parameter.
$path = "C:\temp\NewFolder"
if (!(Test-Path $path)) {
New-Item -ItemType Directory -Path $path
}
Suppose we compare this command’s output with the output from the previous .NET
command. The result is the same because the New-Item
cmdlet and the [System.IO.Directory]::CreateDirectory
command return a DirectoryInfo
object.
It is also possible to shorten the New-Item
command by leaving out the -Path
parameter name and only supplying the full path as a data type string with the ItemType
syntax. This revised command is shown here.
New-Item c:\test4 -ItemType directory
Some might complain that it was easier to create a directory in the old-fashioned command interpreter, cmd, because all they needed to type was md
–-and typing md
is undoubtedly easier than typing New-Item
command.
Using the md
Legacy Command to Create Directory in PowerShell
The previous example leads to the fourth way to create a directory (folder) using Windows PowerShell, using the md
function.
The advantage of using the md
command is that it is faster since it already knows you will create a directory. Therefore, you can leave the ItemType
parameter and type the full path directly.
md c:\test5
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn