How to Create a PHP Function With Multiple Returns
- PHP Array to Return Multiple Values
- PHP Function With Conditional Dynamic Return
- Combination of PHP Array and Condition Dynamic Return
-
PHP
generator
toyield
Multiple Values
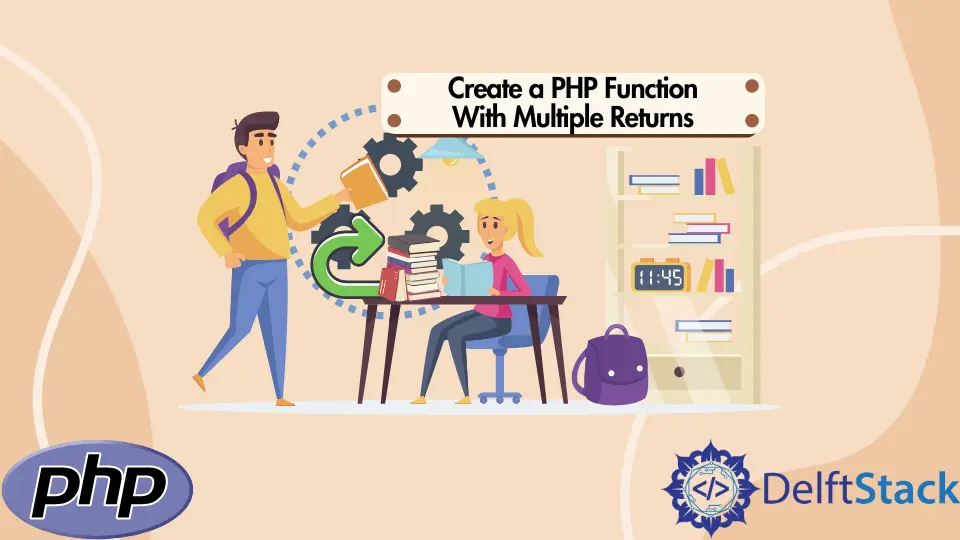
A PHP function is a re-usable block of statements, it allows us to have a return being a simple string, array or a JSON object. But it’s not possible to have more than one return. Although, you can use an array, create a conditional statement to return a dynamic variable or using generators (for PHP 5.5 and above).
PHP Array to Return Multiple Values
funtion arrayFunc(){
$var1 = "return 1";
$var2 = "return 2";
return array($var1, $var2);
}
In application:
$result = arrayFunc();
var_dump($result);
//output: array(2) { [0]=> string(8) "return 1" [1]=> string(8) "return 2" }
The function above can have multiple returns in the form of an array, it can be accessed using:
$result = arrayFunc();
echo $result[0]; // return 1
echo $result[1]; // return 2
Since PHP 7.1, there’s a new function called destructuring
for lists. It means, it’s possible to do things like:
$array = ['dog', 'cat', 'horse', 'fish'];
[$q, $w, $e, $r] = $array;
echo $q; // output: dog
echo $w; // output: cat
Applying in a function would look like this:
function descructingFunction(){
return ['A', 'sample', 'descructing', 'function'];
}
[$a, $b, $c, $d] = descructingFunction();
echo $a; //output: A
echo $d; // output: function
PHP Function With Conditional Dynamic Return
function condFunc($x = true){
$ret1 = "One";
$ret2 = "Two";
if($x == true){
return $ret1;
}else{
return $ret2;
}
}
In application:
echo condFunc(true);
//output: One
By taking this approach, it doesn’t need to return multiple values. Hence, the function will handle the condition to filter the correct value needed.
Combination of PHP Array and Condition Dynamic Return
Modifying the function a little can have the option of returning an array or a dynamic return.
Example:
function combination($x = true){
$ret1 = "One";
$ret2 = "Two";
if($x === true){
return $ret2;
}
if($x == "both"){
return array($ret1, $ret2);
}
}
echo combination(); //output: Two
var_dump(combination("both")) //output: array(2) { [0]=> string(8) "return 1" [1]=> string(8) "return 2" }
Using this approach will enable the function to be more flexible.
PHP generator
to yield
Multiple Values
A generator
function does not return a value, but yields as many values as needed. It returns an object that can be iterated.
Example:
function multipleValues(){
yield "return 1";
yield "return 2";
}
$return = multipleValues();
foreach($return as $ret){
echo $ret; //$ret first value is "return 1" then "return 2"
}
Note: The keyword of a generator
is yield
. It’s acting like a return
to a function statement, but it’s not stopping the execution and returning, it provides value to the code loops.
Caution: When using yield
as an expression, it must be surrounded by parentheses.
Example:
$generator = (yield $test);