How to Use the sleep() Function in PHP
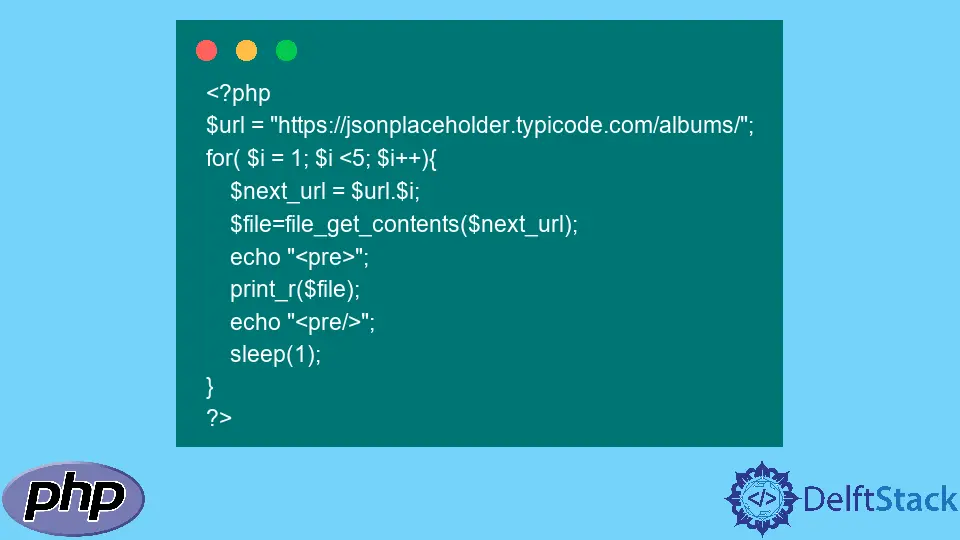
This article will introduce the practical implementation of the sleep function in PHP.
the sleep()
Function in PHP
PHP’s sleep()
function is used to delay execution by the specified time. It accepts a parameter that takes time as an integer, not negative values.
The function returns a 0 in case of success and returns a false or error in failure. Let’s see the working code from the example below.
<?php
echo date("h:i:s") . "<br>";
sleep(5);
echo date("h:i:s") . "<br>";
Output:
11:04:58
11:05:03
Here, the first timestamp printed is 11:04:58
. Then, the sleep()
function halts the program for five seconds.
Therefore, the second timestamp printed is 11:05:03
, which has the five seconds difference from the previous one.
Use the sleep()
Function in PHP
We can use the sleep()
function while requesting data from an API. When we continuously request large amounts of data, the server may get heavily loaded.
In such a case, we can use the sleep()
function to halt the consecutive request with a few seconds. We can utilize the file_get_contents()
function to get the request from an API.
The function takes a parameter $filename
, a file, or a URL. It reads the file and returns it as a string.
For example, create a variable $url
and store the URL https://jsonplaceholder.typicode.com/albums/
in it. Next, create a for
loop that iterates the $i
variable from 1
to 4
.
Next, concatenate the $url
with the iterator $i
so that the URL becomes https://jsonplaceholder.typicode.com/albums/1
in the first iteration. Assign the concatenation operation in the $next_url
variable.
The URL increments its suffix with 1 to get the successive API contents in the successive iterations. Next, create a $file
variable and assign it with the file_get_contents()
function with the $next_url
as the parameter.
Next, print the API response and use the sleep(1)
function to halt the data fetching for one second between successive requests.
In this way, we used the sleep()
function to stop the request to the API for one second between each request. Here, we have only looped four times for the demonstration.
Using the sleep()
function will be more practical when we get a large number of data.
<?php
$url = "https://jsonplaceholder.typicode.com/albums/";
for( $i = 1; $i <5; $i++){
$next_url = $url.$i;
$file=file_get_contents($next_url);
echo "<pre>";
print_r($file);
echo "<pre/>";
sleep(1);
}
?>
Output:
{
"userId": 1,
"id": 1,
"title": "quidem molestiae enim"
}
{
"userId": 1,
"id": 2,
"title": "sunt qui excepturi placeat culpa"
}
{
"userId": 1,
"id": 3,
"title": "omnis laborum odio"
}
{
"userId": 1,
"id": 4,
"title": "non esse culpa molestiae omnis sed optio"
}
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn